Section A
Question 1(i)
Consider the following code snippet
if (c > d)
x = c;
else
x = d;
Choose the correct option if the code mentioned above is rewritten using the ternary operator.
- x = (c > d) ? c : d;
- x = (c > d) ? d : c;
- x = (c > d) ? c : c;
- x = (c > d) ? d : d;
Answer
x = (c > d) ? c : d;
Reason — Correct syntax of ternary operator is:
variable = (test expression) ? exp 1 : exp 2
If the test expression is true, exp 1 is assigned to variable, else exp 2 is assigned to the variable.
Question 1(ii)
Which clause is optional in the switch statement?
- default
- case
- switch
- None of these
Answer
default
Reason — default clause is optional in the switch case.
Question 1(iii)
Errors occur in a program when :
- Syntax of the programming language is not followed
- the program does not run properly or does not execute at all
- The program produces an incorrect result
- All of the above
Answer
All of the above
Reason — Syntax errors occur when the syntax of the programming language is not followed. Runtime errors occur when the program does not run properly or does not execute at all and logical error occurs when the program produces an incorrect result.
Question 1(iv)
Object that share the same attributes and behaviour are grouped together into a/an
- interface
- instance
- alias
- class
Answer
class
Reason — Object that share the same attributes and behaviour are grouped together into a class.
Question 1(v)
The statement (1 > 0) || (1 < 0)
evaluates to
- 0
- 1
- false
- true
Answer
true
Reason — Logical OR operator returns true when even one of the conditions is true. Here condition (1 > 0)
is true. Thus, the OR operator results in true.
Question 1(vi)
The parameters that are passed to the method when it is invoked are called
- formal parameters
- actual parameters
- informal parameters
- void parameters
Answer
actual parameters
Reason — The parameters that are passed to the method when it is invoked are called actual parameters.
Question 1(vii)
Give the output of Math.sqrt(x); when x = 9.0.
- 3
- 3.0
- 3.00
- All of these
Answer
3.0
Reason — Math.sqrt() method returns the square root of an argument. Thus, 3.0 is returned by Math.sqrt(9.0).
Question 1(viii)
What will be the output of the following code?
String a[] = {"MI", "Samsung", "Micromax", "One Plus"};
System.out.println(a[3].length());
- 8
- 7
- 5
- 9
Answer
8
Reason — a[3]
refers to the fourth element of the array, which is "One Plus". The length()
method returns the number of characters in the string. The string "One Plus" has 8 characters, including spaces. Therefore, the output of the code is 8.
Question 1(ix)
Polymorphism is broadly used in implementing ...............
- inheritance
- encapsulation
- abstraction
- literals
Answer
inheritance
Reason — Polymorphism is broadly used in implementing inheritance.
Question 1(x)
Give the output of the following
switch (x)
{
case 'M' :
System.out.print ("Microsoft Teams");
break;
case 'G':
System.out.print ("Google Meet");
default:
System.out.print("Any Software");
break;
case 'W':
System.out.print("Web Ex");
break;
}
when x = 'g'
- Google Meet
- Any Software
- Google Meet
Any Software - Web Ex
Answer
Any Software
Reason — Java is a case sensitive language and thus 'G' and 'g' are treated as two different characters. Since the value of x does not match with any case, the default case will be executed. Thus, "Any Software" will be printed.
Question 1(xi)
Given array int Z[ ] = {15, 16, 17} ; it will occupy ............... bytes in memory.
- 3
- 12
- 24
- 64
Answer
12
Reason — int data type occupies 4 bytes in memory. The array Z[] has 3 elements. Thus, the array Z[] will occupy 4 bytes * 3 = 12 bytes in memory.
Question 1(xii)
In ............... search, the algorithm uses the middle value of the array for the search operation.
- binary
- linear
- bubble
- selection
Answer
binary
Reason — In binary search, the algorithm uses the middle value of the array for the search operation.
Question 1(xiii)
A single dimensional array contains N elements. What will be the last subscript?
- N
- N - 1
- N - 2
- N + 1
Answer
N - 1
Reason — The subscripts of an array range from 0 to size - 1. Thus, an array of size N will have the subscripts ranging from 0 to N - 1.
Question 1(xiv)
Identify the output of the following code :
String P = "20", Q = "19";
int a = Integer.parseInt(P);
int b = Integer.valueOf(Q);
System.out.println(a + "" + b);
- 2019
- 39
- 20
- 19
Answer
2019
Reason — In the given code, first strings P
and Q
are converted to their corresponding integer values. So a
becomes 20 and b
is 19. In the statement System.out.println(a + "" + b);
the expression a + ""
performs string concatenation as one of the operands of +
operator is a string (empty string ""
). As a result, the integer value a
is converted to a string. It is then concatenated with variable b
to give the output as 2019
.
Question 1(xv)
What will be the output of following code?
String c = "Hello i love java";
boolean var;
var = c.startsWith("hello");
System.out.println(var);
- true
- false
- 0
- 1
Answer
false
Reason — An important point to note in this code is that startsWith()
method in Java is case-sensitive, meaning it will differentiate between uppercase and lowercase characters. In this case, the prefix "hello" starts with a lowercase 'h', but the string in c
starts with an uppercase 'H'. Since the cases don't match, the startsWith()
method will return false
. So var
will be assigned false
and given as output.
Question 1(xvi)
A string function which removes the blank spaces provided in the prefix and suffix of a string.
- String.trim()
- String.ltrim()
- String.rtrim
- String.strim
Answer
String.trim()
Reason — String.trim() function removes the blank spaces provided in the prefix and suffix of a string.
Question 1(xvii)
Assertion (A) Identifier is a name given to a package, class, interface, method or variable.
Reason (R) Identifier allows a programmer to refer to the item from other places in the program.
- Both Assertion (A) and Reason (R) are true and Reason (R) is a correct explanation of Assertion (A).
- Both Assertion (A) and Reason (R) are true and Reason (R) is not a correct explanation of Assertion (A).
- Assertion (A) is true and Reason (R) is false.
- Assertion (A) is false and Reason (R) is true.
Answer
Both Assertion (A) and Reason (R) are true and Reason (R) is a correct explanation of Assertion (A).
Reason — Assertion (A) is true because an identifier is indeed a name given to a package, class, interface, method, or variable. Reason (R) is true because identifiers allow a programmer to refer to the item from other places in the program, hence it is the correct explanation of Assertion (A).
Question 1(xviii)
Read the following text and choose the correct answer:
The Java compiler breaks the line of code into text (words) is called Java tokens. These are the smallest element of the Java program. The Java compiler identified these words as tokens. These tokens are separated by the delimiters. Java tokens help the compiler. Which of the following is a type of Java token?
- Identifiers
- Keywords
- Operators
- All of these
Answer
All of these
Reason — Identifiers, keywords and operators are all tokens.
Question 1(xix)
Assertion (A) next() can read the input only till the space.
Reason (R) next() only gets to the line break.
- Both Assertion (A) and Reason (R) are true and Reason (R) is a correct explanation of Assertion (A).
- Both Assertion (A) and Reason (R) are true and Reason (R) is not a correct explanation of Assertion (A).
- Assertion (A) is true and Reason (R) is false.
- Assertion (A) is false and Reason (R) is true.
Answer
Assertion (A) is true and Reason (R) is false.
Reason — Assertion (A) is true because the next() method reads input until it encounters a space character by default. Reason (R) is false because next() stops reading at the first whitespace character (which includes spaces, tabs, and line breaks), not just at line breaks.
Question 1(xx)
It can have the same access specifiers used for variables and methods.
- Method
- Class
- Constructor
- Object
Answer
Constructor
Reason — A constructor can have the same access specifiers used for variables and methods.
Question 2(i)
Write the values of c and d after execution of following code.
int a = 1;
int b = 2;
int c;
int d;
c = ++b;
d = a++;
c++;
Answer
After the execution of the code, c = 4 and d = 1.
Explanation
Statement | Remarks |
---|---|
c = ++b; | Prefix operator first increments and then uses the value. Thus, c = 3, b = 3 |
d = a++; | Postfix operator first uses and then increments the value. Thus, d = 1, a = 2 |
c++; | c = c + 1 = 3 + 1 = 4 |
Question 2(ii)
Observe the following code and write how many times will the loop execute?
a = 5;
b = 2;
while(b != 0)
{
r = a % b;
a = b;
b = r;
}
System.out.println(" " + a);
Answer
The loop will execute 2 times.
Explanation
Iteration | r | a | b | Remark |
---|---|---|---|---|
5 | 2 | Initial values | ||
1 | 1 | 2 | 1 | r = 5 % 2 = 1 |
2 | 0 | 1 | 0 | r = 2 % 1 = 0 Loop terminates as b = 0 |
Question 2(iii)
Write the values that will be assigned to x, y and t after executing the following code.
String s1, s2, x, y;
int t;
s1 = "classxii";
s2 = "cbseboard";
x = s1.substring(5, 8);
y = s2.concat(s1);
t = y.length();
System.out.println("x=" + x);
System.out.println("y=" + y);
System.out.println("t=" + t);
Answer
Output
x=xii
y=cbseboardclassxii
t=17
Explanation
The substring() method returns a substring beginning from the startindex and extending to the character at index endIndex - 1. Here, startindex is 5 and end index is 7 (8 - 1). Since the string starts from index 0, the extracted substring is "xii". Thus x = xii
.
The concat() method adds one string (s1) at the end of another string (s2). Thus, "classxii" is added to the end of "cbseboard". Hence, y = "cbseboardclassxii
.
The length() method returns the total number of characters in a string. Thus, t = 17
.
Question 2(iv)
Write the values that will be stored in variables num and sum after execution of the following code.
int sum = 0, num = -2;
do
{
sum = sum + num;
num++;
} while (num < 1);
Answer
After the execution of the given code, num = 1
and sum = -3
Explanation
Iteration | sum | num | Remarks |
---|---|---|---|
0 | -2 | Initial values | |
1 | -2 | -1 | sum = 0 + (-2) = -2 |
2 | -3 | 0 | sum = -2 + (-1) = -3 |
3 | -3 | 1 | sum = -3 + 0 = -3 Loop terminates |
Question 2(v)
The following code has some error(s). Rewrite the correct code and underlining all the corrections made.
integer counter = 0;
i = 10; num;
for (num = i; num >= 1; num--);
{
If i % num = 0
{
counter = counter + 1;
}
}
Answer
The correct code is as follows:
int counter = 0; //Correction 1
int i = 10, num; //Correction 2
for (num = i; num >= 1; num--) //Correction 3
{
if( i % num == 0) //Correction 4
{
counter = counter + 1;
}
}
Explanation
Correction | Statement | Error |
---|---|---|
Correction 1 | integer counter = 0; | The datatype should be int |
Correction 2 | i = 10; num; | variable declaration cannot be done without a data type. The syntax for variable declaration is datatype variablename = value/expression; . Multiple variables should be separated by comma not semicolon. |
Correction 3 | for (num = i; num >= 1; num--); | If a semicolon is written at the end of the for loop, the block of the loop will not be executed. Thus, no semicolon should be written after for loop. |
Correction 4 | If i % num = 0 | If should be written in lower case and the condition should be written within brackets. Equality operator (==) should be used instead of assignment operator (=). |
Question 2(vi)
Rewrite the following program segment using while instead of for loop.
int f = 1, i;
for(i = 1; i <= 5 ; i++)
{
f *= i;
System.out.println(f);
}
Answer
int f = 1; i = 1;
while(i <= 5) {
f *= i;
System.out.println(f);
i++;
}
Question 2(vii)
State the method that
(a) converts a string to a primitive float data type.
(b) determines if the specified character is an uppercase character.
Answer
(a) Float.parseFloat()
(b) Character.isUpperCase()
Question 2(viii)
Write the Java statement for the following mathematical expression:
Answer
a = (0.02 - 3 * Math.pow(y, 3)) / (x + y);
Question 2(ix)
Give the output of the following expression, when a = 6.
a += ++a + a++ + a-- + a-- + --a + ++a
Answer
The output will be 46.
Explanation
a += ++a + a++ + a-- + a-- + --a + ++a [a = 6]
a = a + (++a + a++ + a-- + a-- + --a + ++a) [a = 6]
a = 6 + (7 + a++ + a-- + a-- + --a + ++a) [a = 7]
a = 6 + (7 + 7 + a-- + a-- + --a + ++a) [a = 8]
a = 6 + (7 + 7 + 8 + a-- + --a + ++a) [a = 7]
a = 6 + (7 + 7 + 8 + 7 + --a + ++a) [a = 6]
a = 6 + (7 + 7 + 8 + 7 + 5 + ++a) [a = 5]
a = 6 + (7 + 7 + 8 + 7 + 5 + 6) [a = 6]
a = 6 + 40
a = 46
Question 2(x)
Predict the output of the following.
(a) Math.pow(2.5, 2) + Math.ceil(5)
(b) Math.round(2.9) + Math.log(1)
Answer
(a) 11.25
(b) 3.0
Explanation
(a) Math.pow(x, y) method returns the value of x raised to the power of y. Math.ceil() method returns the smallest double value that is greater than or equal to the argument and is equal to a mathematical integer. Thus, the given expression is evaluated as follows:
Math.pow(2.5, 2) + Math.ceil(5)
⇒ 6.25 + 5.0
⇒ 11.25
(b) Math.round() method rounds off its argument to the nearest mathematical integer and returns its value as an int or long type. Math.log() method returns the natural logarithm of its argument. Thus, the given expression is evaluated as follows:
Math.round(2.9) + Math.log(1)
⇒ 3 + 0.0
⇒ 3.0
Section B
Question 3
Write a program to input a number. Check and display whether it is a Niven number or not. A number is Niven if it is divisible by the sum of its digits.
e.g. Input: 126
Output: 1 + 2 + 6 = 9 and 126 is divisible by 9.
import java.util.Scanner;
public class KboatNivenNumber
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int num = in.nextInt();
int orgNum = num;
int digitSum = 0;
while (num != 0) {
int digit = num % 10;
num /= 10;
digitSum += digit;
}
/*
* digitSum != 0 check prevents
* division by zero error for the
* case when users gives the number
* 0 as input
*/
if (digitSum != 0 && orgNum % digitSum == 0)
System.out.println(orgNum + " is a Niven number");
else
System.out.println(orgNum + " is not a Niven number");
}
}
Output
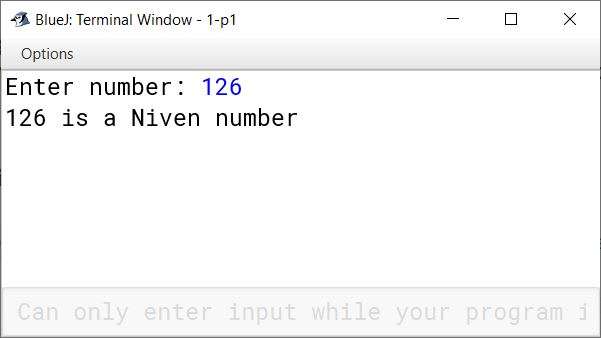
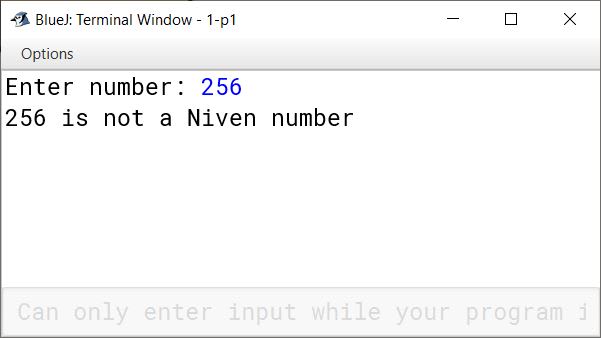
Question 4
Define a class to accept 10 different decimal numbers (double data type) in a Single Dimensional Array (say, A). Truncate the fractional part of each number of the array A and store their integer part in another array (say, B).
import java.util.Scanner;
public class KboatSDATruncate
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
double a[] = new double[10];
int b[] = new int[10];
System.out.println("Enter 10 decimal numbers");
for (int i = 0; i < a.length; i++) {
a[i] = in.nextDouble();
b[i] = (int)a[i];
}
System.out.println("Truncated numbers");
for (int i = 0; i < b.length; i++) {
System.out.print(b[i] + ", ");
}
}
}
Output
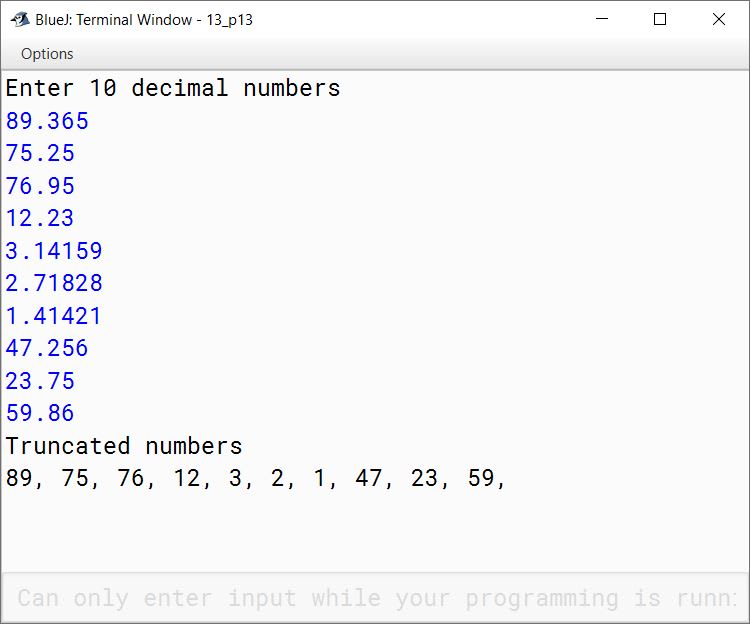
Question 5
Write a program to print the following patterns.
(i)
5
4 5
3 4 5
2 3 4 5
1 2 3 4 5
(ii)
J
I H
G F E
D C B A
public class KboatPattern
{
public static void main(String args[]) {
System.out.println("Pattern 1 ");
for (int k = 5; k >= 1; k--) {
for (int l = k; l <= 5; l++) {
System.out.print(l + " ");
}
System.out.println();
}
System.out.println();
System.out.println("Pattern 2 ");
char ch = 'J';
for (int i = 1; i <= 4; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(ch + " ");
ch--;
}
System.out.println();
}
}
}
Output
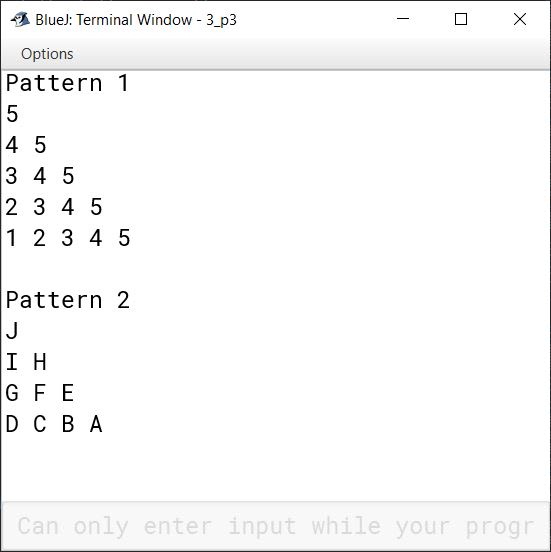
Question 6
Define a class StringMinMax to find the smallest and the largest word present in the string.
E.g.
Input:
Hello this is wow world
Output:
Smallest word: is
Largest word: Hello
import java.util.Scanner;
public class StringMinMax
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str += " ";
String word = "", lWord = "", sWord = str;
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ') {
if (word.length() > lWord.length())
lWord = word;
else if (word.length() < sWord.length())
sWord = word;
word = "";
}
else {
word += ch;
}
}
System.out.println("Smallest word: " + sWord);
System.out.println("Longest word: " + lWord);
}
}
Output
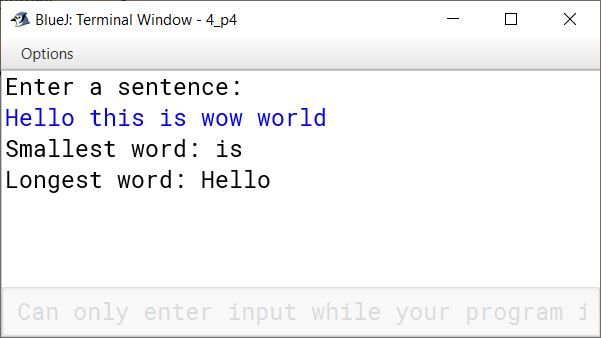
Question 7
A class Employee contains the following member:
Class name : Employee
Data members/Instance variables
String ename : To store the name of employee
int ecode : To store the employee code
double basicpay : To store the basic pay of employee
Member functions/Methods
Employee( - - - - ) : An argumented constructor to assign name, employee code and basic salary to data members/instance variables
double salCalc( ) : To compute and return the total salary of an employee
void display( ) : To display ename, ecode, basicpay and calculated salary
The salary is calculated according to the following rules :
Salary = Basic pay + HRA + DA + TA
where, HRA = 20% of basic pay
DA = 25% of basic pay
TA = 10% of basic pay
if the ecode <= 100, then a special allowance (20% of salary) will be added and the maximum amount for special allowance will be 2500.
if the ecode > 100 then the special allowance will be 1000.
Hence, the total salary for the employee will calculated as :
Total Salary = Salary + Special Allowance
Specify the class Employee giving the details of the constructor, double salCalc() and void display(). Define the main() function to create an object and call the functions accordingly to enable the task.
import java.util.Scanner;
public class Employee
{
private String ename;
private int ecode;
private double basicpay;
public Employee(String name,
int code,
double sal) {
ename = name;
ecode = code;
basicpay = sal;
}
public double salCalc() {
double hra = 20.0/100.0 * basicpay;
double da = 25.0/100.0 * basicpay;
double ta = 10.0/100.0 * basicpay;
double allowance;
double sal = basicpay + hra + da + ta;
if (ecode <= 100) {
allowance = 20.0/100.0 * sal;
if(allowance > 2500)
allowance = 2500;
}
else {
allowance = 1000;
}
double tsal = sal + allowance;
return tsal;
}
public void display() {
double totalsal = salCalc();
System.out.println("Name : " + ename);
System.out.println("Employee Code : " + ecode);
System.out.println("Basic Pay : Rs. " + basicpay);
System.out.println("Salary : " + totalsal);
}
public static void main() {
Scanner in = new Scanner(System.in);
double totalsal;
System.out.print("Enter Name : ");
String name = in.nextLine();
System.out.print("Enter Employee Code : ");
int empcode = in.nextInt();
System.out.print("Enter Basic Pay : ");
double basicsal = in.nextDouble();
Employee obj = new Employee(name,
empcode,
basicsal);
obj.display();
}
}
Output
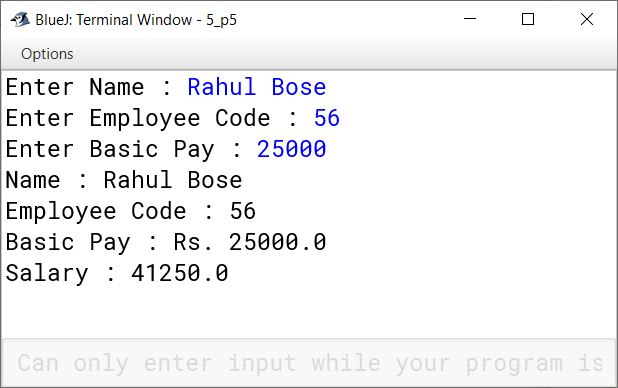
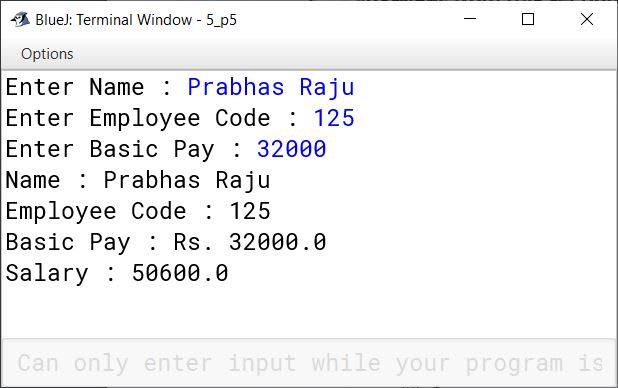
Question 8
Write a program to accept the year of graduation from school as an integer value from the user. Using the binary search technique on the sorted array of integers given below, output the message "Record exists" if the value input is located in the array. If not, output the message "Record does not exist".
{ 1982, 1987, 1993, 1996, 1999, 2003, 2006, 2007, 2009, 2010 }
import java.util.Scanner;
public class KboatGraduationYear
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int n[] = {1982, 1987, 1993, 1996, 1999, 2003,
2006, 2007, 2009, 2010};
System.out.print("Enter graduation year to search: ");
int year = in.nextInt();
int l = 0, h = n.length - 1, idx = -1;
while (l <= h) {
int m = (l + h) / 2;
if (n[m] == year) {
idx = m;
break;
}
else if (n[m] < year) {
l = m + 1;
}
else {
h = m - 1;
}
}
if (idx == -1)
System.out.println("Record does not exist");
else
System.out.println("Record exists");
}
}
Output
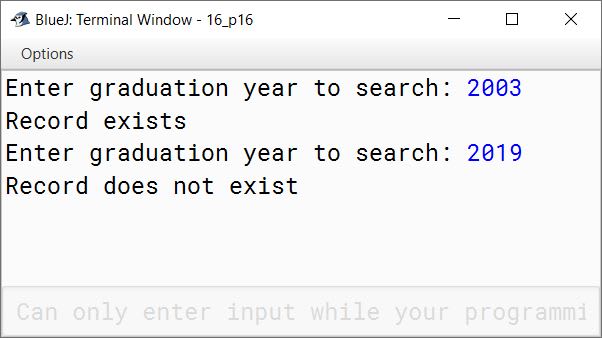