Section A
Question 1(i)
Return data type of isLetter(char) is ............... .
- Boolean
- boolean
- bool
- char
Answer
boolean
Reason — isLetter() method returns true if the specified character is a letter else, it returns false. Thus, the return data type of isLetter(char) is boolean.
Question 1(ii)
Method that converts a character to uppercase is ............... .
- toUpper()
- ToUpperCase()
- toUppercase()
- toUpperCase(char)
Answer
toUpperCase(char)
Reason — Method that converts a character to uppercase is toUpperCase(char) method.
Question 1(iii)
Give output of the following String methods:
"SUCESS".indexOf('S') + "SUCCESS".lastIndexOf('S')
- 0
- 5
- 6
- -5
Answer
6
Reason — indexOf() returns the index of the first occurrence of the specified character within the string and lastIndexOf() returns the index of the last occurrence of the specified character within the string. Since a string begins with index 0, the given methods are evaluated as follows:
"SUCESS".indexOf('S') + "SUCCESS".lastIndexOf('S')
⇒ 0 + 6
⇒ 6
Question 1(iv)
Corresponding wrapper class of float data type is ............... .
- FLOAT
- float
- Float
- Floating
Answer
Float
Reason — Corresponding wrapper class of float data type is Float.
Question 1(v)
............... class is used to convert a primitive data type to its corresponding object.
- String
- Wrapper
- System
- Math
Answer
Wrapper
Reason — Wrapper class is used to convert a primitive data type to its corresponding object.
Question 1(vi)
Give the output of the following code:
System.out.println("Good".concat("Day"));
- GoodDay
- Good Day
- Goodday
- goodDay
Answer
GoodDay
Reason — concat() method is used to join two strings. Thus, "Good" and "Day" are joined together and printed as "GoodDay".
Question 1(vii)
A single dimensional array contains N elements. What will be the last subscript?
- N
- N - 1
- N - 2
- N + 1
Answer
N - 1
Reason — The index of an array begins from 0 and continues till Size - 1. Thus, if an array has N elements, the last subscript/index will be N - 1.
Question 1(viii)
The access modifier that gives least accessibility is:
- private
- public
- protected
- package
Answer
private
Reason — A data member or member method declared as private is only accessible inside the class in which it is declared. Thus, it gives the least accessibility.
Question 1(ix)
Give the output of the following code :
String A = "56.0", B = "94.0";
double C = Double.parseDouble(A);
double D = Double.parseDouble(B);
System.out.println((C+D));
- 100
- 150.0
- 100.0
- 150
Answer
150.0
Reason — parseDouble() method returns a double value represented by the specified string. Thus, double values 56.0 and 94.0 are stored in C and D, respectively. Both C and D are added and 150.0 (56.0 + 94.0) is printed on the screen.
Question 1(x)
What will be the output of the following code?
System.out.println("Lucknow".substring(0,4));
- Lucknow
- Luckn
- Luck
- luck
Answer
Luck
Reason — The substring() method returns a substring beginning from the startindex and extending to the character at index endIndex - 1. Since a string index begins at 0, the character at startindex (0) is 'L' and the character at the endIndex (4-1 = 3) is 'k'. Thus, "Luck" is extracted and printed on the screen.
Section B
Question 2
Define a class to perform binary search on a list of integers given below, to search for an element input by the user, if it is found display the element along with its position, otherwise display the message "Search element not found".
2, 5, 7, 10, 15, 20, 29, 30, 46, 50
import java.util.Scanner;
public class KboatBinarySearch
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = {2, 5, 7, 10, 15, 20, 29, 30, 46, 50};
System.out.print("Enter number to search: ");
int n = in.nextInt();
int l = 0, h = arr.length - 1, index = -1;
while (l <= h) {
int m = (l + h) / 2;
if (arr[m] < n)
l = m + 1;
else if (arr[m] > n)
h = m - 1;
else {
index = m;
break;
}
}
if (index == -1) {
System.out.println("Search element not found");
}
else {
System.out.println(n + " found at position " + index);
}
}
}
Output
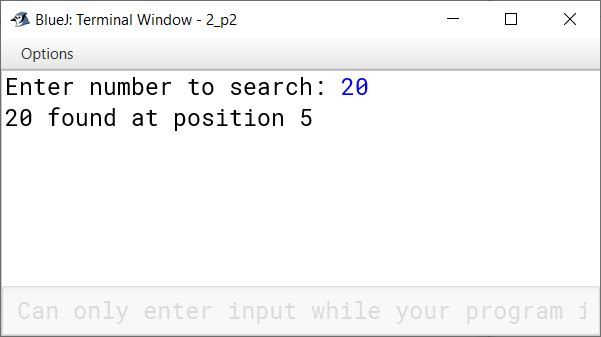
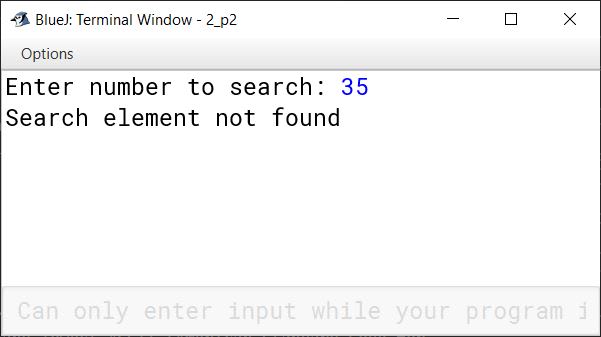
Question 3
Define a class to declare a character array of size ten. Accept the characters into the array and display the characters with highest and lowest ASCII (American Standard Code for Information Interchange) value.
EXAMPLE :
INPUT:
'R', 'z', 'q', 'A', 'N', 'p', 'm', 'U', 'Q', 'F'
OUTPUT :
Character with highest ASCII value = z
Character with lowest ASCII value = A
import java.util.Scanner;
public class KboatASCIIVal
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
char ch[] = new char[10];
int len = ch.length;
System.out.println("Enter 10 characters:");
for (int i = 0; i < len; i++)
{
ch[i] = in.nextLine().charAt(0);
}
char h = ch[0];
char l = ch[0];
for (int i = 1; i < len; i++)
{
if (ch[i] > h)
{
h = ch[i];
}
if (ch[i] < l)
{
l = ch[i];
}
}
System.out.println("Character with highest ASCII value: " + h);
System.out.println("Character with lowest ASCII value: " + l);
}
}
Output
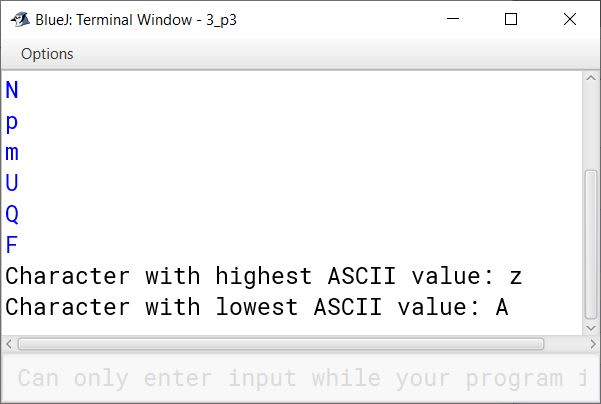
Question 4
Define a class to declare an array of size twenty of double datatype, accept the elements into the array and perform the following :
Calculate and print the product of all the elements.
Print the square of each element of the array.
import java.util.Scanner;
public class KboatSDADouble
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
double arr[] = new double[20];
int l = arr.length;
double p = 1.0;
System.out.println("Enter 20 numbers:");
for (int i = 0; i < l; i++)
{
arr[i] = in.nextDouble();
}
for (int i = 0; i < l; i++)
{
p *= arr[i];
}
System.out.println("Product = " + p);
System.out.println("Square of array elements :");
for (int i = 0; i < l; i++)
{
double sq = Math.pow(arr[i], 2);
System.out.println(sq + " ");
}
}
}
Output
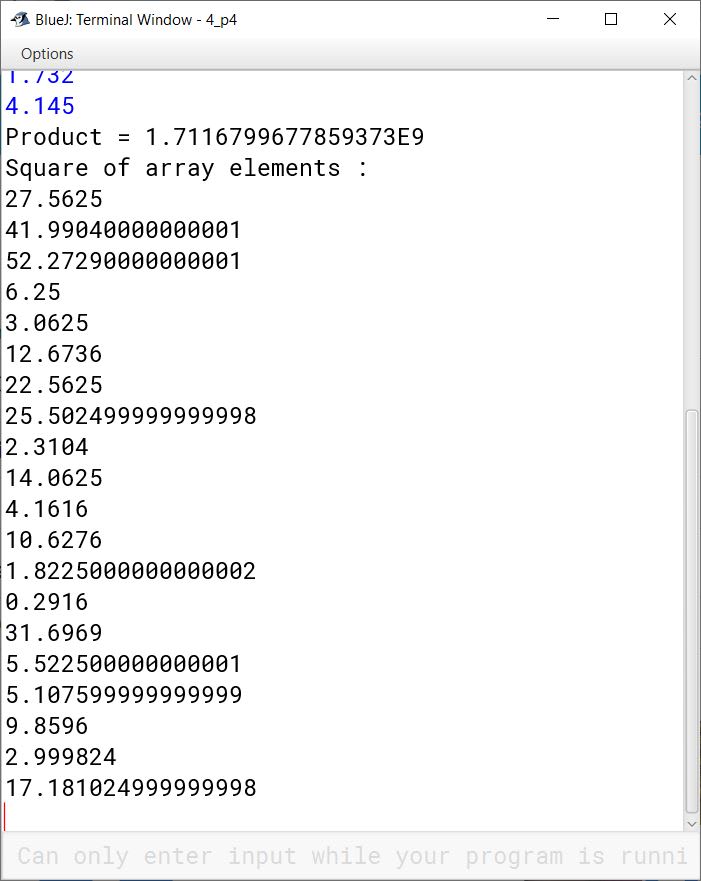
Question 5
Define a class to accept a string, and print the characters with the uppercase and lowercase reversed, but all the other characters should remain the same as before.
EXAMPLE:
INPUT : WelCoMe_2022
OUTPUT : wELcOmE_2022
import java.util.Scanner;
public class KboatChangeCase
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.println("Enter a string:");
String str = in.nextLine();
int len = str.length();
String rev = "";
for (int i = 0; i < len; i++)
{
char ch = str.charAt(i);
if (Character.isLetter(ch))
{
if(Character.isUpperCase(ch))
{
rev += Character.toLowerCase(ch);
}
else
{
rev += Character.toUpperCase(ch);
}
}
else
{
rev += ch;
}
}
System.out.println(rev);
}
}
Output
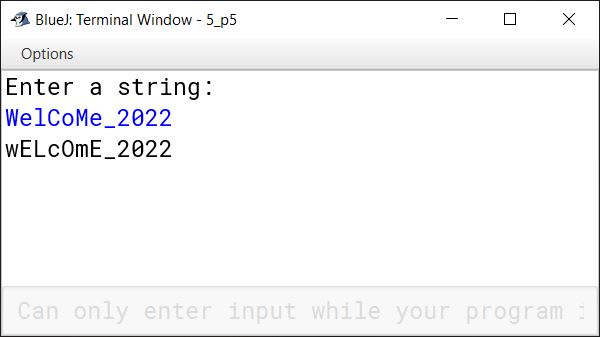
Question 6
Define a class to declare an array to accept and store ten words. Display only those words which begin with the letter 'A' or 'a' and also end with the letter 'A' or 'a'.
EXAMPLE :
Input : Hari, Anita, Akash, Amrita, Alina, Devi Rishab, John, Farha, AMITHA
Output: Anita
Amrita
Alina
AMITHA
import java.util.Scanner;
public class KboatWords
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
String names[] = new String[10];
int l = names.length;
System.out.println("Enter 10 names : ");
for (int i = 0; i < l; i++)
{
names[i] = in.nextLine();
}
System.out.println("Names that begin and end with letter A are:");
for(int i = 0; i < l; i++)
{
String str = names[i];
int len = str.length();
char begin = Character.toUpperCase(str.charAt(0));
char end = Character.toUpperCase(str.charAt(len - 1));
if (begin == 'A' && end == 'A') {
System.out.println(str);
}
}
}
}
Output
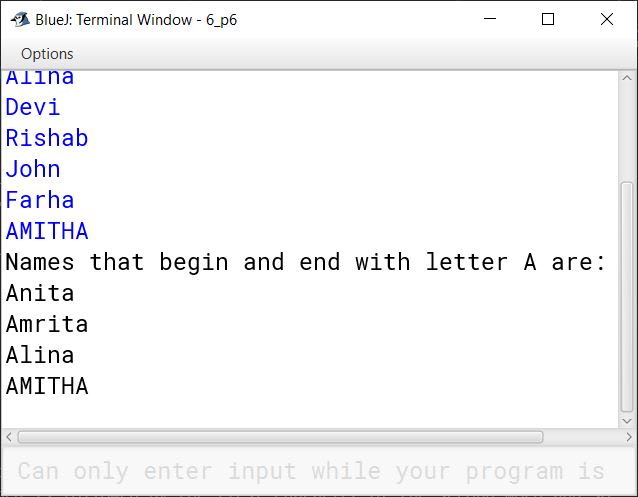
Question 7
Define a class to accept two strings of same length and form a new word in such a way that, the first character of the first word is followed by the first character of the second word and so on.
Example :
Input string 1 – BALL
Input string 2 – WORD
OUTPUT : BWAOLRLD
import java.util.Scanner;
public class KboatStringMerge
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.println("Enter String 1: ");
String s1 = in.nextLine();
System.out.println("Enter String 2: ");
String s2 = in.nextLine();
String str = "";
int len = s1.length();
if(s2.length() == len)
{
for (int i = 0; i < len; i++)
{
char ch1 = s1.charAt(i);
char ch2 = s2.charAt(i);
str = str + ch1 + ch2;
}
System.out.println(str);
}
else
{
System.out.println("Strings should be of same length");
}
}
}
Output
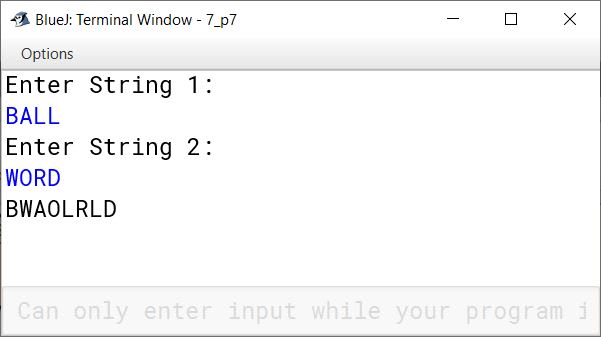
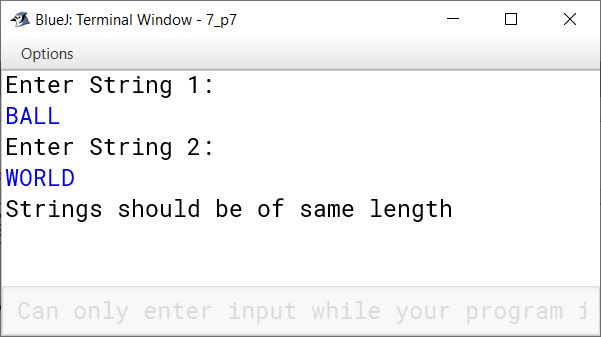