Character literals are written by enclosing the character you want to represent within a pair of single quotes. Some examples of character literals are 'd'
, '$'
, '7'
, 'P'
, 's'
, 'Z'
.
Escape Sequence
Most of the characters, you can directly enter inside the single quotes. But there are a few cases where entering a character directly is not possible. For example, what if you want to specify the single quote character itself as a character literal. Newline is also a very common example which you can’t enter directly inside the single quotes. For such scenarios, we use escape sequences.
An escape sequence is a sequence of characters that gets translated to another character when used inside single quotes. We can specify a character which is difficult or impossible to represent directly using its escape sequence.
The escape sequence starts with a backslash, followed by one or more characters that determine the interpretation of the escape sequence. The backslash along with the characters are enclosed within a pair of single quotes like regular character literals.
The escape sequence for newline is written like this — '\n'
. It starts with a backslash followed by letter n in lowercase and is enclosed in single quotes.
The escape sequence for single quote is this — '\''
. Backslash followed by a single quote character enclosed within a pair of single quotes.
Can you guess what will be the escape sequence of backslash itself? It is this — '\\'
. Two backslashes enclosed within single quotes.
When we were discussing char data type, we learnt that characters in Java are represented by their Unicode values. Using the concept of escape sequence, it is possible to write character literals as Octal or Hexadecimal representation of their Unicode values.
To write the character literal in Octal form, use the backslash followed by the three-digit number. Taking small a as an example, its Unicode value in octal is 141. It can be written as a character literal in octal form like this — '\141'
.
For Hexadecimal form use the backslash and u and then exactly four hexadecimal digits of the Unicode value. Small a in hexadecimal form will be written like this — '\u0061'
.
This table lists the character escape sequences in Java. You can use it as a handy reference.
Escape Sequence | Description |
---|---|
\ddd | Octal Unicode character (ddd) |
\uxxxx | Hexadecimal Unicode character (xxxx) |
\’ | Single quote |
\” | Double quote |
\ | Backslash |
\r | Carriage Return |
\n | New line |
\f | Form feed |
\t | Tab |
\b | Backspace |
Ok now that we have some understanding of character literals, let’s play around with them in a BlueJ program:
public class CharacterLiteralDemo
{
public void demoCharacterLiterals() {
//Regular Character Literals
char smallA = 'a';
char smallD = 'd';
char dollarSign = '$';
char capitalP = 'P';
System.out.println("Value of smallA is " + smallA);
System.out.println("Value of smallD is " + smallD);
System.out.println("Value of dollarSign is " + dollarSign);
System.out.println("Value of capitalP is " + capitalP);
System.out.println("");
//Escape Sequences
char singleQuote = '\'';
char newLine = '\n';
char backslash = '\\';
System.out.print("Value of singleQuote is " + singleQuote);
System.out.print(newLine);
System.out.print("Value of backslash is " + backslash);
System.out.print(newLine);
System.out.print(newLine);
//Octal & Hexadecimal form using Escape Sequences
char aInOctalForm = '\141';
char aInHexadeciamlForm = '\u0061';
System.out.println("Value of aInOctalForm is " + aInOctalForm);
System.out.println("Value of aInHexadeciamlForm is " + aInHexadeciamlForm);
}
}
Here is the output of this program:
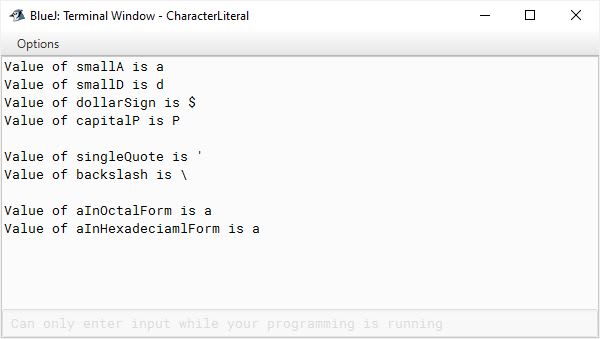
Now let’s go over the demoCharacterLiterals method. In the below lines of the program,
//Regular Character Literals
char smallA = 'a';
char smallD = 'd';
char dollarSign = '$';
char capitalP = 'P';
System.out.println("Value of smallA is " + smallA);
System.out.println("Value of smallD is " + smallD);
System.out.println("Value of dollarSign is " + dollarSign);
System.out.println("Value of capitalP is " + capitalP);
System.out.println("");
we define the character literals in the regular way by enclosing them within single quotes and then printing their values. In the output, all the char variables have their expected values.
Moving on to the next set of lines:
//Escape Sequences
char singleQuote = '\'';
char newLine = '\n';
char backslash = '\\';
System.out.print("Value of singleQuote is " + singleQuote);
System.out.print(newLine);
System.out.print("Value of backslash is " + backslash);
System.out.print(newLine);
System.out.print(newLine);
Here, we use escape sequences to represent single quote, new line and backslash as explained earlier in the lesson. Then we first print the value of singleQuote. It gets printed properly confirming that our escape sequence is correct and is working fine. Notice that we used print
and not println
in this set of print statements still the output appears as if it was printed using println
statements. The reason is the newLine
char variable. We are printing this after singleQuote
and backslash
. Newline character doesn’t have a graphical representation of itself, it makes the next output start on a new line. println
statements add the newline character after every output. Here we are simulating println by printing the newline character ourselves with the help of its escape sequence.
In the last set of lines:
//Octal & Hexadecimal form using Escape Sequences
char aInOctalForm = '\141';
char aInHexadeciamlForm = '\u0061';
System.out.println("Value of aInOctalForm is " + aInOctalForm);
System.out.println("Value of aInHexadeciamlForm is " + aInHexadeciamlForm);
we use the Octal and Hexadecimal forms of character literal a
to print it on the screen. Both println
statements output the lowercase letter a
as per the expectation.
String Literal
String literals are written by enclosing a sequence of characters in a pair of double quotes like this — "Hello Java"
.
We have already been using string literals a lot in our BlueJ programs as the input arguments of println
and print
methods. The escape sequences and octal and hexadecimal forms that we learnt in character literals works the same way in string literals too. Let’s quickly go through a few examples of string literals by trying them out in a BlueJ program:
public class StringLiteralDemo
{
public void demoStringLiteral() {
//Regular String literal
System.out.println("I love Java & BlueJ");
//String literal with escape sequence
System.out.println("Line1\nLine2\nLine3");
//String literal with hexadecimal
// '\u20b9' represents the rupee symbol in hexadecimal
System.out.println("\u20b984,599");
}
}
This is the output of the above program:
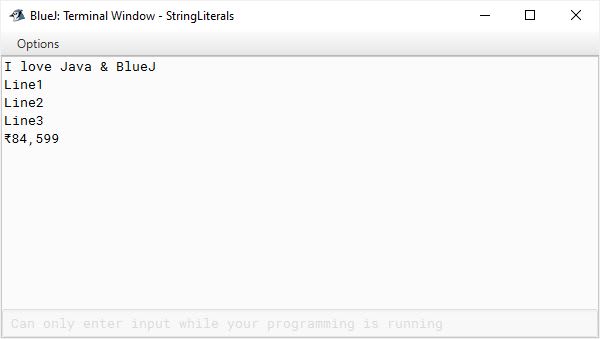
Let's go over the code of demoStringLiteral()
now. We first define a regular string literal — "I love Java & BlueJ"
and print it. We have been using this for a while now, nothing new here.
In the next string literal — "Line1\nLine2\nLine3"
, we have used the escape sequence for newline in between the words Line1,Line2 and Line2, Line3 so this literal gets printed on 3 lines as shown in the output above.
After that, in the next string literal — "\u20b984,599"
, we have used the Unicode value of rupee symbol in hexadecimal form which is this \u20b9
and printed a rupee amount of 84,599
on the screen.
One thing about Java string literals that is different from other programming languages is that strings in Java should begin and end on the same line. There is no line continuation escape sequence which we can use to continue our strings on multiple lines.
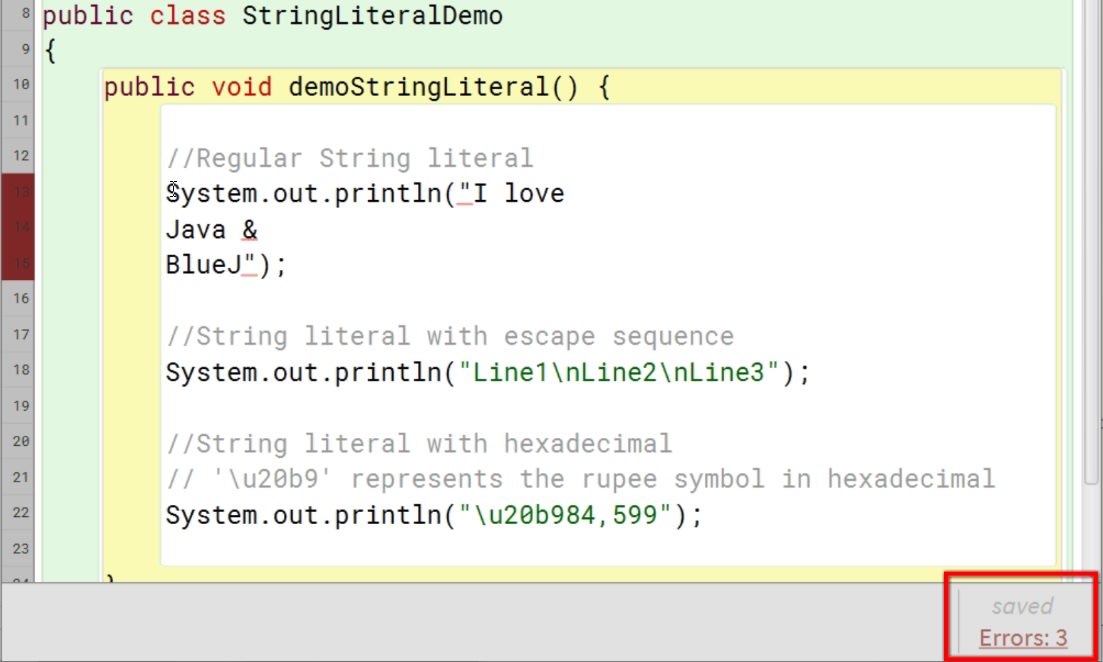
If I try writing a string literal on more than one line, it will result in a compilation error.