Practical Questions
Question 1
Design a program to accept a day number (between 1 and 366), year (in 4 digits) from the user to generate and display the corresponding date. Also, accept 'N' (1 <= N <= 100) from the user to compute and display the future date corresponding to 'N' days after the generated date. Display an error message if the value of the day number, year and N are not within the limit or not according to the condition specified.
Test your program with the following data and some random data:
Example 1
INPUT:
DAY NUMBER: 255
YEAR: 2018
DATE AFTER (N DAYS): 22
OUTPUT:
DATE: 12TH SEPTEMBER, 2018
DATE AFTER 22 DAYS: 4TH OCTOBER, 2018
Example 2
INPUT:
DAY NUMBER: 360
YEAR: 2018
DATE AFTER (N DAYS): 45
OUTPUT:
DATE: 26TH DECEMBER, 2018
DATE AFTER 45 DAYS: 9TH FEBRUARY, 2019
Example 3
INPUT:
DAY NUMBER: 500
YEAR: 2018
DATE AFTER (N DAYS): 33
OUTPUT:
DAY NUMBER OUT OF RANGE
Example 4
INPUT:
DAY NUMBER: 150
YEAR: 2018
DATE AFTER (N DAYS): 330
OUTPUT:
DATE AFTER (N DAYS) OUT OF RANGE
Solution
import java.util.Scanner;
public class DateCalculator
{
public static boolean isLeapYear(int y) {
boolean ret = false;
if (y % 400 == 0) {
ret = true;
}
else if (y % 100 == 0) {
ret = false;
}
else if (y % 4 == 0) {
ret = true;
}
else {
ret = false;
}
return ret;
}
public static String computeDate(int day, int year) {
int monthDays[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
String monthNames[] = {"JANUARY", "FEBRUARY", "MARCH",
"APRIL", "MAY", "JUNE",
"JULY", "AUGUST", "SEPTEMBER",
"OCTOBER", "NOVEMBER", "DECEMBER"};
boolean leap = isLeapYear(year);
if (leap) {
monthDays[1] = 29;
}
int i = 0;
int daySum = 0;
for (i = 0; i < monthDays.length; i++) {
daySum += monthDays[i];
if (daySum >= day) {
break;
}
}
int date = day + monthDays[i] - daySum;
StringBuffer sb = new StringBuffer();
sb.append(date);
sb.append("TH ");
sb.append(monthNames[i]);
sb.append(", ");
sb.append(year);
return sb.toString();
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("DAY NUMBER: ");
int dayNum = in.nextInt();
System.out.print("YEAR: ");
int year = in.nextInt();
System.out.print("DATE AFTER (N DAYS): ");
int n = in.nextInt();
if (dayNum < 1 || dayNum > 366) {
System.out.println("DAY NUMBER OUT OF RANGE");
return;
}
if (n < 1 || n > 100) {
System.out.println("DATE AFTER (N DAYS) OUT OF RANGE");
return;
}
String dateStr = computeDate(dayNum, year);
int nDays = dayNum + n;
int nYear = year;
boolean leap = isLeapYear(year);
if (leap && nDays > 366) {
nYear = nYear + 1;
nDays = nDays - 366;
}
else if (nDays > 365) {
nYear = nYear + 1;
nDays = nDays - 365;
}
String nDateStr = computeDate(nDays, nYear);
System.out.println();
System.out.println("DATE: " + dateStr);
System.out.println("DATE AFTER " + n
+ " DAYS: " + nDateStr);
}
}
Output
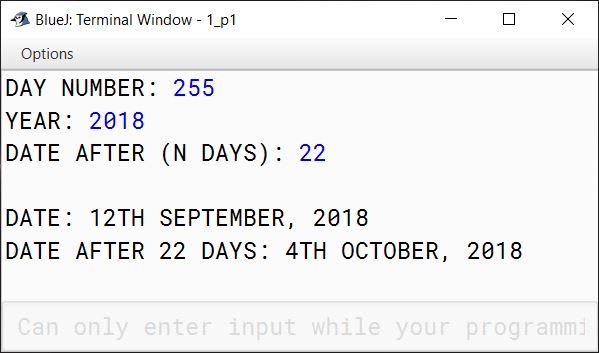
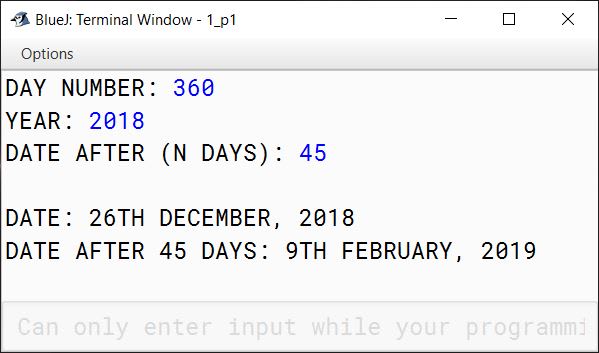
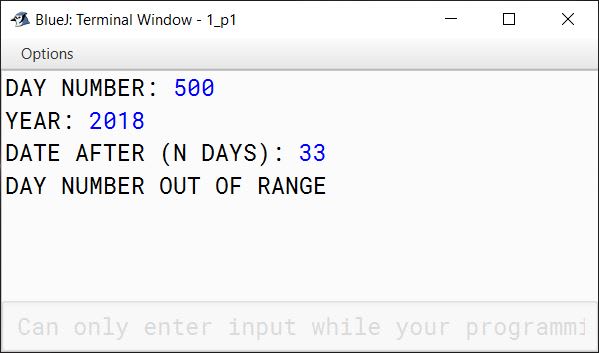
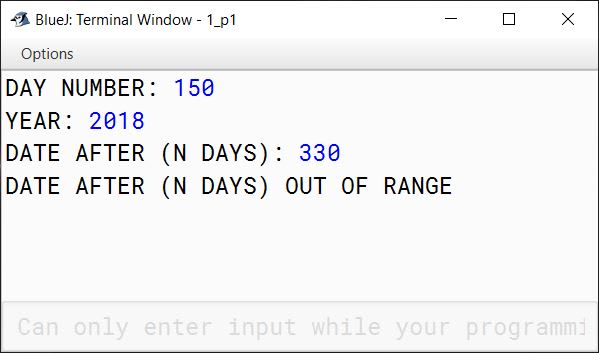
Question 2
Write a program to declare a single-dimensional array a[] and a square matrix b[][] of size N, where N > 2 and N < 10. Allow the user to input positive integers into the single dimensional array.
Perform the following tasks on the matrix:
- Sort the elements of the single-dimensional array in ascending order using any standard sorting technique and display the sorted elements.
- Fill the square matrix b[][] in the following format:
If the array a[] = {5, 2, 8, 1} then, after sorting a[] = {1, 2, 5, 8}
Then, the matrix b[][] would fill as below:
- Display the filled matrix in the above format.
Test your program for the following data and some random data:
Example 1
INPUT:
N = 3
ENTER ELEMENTS OF SINGLE DIMENSIONAL ARRAY: 3 1 7
OUTPUT:
SORTED ARRAY: 1 3 7
FILLED MATRIX
Example 2
INPUT:
N = 13
OUTPUT:
MATRIX SIZE OUT OF RANGE
Example 3
INPUT:
N = 5
ENTER ELEMENTS OF SINGLE DIMENSIONAL ARRAY: 10 2 5 23 6
OUTPUT:
SORTED ARRAY: 2 5 6 10 23
FILLED MATRIX
Solution
import java.util.Scanner;
public class Array
{
public static void sortArray(int arr[]) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int t = arr[j];
arr[j] = arr[j+1];
arr[j+1] = t;
}
}
}
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("ENTER VALUE OF N: ");
int n = in.nextInt();
if (n <= 2 || n >= 10) {
System.out.println("MATRIX SIZE OUT OF RANGE");
return;
}
int a[] = new int[n];
int b[][] = new int[n][n];
System.out.println("ENTER ELEMENTS OF SINGLE DIMENSIONAL ARRAY:");
for (int i = 0; i < n; i++) {
a[i] = in.nextInt();
}
sortArray(a);
System.out.println("SORTED ARRAY:");
for (int i = 0; i < n; i++) {
System.out.print(a[i] + " ");
}
for (int i = n - 1, r = 0; i >= 0; i--, r++) {
for (int j = 0; j <= i; j++) {
b[r][j] = a[j];
}
for (int k = n - 1; k > i; k--) {
b[r][k] = a[k - i - 1];
}
}
System.out.println();
System.out.println("FILLED MATRIX:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(b[i][j] + " ");
}
System.out.println();
}
}
}
Output
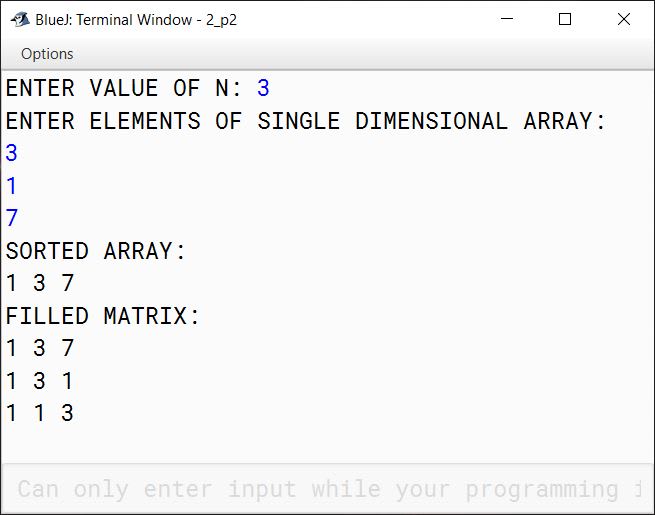
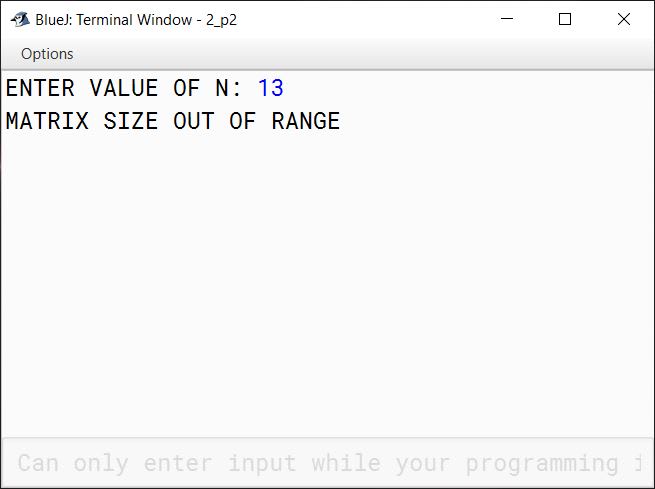
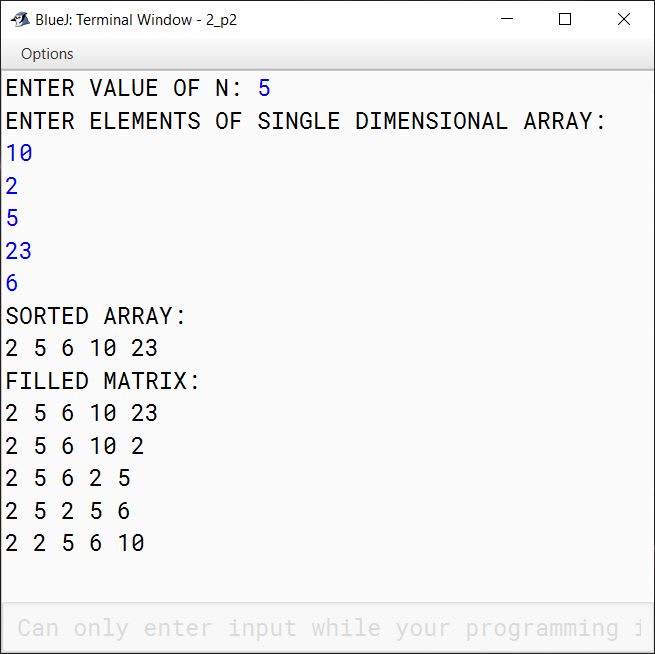
Question 3
Write a program to accept a sentence which may be terminated by either ‘.’, ‘?’ or ‘!’ only. The words are to be separated by a single blank space and are in uppercase.
Perform the following tasks:
(a) Check for the validity of the accepted sentence.
(b) Convert the non-palindrome words of the sentence into palindrome words by concatenating the word by its reverse (excluding the last character).
Example:
The reverse of the word HELP would be LEH (omitting the last alphabet) and by concatenating both, the new palindrome word is HELPLEH. Thus, the word HELP becomes HELPLEH.
Note: The words which end with repeated alphabets, for example ABB would become ABBA and not ABBBA and XAZZZ becomes XAZZZAX.
[Palindrome word: Spells same from either side. Example: DAD, MADAM etc.]
(c) Display the original sentence along with the converted sentence.
Test your program for the following data and some random data:
Example 1
INPUT:
THE BIRD IS FLYING.
OUTPUT:
THE BIRD IS FLYING.
THEHT BIRDRIB ISI FLYINGNIYLF
Example 2
INPUT:
IS THE WATER LEVEL RISING?
OUTPUT:
IS THE WATER LEVEL RISING?
ISI THEHT WATERETAW LEVEL RISINGNISIR
Example 3
INPUT:
THIS MOBILE APP LOOKS FINE.
OUTPUT:
THIS MOBILE APP LOOKS FINE.
THISIHT MOBILELIBOM APPA LOOKSKOOL FINENIF
Example 3
INPUT:
YOU MUST BE CRAZY#
OUTPUT:
INVALID INPUT
Solution
import java.util.*;
public class Palindrome
{
public static boolean isPalindrome(String word) {
boolean palin = true;
int len = word.length();
for (int i = 0; i <= len / 2; i++) {
if (word.charAt(i) != word.charAt(len - 1 - i)) {
palin = false;
break;
}
}
return palin;
}
public static String makePalindrome(String word) {
int len = word.length();
char lastChar = word.charAt(len - 1);
int i = len - 1;
while (word.charAt(i) == lastChar) {
i--;
}
StringBuffer sb = new StringBuffer(word);
for (int j = i; j >= 0; j--) {
sb.append(word.charAt(j));
}
return sb.toString();
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("ENTER THE SENTENCE:");
String ipStr = in.nextLine().trim().toUpperCase();
int len = ipStr.length();
char lastChar = ipStr.charAt(len - 1);
if (lastChar != '.'
&& lastChar != '?'
&& lastChar != '!') {
System.out.println("INVALID INPUT");
return;
}
String str = ipStr.substring(0, len - 1);
StringTokenizer st = new StringTokenizer(str);
StringBuffer sb = new StringBuffer();
while (st.hasMoreTokens()) {
String word = st.nextToken();
boolean isPalinWord = isPalindrome(word);
if (isPalinWord) {
sb.append(word);
}
else {
String palinWord = makePalindrome(word);
sb.append(palinWord);
}
sb.append(" ");
}
String convertedStr = sb.toString().trim();
System.out.println();
System.out.println(ipStr);
System.out.println(convertedStr);
}
}
Output
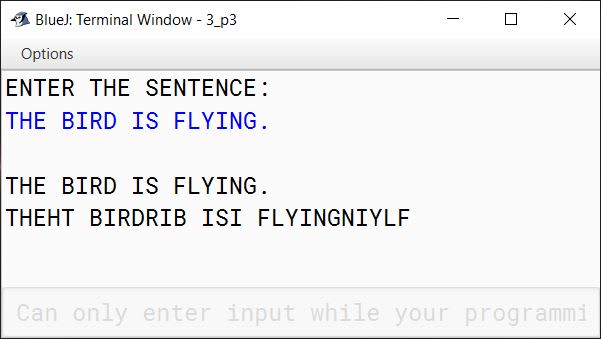
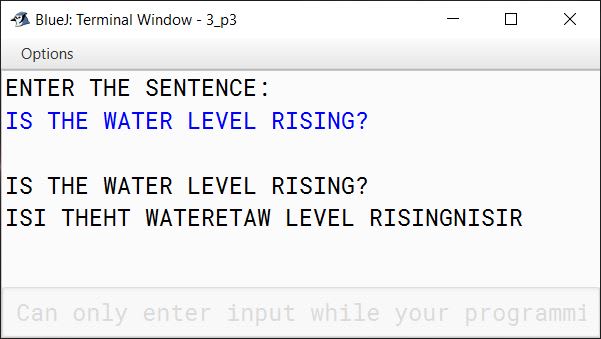
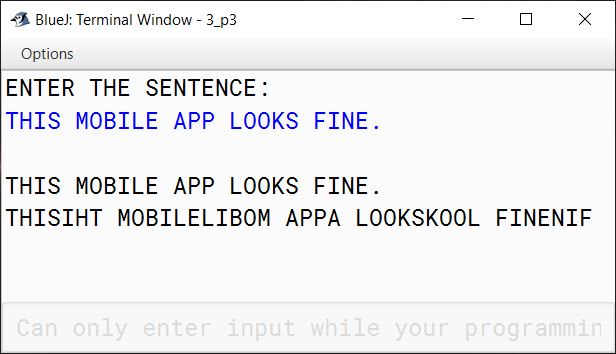
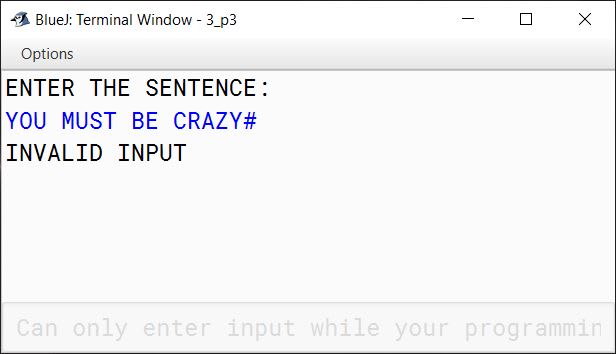