Java provides two selection statements — if and switch. We will first look at the if statement followed by the switch statement.
In our daily lives, most of the decisions we make depend upon some factors.
If it is cold, we wear a jacket. If it is raining and we must step outside, we wear a raincoat, or we take an umbrella. If our mobile phone’s battery is low, we put it on charging. If we are hungry, we eat food.
Java’s if statement helps us to do the same thing in our programs i.e. if statement helps to execute a set of statements based on certain conditions.
In its most simple form, if statement is written like this:
if (condition)
statement;
The if statement will test the condition. If condition is true, then statement is executed. If condition is false, then the statement is bypassed.
Recall from our “Code Blocks and Tokens” discussion that a code block containing multiple statements can replace the statement after if like this:
if (condition) {
statement1;
statement2;
statement3;
..
..
..
}
The condition in if statement should be an expression that evaluates to a boolean value. We can also use a boolean variable as condition of if statement. We will see an example of this soon.
if-else Statement
Many times, we want to do one set of things if some condition is true and a different set of things if that condition is false.
Building on our previous example, if it is raining, I will go to my office by car otherwise I will go by bike. If it is a holiday, you will not go to school otherwise you will go to school.
if statement provides an optional else clause to do such decision making in our programs. Its syntax is this:
if (condition)
statement a;
else
statement b;
If the condition
evaluates to true, statement a
is executed. If condition
is false, statement b
is executed. The if-else construct ensures that depending on the condition either statement a
or statement b
will be executed. Remember that, in no case will both statements be executed. Like if statement, here also we can replace statement a
and statement b
with code blocks containing multiple statements.
Enough theory, it is time to look at a few BlueJ programs to see if-else statement in action. Our first program takes 3 numbers as input from the user and prints the largest of the 3 numbers. This program demonstrates the simple use of if statement without any else clause.
import java.util.Scanner;
public class KboatGreatestNumber
{
public void findGreatestNumber() {
Scanner in = new Scanner(System.in);
System.out.println("Enter first number: ");
int a = in.nextInt();
System.out.println("Enter second number: ");
int b = in.nextInt();
System.out.println("Enter third number: ");
int c = in.nextInt();
int max;
/*
* Assume first number to
* be the greatest
*/
max = a;
/*
* If b is greater than
* greatest number then
* b is the greatest number
*/
if (b > max)
max = b;
/*
* If c is greater than
* greatest number then
* c is the greatest number
*/
if (c > max)
max = c;
/*
* Finally max will
* contain greatest
* of a, b, c
*/
System.out.println("Greatest number is " + max);
}
}
Let’s go through the program to understand it.
Scanner in = new Scanner(System.in);
System.out.println("Enter first number: ");
int a = in.nextInt();
System.out.println("Enter second number: ");
int b = in.nextInt();
System.out.println("Enter third number: ");
int c = in.nextInt();
In the above lines of the program, we accept 3 numbers from the user as input using the Scanner class and store them in int
variables a
, b
and c
, respectively.
Now we need to find the greatest of these 3 numbers. How to do that?
int max;
/*
* Assume first number to
* be the greatest
*/
max = a;
/*
* If b is greater than
* greatest number then
* b is the greatest number
*/
if (b > max)
max = b;
In the above lines of the program, I have declared the variable max
to hold the value of the greatest number. I will start by assuming that the first number is the greatest number. So, I am assigning the value of first number to max — max = a;
. After that, I will check if the second number is greater than my greatest number. If it is, then second number will become my greatest number. So, in the first if
statement I am checking if the value of b
is greater than the value of max
. If it is, then I am assigning the value of b
to max
as b
is the greatest number now. If b
is not greater than max
, then max
will keep the value of a
which we assigned to it previously. At this point we have the found the greater of the two numbers between a
and b
.
/*
* If c is greater than
* greatest number then
* c is the greatest number
*/
if (c > max)
max = c;
/*
* Finally max will
* contain greatest
* of a, b, c
*/
System.out.println("Greatest number is " + max);
Next, I will check if the third number is greater than my greatest number. To do that, in the if statement I am checking if c
is greater than max
. If it is, then I am assigning the value of c
to max
. If it is not, then max
keeps its value. After this second if check, max
has the value of the greatest of the 3 numbers which I am printing with the help of println method.
Below is the output of the program. It is correctly printing 150 as the greatest of 88, 150 & 37.
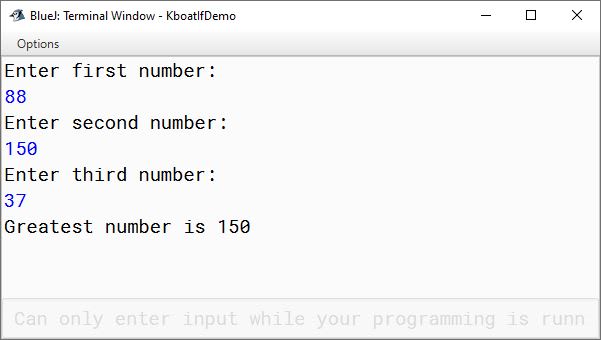
Next program shows the use of if-else statement:
import java.util.Scanner;
public class KboatEvenOdd
{
public void checkEvenOdd() {
Scanner in = new Scanner(System.in);
System.out.print("Enter number to check: ");
int number = in.nextInt();
/*
* If number is
* divisible by 2
* print even else
* print odd
*/
if (number % 2 == 0)
System.out.println(number + " is even.");
else
System.out.println(number + " is odd.");
}
}
This is a very simple program, which accepts a number from the user and prints whether the number is even or odd. First, we ask the user to input the number that we will be checking for even or odd. Next, the line if (number % 2 == 0)
checks if the number is divisible by 2 with the help of the modulus operator. If dividing the number by 2 doesn’t leave any remainder then the number is even otherwise it is odd.
Below is the output of the program, it is correctly identifying even and odd numbers.
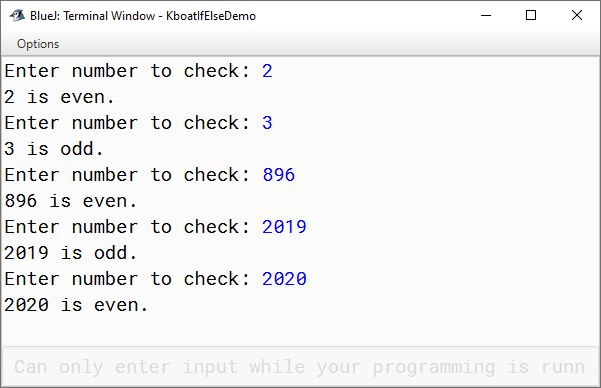
So far in our if-else programs, we have been using expressions which evaluate to a boolean value as the condition of our if statements. In the greatest number program, we used (b > max)
and (c > max)
as conditions of the if statement. In odd even program, we used (number % 2 == 0)
. As I mentioned before, we can also use a boolean variable as the condition of the if statement. This simple program will demonstrate it.
public class KboatRain
{
public void rainCheck() {
boolean isRaining = true;
if (isRaining)
System.out.println("I will go to office in car");
else
System.out.println("I will go to office on bike");
}
}
I have a Boolean variable isRaining
which I have initialized to true. In the if check I am directly using the variable isRaining
. If isRaining
is true, I am printing I will go to office in car
. If it is false, I am printing I will go to office on bike
. Let me execute it.
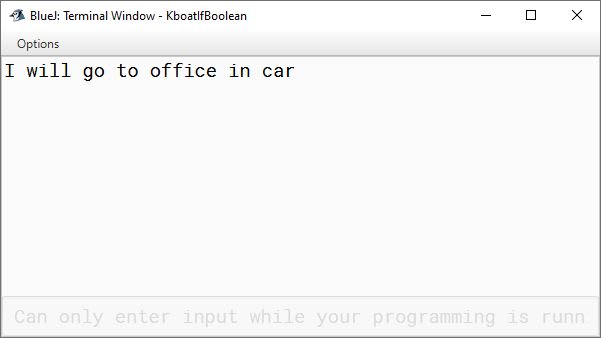
As, I have set isRaining
to true, "I will go to office in car" is getting printed on the screen. Let me change isRaining
to false and execute it.
Now I get "I will go to office on bike" as the output. So, we can use a Boolean variable directly as the condition of an if statement.
I can write the if statement like this as well — if (isRaining == true)
. Both ways are equivalent. Let me execute it and show you that I will get the same output as before. Here this usage of equality operator to check for true is redundant as isRaining
is a Boolean variable itself and can be directly used as the condition of if statement.
Missing Block Issue
import java.util.Scanner;
public class KboatGuessLetter
{
public void guessLetter() {
Scanner in = new Scanner(System.in);
System.out.print("Guess my favourite letter: ");
char favLetter = in.next().charAt(0);
if (favLetter == 'k' || favLetter == 'K')
System.out.println("Bingo! My favourite letter is " + favLetter);
else
System.out.println("My favourite letter is not " + favLetter);
System.out.println("Incorrect guess! Better luck next time.");
}
}
This is a very simple guess my favourite letter program. I have chosen 'k' as my favourite letter. I ask the user to input their guess for what my favourite letter is. I check if the user has entered 'k'. I check for both uppercase and lowercase 'k' as I want my program to be case-insensitive. Depending on whether or not the user has guessed correctly, I print an appropriate message for the user. Take a moment, go through the program and see if you can spot any error in it.
Were you able to figure out what’s wrong? Let’s see if you identified the error correctly. Let’s execute the program and make an incorrect guess first.
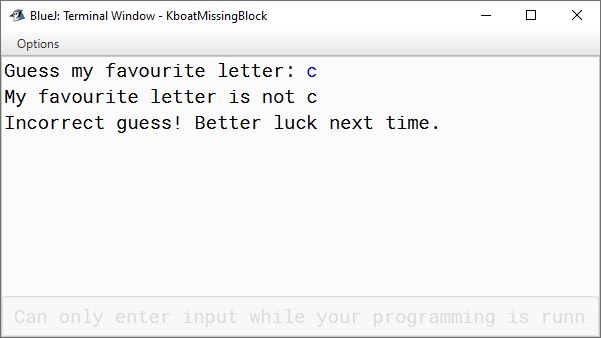
I have entered 'c' as my guess. Nothing wrong there, the output looks fine. Let me execute the program again and this time I will enter the correct guess 'k'.
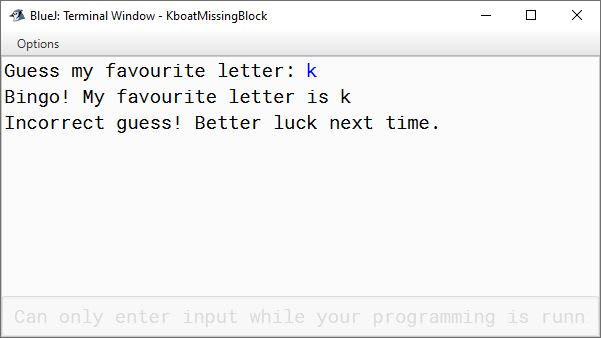
Now do you see the error? Along with the message for correct guess, the program is also printing the second line of incorrect message output.
So, what went wrong? Remember, only one statement can appear directly after the if or the else. If you want to include more than one statements, you must create a code block by enclosing the statements within a set of curly braces.
if (favLetter == 'k' || favLetter == 'K')
System.out.println("Bingo! My favourite letter is " + favLetter);
else
System.out.println("My favourite letter is not " + favLetter);
System.out.println("Incorrect guess! Better luck next time.");
Looking at the indentation level of this line, it is clear that I want to execute both statements inside the else clause of the if statement. But if you remember our discussion about whitespace in Java, compiler ignores all the whitespace. It only cares about the tokens in the program. So, this code compiles and executes but behaves incorrectly.
To make both these statements execute inside the else clause, I need to enclose them within a pair of curly braces like this.
if (favLetter == 'k' || favLetter == 'K')
System.out.println("Bingo! My favourite letter is " + favLetter);
else {
System.out.println("My favourite letter is not " + favLetter);
System.out.println("Incorrect guess! Better luck next time.");
}
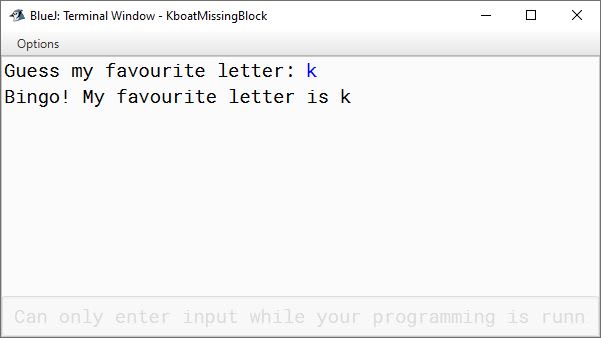
As you can see in the output above, our program is behaving correctly now.
Forgetting to define a code block when one is needed is a common cause of errors. So be watchful for your statements after the if and else clause and don’t let this error happen to you.