Multiple Choice Questions
Question 1
A member method having the same name as that of the class is called ............... .
- an alias
- a friendly method
- a constructor
- a protected method
Answer
a constructor
Reason — A member method having the same name as that of the class is called constructor.
Question 2
A constructor has ............... return type.
- a void
- more than one
- String[] args
- no
Answer
no
Reason — A constructor has no return type.
Question 3
A constructor is used when an object is ............... .
- created
- destroyed
- assigned a value
- abstracted
Answer
created
Reason — A constructor is used when an object is created.
Question 4
A default constructor has ............... .
- no parameters
- one parameter
- two parameters
- multiple parameters
Answer
no parameters
Reason — A default constructor has no parameters.
Question 5
Pick the correct answer.
- A constructor has exactly the same name as its defining class.
- A constructor method does not have a return type.
- A constructor can be invoked only via the new operator.
- All of the above
Answer
All of the above
Reason — All the statements are correct.
Question 6
In constructor overloading, ............... .
- All constructors must have the same name as that of the class.
- All constructors must have the same number of arguments.
- All constructors must have arguments of type String[] args.
- All constructors must have no arguments.
Answer
All constructors must have the same name as that of the class.
Reason — In constructor overloading, all constructors must have the same name as that of the class.
Assignment Questions
Question 1
What is a constructor? Why do you need a constructor?
Answer
A constructor is a member method of a class that is used to initialise the instance variables. It has the same name as that of its defining class and does not have a return type.
A constructor is needed as it instructs the computer to perform the start-up tasks of a new object. These can be like assigning default values to instance variables, aligning all the buttons on a screen when a new window object is created, or moving the cursor to a specific data entry field for the user input.
Question 2
If the constructor is automatically generated by the compiler, why do you need to define your own constructor?
Answer
A default constructor does not have any statement inside it. It initializes default values to the instance variables.
If we define our own constructor in a class, we can initialise the instance variables according to our requirements. A parameterised constructor allows the programmer to initialise objects with different values. This is achieved by passing the required values as arguments to the constructor method.
Question 3
Explain the statement, "you cannot invoke constructors as normal method calls".
Answer
A constructor cannot be explicitly invoked like a method. It is automatically invoked via the new operator at the time of object creation. On the other hand, a method can be called directly by an object that has already been created. Normal method calls are specified by the programmer.
The below program highlights the difference in the way the constructor is invoked versus a normal method call:
class Test {
int a, b;
public Test(int x, int y) {
a = x;
b = y;
}
void add() {
System.out.println(a + b);
}
}
class invokeConst {
public static void main(String args[]) {
Test obj1 = new Test(10, 20); // constructor invoked
obj1.add(); // calling add() method
}
}
Question 4
Describe the importance of parameterised constructors.
Answer
Parameterized constructors are important because they provide a way to initialize objects with different values. For example, if we have a class representing a bank account, we could use a parameterized constructor to initialize a bank account with a specific balance, rather than always starting with a balance of zero. This makes the class more flexible and reusable, as it can be used to create objects with a variety of different initial states.
Question 5
If a class is named DemoClass, what names are allowed as constructor names in the class DemoClass?
Answer
The constructor can only have the name of the class in which it is defined. Thus, all the constructors of class DemoClass will be named DemoClass.
Question 6
Explain the concept of constructor overloading with an example.
Answer
The process of creating more than one constructor with the same name but with different parameter declarations is called constructor overloading.
The below example shows constructor overloading being used to create a class having three constructors:
public class Rectangle
{
int length;
int width;
//Constructor without any parameters
public Rectangle() {
System.out.println("Invoking constructor with no parameters");
length = 20;
width = 15;
}
// Constructor with one parameter
public Rectangle(int len) {
System.out.println("Invoking constructor with one parameter");
length = len;
width = 20;
}
// Constructor with two parameters
public Rectangle(int len, int wd) {
System.out.println("Invoking constructor with two parameters");
length = len;
width = wd;
}
public void Area() {
int area = length * width;
System.out.println("Length = " + length);
System.out.println("Width = " + width);
System.out.println("Area = " + area);
}
public static void main(String args[]) {
Rectangle rect1 = new Rectangle();
rect1.Area();
Rectangle rect2 = new Rectangle(30);
rect2.Area();
Rectangle rect3 = new Rectangle(30, 10);
rect3.Area();
}
}
Question 7
What is the use of the keyword this?
Answer
Within a constructor or a method, this
is a reference to the current object — the object whose constructor or method is being invoked. The keyword this
stores the address of the currently-calling object.
For example, the below program makes use of the this
keyword to resolve the conflict between method parameters and instance variables.
class Area
{
int length, breadth;
public Area(int length, int breadth) {
this.length = length;
this.breadth = breadth;
}
public void Display() {
int area = length * breadth;
System.out.println("Area is " + area);
}
public static void main(String args[J) {
Area area = new Area(2, 3);
area.Display();
}
}
Question 8
What is a no-argument constructor? Does every class have a no-argument constructor?
Answer
A no-argument constructor is a constructor that accepts no arguments and has no statements in its body. It is also called the default constructor.
If the programmer has not explicitly defined a constructor for the class then Java compiler automatically adds a no-argument constructor/default constructor. However, if the constructor is defined explicitly then no-argument constructor is not added.
Question 9
Create a class named Pizza that stores details about a pizza. It should contain the following:
Instance Variables:
String pizzaSize — to store the size of the pizza (small, medium, or large)
int cheese — the number of cheese toppings
int pepperoni — the number of pepperoni toppings
int mushroom — the number of mushroom toppings
Member Methods:
Constructor — to initialise all the instance variables
CalculateCost() — A public method that returns a double value, that is, the cost of the pizza.
Pizza cost is calculated as follows:
- Small: Rs.500 + Rs.25 per topping
- Medium: Rs.650 + Rs.25 per topping
- Large: Rs.800 + Rs.25 per topping
PizzaDescription() — A public method that returns a String containing the pizza size, quantity of each topping, and the pizza cost as calculated by CalculateCost().
public class Pizza
{
String pizzaSize;
int cheese;
int pepperoni;
int mushroom;
public Pizza(String size,
int tc,
int tp,
int tm) {
pizzaSize = size;
cheese = tc;
pepperoni = tp;
mushroom = tm;
}
public double CalculateCost() {
double totalCost = 0;
double topCost = 25 * (cheese + pepperoni + mushroom);
if (pizzaSize == "small")
totalCost = 500 + topCost;
else if(pizzaSize == "medium")
totalCost = 650 + topCost;
else
totalCost = 800 + topCost;
return totalCost;
}
public String PizzaDescription() {
double cost = CalculateCost();
String desc = "Size: " + pizzaSize
+ " Cheese: " + cheese
+ " Pepperoni: " + pepperoni
+ " Mushroom: " + mushroom
+ " Cost: Rs. " + cost;
return desc;
}
public static void main(String args[]) {
Pizza p = new Pizza("medium", 15, 5, 10);
String desc = p.PizzaDescription();
System.out.println(desc);
}
}
Output
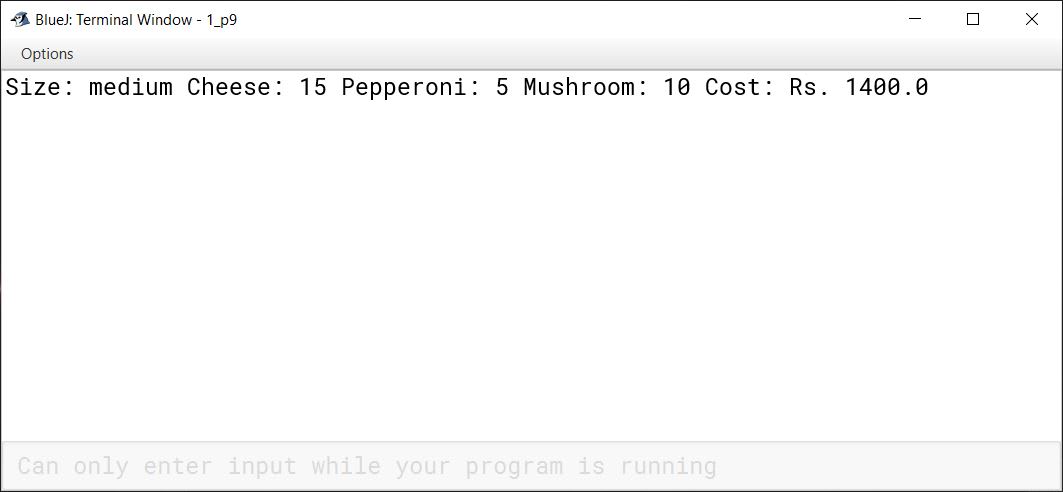