Which operator is used in Python to import all modules from packages ?
.
operator*
operator->
symbol,
operator
Answer
*
operator
Reason — The syntax to import all modules from package is : from <modulename> import *
. According to the syntax, *
operator is used to import all modules from a package.
Which file must be part of the folder containing Python module files to make it importable Python package ?
- init.py
- __setup__.py
- __init__.py
- setup.py
Answer
__init__.py
Reason — The file __init__.py in a folder, indicates it is an importable Python package, without __init__.py, a folder is not considered a Python package.
In Python which is the correct method to load a module math ?
- include math
- import math
- #include<math.h>
- using math
Answer
import math
Reason — The syntax for importing whole module is : import modulename
. According to the syntax, the correct method to load a module math is import math
.
Which is the correct command to load just the tempc method from a module called usable ?
- import usable, tempc
- import tempc from usable
- from usable import tempc
- import tempc
Answer
from usable import tempc
Reason — The syntax for importing single method form a module is : from <module> import <objectname>
. According to the syntax, the correct method is from usable import tempc
.
What is the extension of Python library modules ?
- .mod
- .lib
- .code
- .py
Answer
.py
Reason — A Python library module has the .py extension.
A .py file containing constants/variables, classes, functions etc. related to a particular task and can be used in other programs is called
- module
- library
- classes
- documentation
Answer
module
Reason — A Python module is a file (.py file) containing variables, class definitions, statements and functions related to a particular task and can be used in other programs.
The collection of modules and packages that together cater to a specific type of applications or requirements, is called ...............
- module
- library
- classes
- documentation
Answer
library
Reason — A library refers to a collection of modules and packages that together cater to a specific type of applications or requirements.
An independent triple quoted string given inside a module, containing documentation related information is a ...............
- Documentation string
- docstring
- dstring
- stringdoc
Answer
docstring
Reason — The docstrings are triple quoted strings in a Python module/program which are displayed as documentation when help (<module-or-program-name>) command is issued.
The help <module> statement displays ............... from a module.
- constants
- functions
- classes
- docstrings
Answer
docstrings
Reason — Docstrings of a module are displayed as documentation, when help <module> statement is issued.
Which command(s) modifies the current namespace with the imported object name ?
- import <module>
- import <module1>, <module2>
- from <module> import <object>
- from <module> import *
Answer
from <module> import <object>
from <module> import *
Reason —
from <module> import <object>
— This syntax is used to import a specific object from a module into the current namespace.from <module> import *
— This syntax is used to import all objects from the module into the current namespace.
Which command(s) creates a separate namespace for each of the imported module ?
- import <module>
- import <module1>, <module2>
- from <module> import <object>
- from <module> import *
Answer
import <module>
import <module1>, <module2>
Reason —
import <module>
— This syntax is used to import an entire module. It creates a new namespace with the same name as that of the module.import <module1>, <module2>
— This syntax is used to import multiple modules. It creates separate namespaces for each module, with names corresponding to the module names.
Which of the following random module functions generates a floating point number ?
- random()
- randint()
- uniform()
- all of these
Answer
random()
uniform()
Reason — Random module functions random() and uniform()
both return random floating point number .
Which of the following random module functions generates an integer ?
- random()
- randint()
- uniform()
- all of these
Answer
randint()
Reason — Random module function randint()
returns a random integer.
Which file must be a part of a folder to be used as a Python package ?
- package.py
- __init__.py
- __package__.py
- __module__.py
Answer
__init__.py
Reason — For a folder containing Python files to be recognized as a package, an __init__.py file (even if empty) must be part of the folder.
A python module has ............... extension.
- .mod
- .imp
- .py
- .mpy
Answer
.py
Reason — A Python module has a .py extension.
Which of the following is not a function/method of the random module in Python ?
- randfloat()
- randint()
- random()
- randrange()
Answer
randfloat()
Reason — Some most common random number generator functions in random module are random(), randint(), uniform(), randrange(). Hence, randfloat() is not a function of random module.
The file __init__.py must be the part of the folder holding library files and other definitions in order to be treated as importable package.
A library refers to a collection of modules that together cater to specific type of needs or applications.
A Python module is a file (.py file) containing variables, class definitions, statements and functions related to a particular task.
The uniform() function is the part of random module.
The capwords() function is the part of string module.
A Python program and a Python module means the same.
Answer
False
Reason — A Python program is a set of instructions or code written in the Python programming language that performs a specific task or a series of tasks. A Python module is a file containing Python code and is saved with a .py extension. Hence, Python program and module are not same.
A Python program and a Python module have the same .py file extension.
Answer
True
Reason — A Python program is saved in a .py file, which can be executed to perform a specific task. A Python module is also a .py file containing Python code, but it's meant to be imported and used in other Python programs or modules to reuse code. So, both Python programs and modules share the same file extension, i.e., .py.
The import <module> statement imports everything in the current namespace of the Python program.
Answer
False
Reason — The import <module>
statement imports entire module but it creates a new namespace with the same name as that of the module.
Any folder having .py files is a Python package.
Answer
False
Reason — Not all folders having multiple .py files are packages. In order for a folder containing Python files to be recognized as a package, an __init__.py file must be part of the folder.
A folder having .py files along with a special file i.e, __init__.py in it is an importable Python package.
Answer
True
Reason — The file __init__.py (even if empty) in a folder, indicates it is an importable Python package, without __init__.py, a folder is not considered a Python package.
The statement from <module> import <objects> is used to import a module in full.
Answer
False
Reason — The statement from <module> import <objects>
is used to import some selected items, not all from a module.
Assertion. The documentation for a Python module should be written in triple-quoted strings.
Reason. The docstrings are triple-quoted strings in Python that are displayed as documentation when help <module> command is issued.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
Docstrings are typically written within triple-quoted strings (i.e., using """ or '''), which allows for multiline strings. When the help<module>
command is issued in Python, it retrieves and displays the documentation string (docstring) associated with the specified modules, classes, functions, methods.
Assertion. After importing a module through import statement, all its function definitions, variables, constants etc. are made available in the program.
Reason. Imported module's definitions do not become part of the program's namespace if imported through an import <module> statement.
Answer
(b)
Both Assertion and Reason are true but Reason is not the correct explanation of Assertion.
Explanation
The import <module>
statement imports the entire module, making its contents available in a new namespace with the same name as the module. They do become accessible in the program's namespace through dot notation, but they are not added directly to the current namespace.
Assertion. If an item is imported through from <module> import <item> statement then you do not use the module name along with the imported item.
Reason. The from <module> import command modifies the namespace of the program and adds the imported item to it.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
The from <module> import <item>
command directly imports the specified item into the current namespace. This means that the imported item becomes part of the program's namespace and can be accessed directly without using the module name.
Assertion. Python's built-in functions, which are part of the standard Python library, can directly be used without specifying their module name.
Reason. Python's standard library's built-in functions are made available by default in the namespace of a program.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
Python's built-in functions such as print()
, len()
, range()
etc, are automatically added to the program's namespace from the Python's standard library. They can be used directly without importing any modules or specifying their module name.
Assertion. Python offers two statements to import items into the current program : import and from <module> import, which work identically.
Reason. Both import and from <module> import bring the imported items into the current program.
Answer
(e)
Both Assertion and Reason are false or not fully true.
Explanation
The import <module>
statement imports the entire module into the new namespace setup with same name as that of module, while the from <module> import <item>
statement imports specific items from the module into the current namespace. Hence, they both are different statements.
What is the significance of Modules ?
Answer
The significance of Python modules is as follows:
- Modules reduce complexity of programs to some degree.
- Modules create a number of well-defined, documented boundaries within the program.
- Contents of modules can be reused in other programs, without having to rewrite or recreate them.
What are docstrings ? What is their significance ? Give example to support your answer.
Answer
The docstrings are triple quoted strings in a Python module/program which are displayed as documentation when help (<module-or-program-name>) command is displayed.
Significance of docstrings is as follows:
- Documentation — Docstrings are used to document Python modules, classes, functions, and methods.
- Readability — Well-written docstrings improve code readability by providing clear explanations of the code's functionality.
- Interactive Help — Docstrings are displayed as documentation when help (<module-or-program-name>) command is displayed.
For example:
# tempConversion.py
"""Conversion functions between fahrenheit and centigrade"""
# Functions
def to_centigrade(x):
"""Returns: x converted to centigrade"""
return 5 * (x - 32) / 9.0
def to_fahrenheit(x):
"""Returns: x converted to fahrenheit"""
return 9 * x / 5.0 + 32
# Constants
FREEZING_C = 0.0 #water freezing temp.(in celcius)
FREEZING_F = 32.0 #water freezing temp.(in fahrenheit)
import tempConversion
help(tempConversion)
Help on module tempConversion:
NAME
tempConversion-Conversion functions between fahrenheit and centigrade
FILE
c:\python37\pythonwork\tempconversion.py
FUNCTIONS
to_centigrade(x)
Returns : x converted to centigrade
to_fahrenheit(x)
Returns : x converted to fahrenheit
DATA
FREEZING_C = 0.0
FREEZING_F = 32.0
What is a package ? How is a package different from module ?
Answer
A package is collection of Python modules under a common namespace, created by placing different modules on a single directory along with some special files (such as __init__.py).
Difference between package and module:
Package | Module |
---|---|
A package is a collection of Python modules under a common namespace organized in directories. | A module is a single Python file containing variables, class definitions, statements and functions related to a particular task. |
For a directory to be treated as a Python package, __init__.py (which can be empty) should be present. | __init__.py is not required for a Python module. |
Packages can contain sub-packages, forming a nested structure. | Modules cannot be nested. |
What is a library ? Write procedure to create own library in Python.
Answer
A library refers to a collection of modules that together cater to specific type of requirements or applications.
The procedure to create own library in Python is shown below:
- Create a package folder having the name of the library.
- Add module files (.py files containing actual code functions) to this package folder.
- Add a special file __init__.py to it(even if the file is empty).
- Attach this package folder to site-packages folder of Python installation.
What is the use of file __init__.py in a package even when it is empty ?
Answer
The use of file __init__.py in a package even when it is empty is that without the __init__.py file, Python will not look for submodules inside that directory, so attempts to import the module will fail. Hence, the file __init__.py in a folder, indicates it is an importable Python package.
What is the importance of site-packages folder of Python installation ?
Answer
The importance of site-packages folder of Python installation is that we can easily import a library and package using import command in Python only if it is attached to site-packages folder as this is the default place from where python interpreter imports Python library and packages.
How are following import statements different ?
(a) import X
(b) from X import *
(c) from X import a, b, c
Answer
(a) import X
— This statement is used to import entire module X. All the functions are imported in a new namespace setup with same name as that of the module X. To access one of the functions, we have to specify the name of the module (X) and the name of the function, separated by a dot. This format is called dot notation.
(b) from X import *
— This statement is used to import all the items from module X in the current namespace. We can use all defined functions, variables etc from X module, without having to prefix module's name to imported item name.
(c) from X import a, b, c
— This statement is used to import a, b, c objects from X module in the current namespace. We can use a, b, c objects from X module, without having to prefix module's name to imported item name.
What is Python PATH variable ? What is its significance ?
Answer
PYTHON PATH VARIABLE is an environment variable used by python to specify directories where the python interpreter will look in when importing modules.
The significance of the PYTHON PATH variable lies in its ability to extend python's module search path. By adding directories to the PYTHON PATH variable, we can make python aware of additional locations where our custom modules or libraries are located. Overall, the PYTHON PATH variable provides flexibility and control over how python locates modules and packages, allowing us to customize the module search path according to our needs.
In which order Python looks for the function/module names used by you.
Answer
Python looks for the module names in the below order :
- Built-in Modules — Python first looks for the module in the built-in modules that are part of the Python standard library.
- Directories in sys.path — If the module is not found in the built-in modules, Python searches for it in the directories listed in the sys.path variable. The directories in sys.path typically include the current directory, directories specified by the PYTHON PATH environment variable, and other standard locations such as the site-packages directory.
- Current Directory — Python also looks for the module in the current directory where the Python script or interactive session is running.
What is the usage of help( ) and dir( ) functions.
Answer
help() function — This function is used to display docstrings of a module as documentation. The syntax is : help(<module-name>)
dir() function — This function is used to display all the names defined inside the module. The syntax is : dir(<module-name>)
Name the Python Library modules which need to be imported to invoke the following functions :
(i) log()
(ii) pow()
(iii) cos
(iv) randint
(v) sqrt()
Answer
The Python Library modules required to be imported for these functions are:
Functions | Python library module |
---|---|
log() | math |
pow() | Built-in function |
cos | math |
randint | Random |
sqrt() | math |
What is dot notation of referring to objects inside a module ?
Answer
After importing a module using import module
statement, to access one of the functions, we have to specify the name of the module and the name of the function, separated by a dot. This format is called dot notation.
For example:
import math
print(math.pi)
print(math.sqrt(25))
Why should the from <module> import <object> statement be avoided to import objects ?
Answer
The from <module> import <object> statement should be avoided to import objects because it imports objects directly into the current namespace. If a program already has a variable or function with the same name as the one imported via the module, the imported object will overwrite the existing variable or function, leading to potential name clashes because there cannot be two variables with the same name in one namespace.
What do you understand by standard library of Python ?
Answer
Python standard library is distributed with Python that contains modules for various types of functionalities. Some commonly used modules of Python standard library are math module, random module, cmath module etc.
Explain the difference between import <module>
and from <module> import
statements, with examples.
Answer
import <module> statement | from <module> import statement |
---|---|
It imports entire module. | It imports single, multiple or all objects from a module. |
To access one of the functions, we have to specify the name of the module and the name of the function, separated by a dot. This format is called dot notation. The syntax is : <module-name>.<function-name>() | To access functions, there is no need to prefix module's name to imported item name. The syntax is : <function-name> |
Imports all its items in a new namespace with the same name as of the module. | Imports specified items from the module into the current namespace. |
This approach does not cause any problems. | This approach can lead to namespace pollution and name clashes if multiple modules import items with the same name. |
For example: import math print(math.pi) print(math.sqrt(25)) | For example: from math import pi, sqrt print(pi) print(sqrt(25)) |
Create module tempConversion.py as given in Fig. 4.2 in the chapter. If you invoke the module with two different types of import statements, how would the function call statement for imported module's functions be affected ?
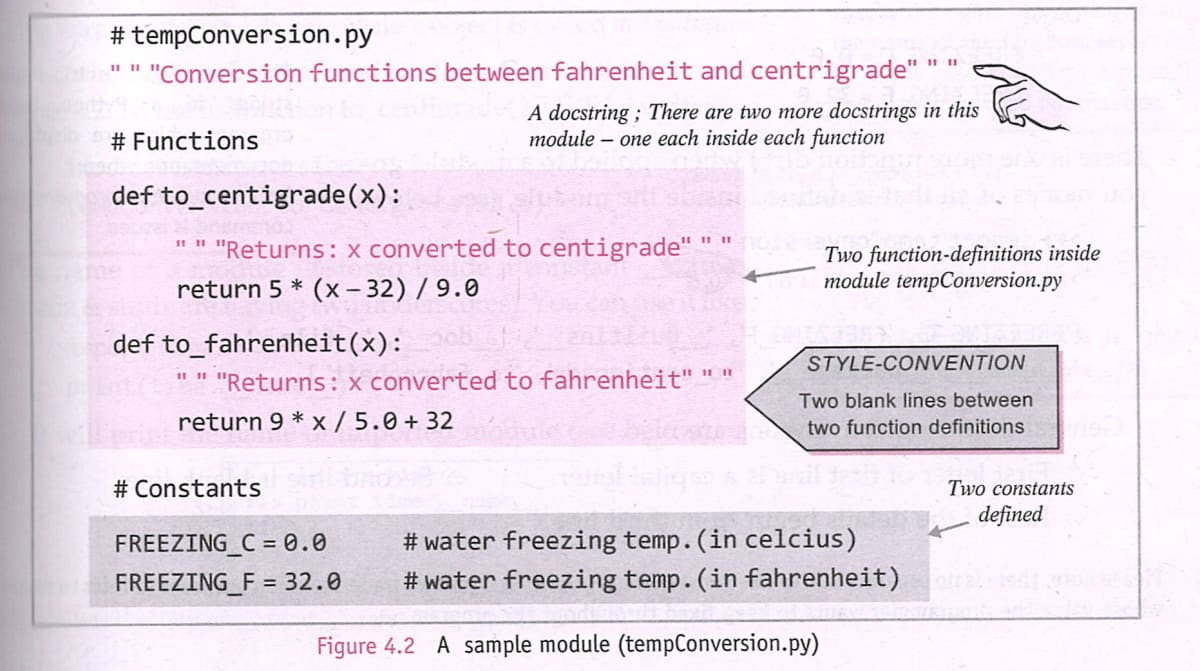
Answer
If we invoke the tempConversion module with two different types of import statements (import tempConversion
and from tempConversion import *
), the way we call the module's functions will be affected as follows:
1. import tempConversion
— This imports the entire module in a new namespace setup with the same name as that of the module tempConversion. To call the module's functions, we need to use the dot notation tempConversion.<function_name>
.
For example:
import tempConversion
tempConversion.to_centigrade(32)
tempConversion.to_fahrenheit(0)
2. from tempConversion import *
— This imports all objects (functions, variables, etc.) from the module into the current namespace. This approach imports all objects directly into the current namespace, so we can call the module's functions without using the module name.
For example:
from tempConversion import *
to_centigrade(32)
to_fahrenheit(0)
A function checkMain() defined in module Allchecks.py is being used in two different programs. In program 1 as Allchecks.checkMain(3, 'A')
and in program 2 as checkMain(4, 'Z')
. Why are these two function-call statements different from one another when the function being invoked is just the same ?
Answer
Allchecks.checkMain(3, 'A')
and checkMain(4, 'Z')
these two function-call statements are different from one another when the function being invoked is same because in Allchecks.checkMain(3, 'A')
statement, Allchecks.py module is imported using import Allchecks
import statement in a new namespace created with the same name as that of module name and hence they are called by dot notation whereas in checkMain(4, 'Z')
statement Allchecks.py module is imported using from Allchecks import checkMain
import statement in the current namespace and hence its module name is not specified along with the function name.
Given below is semi-complete code of a module basic.py :
#
"""..............."""
def square(x):
"""..............."""
return mul(x, x)
...............mul(x, y):
"""..............."""
return x * y
def div(x, y):
"""..............."""
return float(x)/y
...............fdiv(x, y)...............
"""..............."""
...............x//y
def floordiv(x, y)...............
...............fdiv(x, y)
Complete the code. Save it as a module.
Answer
# basic.py
"""This module contains basic mathematical operations."""
def square(x):
"""Returns the square of a number."""
return mul(x, x)
def mul(x, y):
"""Returns the product of two numbers."""
return x * y
def div(x, y):
"""Returns the result of dividing x by y."""
return float(x) / y
def fdiv(x, y):
"""Returns the result of floor division of x by y."""
return x // y
def floordiv(x, y):
return fdiv(x, y)
The code is saved with .py extension to save it as module.
After importing the above module, some of its functions are executed as per following statements. Find errors, if any:
(a) square(print 3)
(b) basic.div()
(c) basic.floordiv(7.0, 7)
(d) div(100, 0)
(e) basic.mul(3, 5)
(f) print(basic.square(3.5))
(g) z = basic.div(13, 3)
Answer
(a) square(print 3)
— print function should not be there as parameter in the function call. So the correct function call statement is square(3)
. Also, to call square function without prefixing the module name, it must be imported as from basic import square
.
(b) basic.div()
— The div() function requires two arguments but none are provided. So the correct statement is basic.div(x, y)
.
(c) basic.floordiv(7.0, 7)
— There is no error.
(d) div(100, 0)
— This will result in a runtime error of ZeroDivisionError as we are trying to divide a number by zero. The second argument of the function call should be any number other than 0. For example, div(100, 2)
. Also, to call div function without prefixing the module name, it must be imported as from basic import div
.
(e) basic.mul(3, 5) — There is no error.
(f) print(basic.square(3.5)) — There is no error.
(g) z = basic.div(13, 3) — There is no error.
Import the above module basic.py and write statements for the following :
(a) Compute square of 19.23.
(b) Compute floor division of 1000.01 with 100.23.
(c) Compute product of 3, 4 and 5. (Hint. use a function multiple times).
(d) What is the difference between basic.div(100, 0) and basic.div(0, 100)?
Answer
The statements are given in the program below:
import basic
# (a) Compute square of 19.23
square_result = basic.square(19.23)
print("Square of 19.23:", square_result)
# (b) Compute floor division of 1000.01 with 100.23
floor_div_result = basic.floordiv(1000.01, 100.23)
print("Floor division of 1000.01 by 100.23:", floor_div_result)
# (c) Compute product of 3, 4 and 5
product_result = basic.mul(basics.mul(3, 4), 5)
print("Product of 3, 4, and 5:", product_result)
# (d)
result2 = basic.div(0, 100)
result1 = basic.div(100, 0)
print("Result of basic.div(100, 0):", result1)
print("Result of basic.div(0, 100):", result2)
Square of 19.23: 369.79290000000003
Floor division of 1000.01 by 100.23: 9.0
Product of 3, 4, and 5: 60
Result of basic.div(0, 100): 0.0
ZeroDivisionError
(d) basic.div(100, 0)
results in a ZeroDivisionError because division by zero is not defined whereas basic.div(0, 100)
results in 0.0 as the quotient of '0 / 100'.
Suppose that after we import the random module, we define the following function called diff
in a Python session :
def diff():
x = random.random() - random.random()
return(x)
What would be the result if you now evaluate
y = diff()
print(y)
at the Python prompt ? Give reasons for your answer.
Answer
import random
def diff():
x = random.random() - random.random()
return(x)
y = diff()
print(y)
0.006054151450219258
-0.2927493777465524
Output will be a floating-point number representing the difference between two random numbers. Since every call to random() function of random module generates a new number, hence the output will be different each time we run the code.
What are the possible outcome(s) executed from the following code? Also specify the maximum and minimum values that can be assigned to variable NUMBER.
STRING = "CBSEONLINE"
NUMBER = random.randint(0, 3)
N = 9
while STRING[N] != 'L' :
print(STRING[N] + STRING[NUMBER] + '#', end = '')
NUMBER = NUMBER + 1
N = N - 1
- ES#NE#IO#
- LE#NO#ON#
- NS#IE#LO#
- EC#NB#IS#
Answer
The outcomes are as follows:
ES#NE#IO#
EC#NB#IS#
NUMBER is assigned a random integer between 0 and 3 using random.randint(0, 3). Therefore, the minimum value for NUMBER is 0 and the maximum value is 3.
Length of STRING
having value "CBSEONLINE" is 10. So the character at index 9 is "E". Since the value of N starts at 9 the first letter of output will be "E". This rules out LE#NO#ON# and NS#IE#LO# as possible outcomes as they don't start with "E". NUMBER
is a random integer between 0 and 3. So, the second letter of output can be any letter from "CBSE". After that, NUMBER
is incremented by 1 and N
is decremented by 1.
In next iteration, STRING[N]
will be STRING[8]
i.e., "N" and STRING[NUMBER]
will be the letter coming immediately after the second letter of the output in the string "CBSEONLINE" i.e., if second letter of output is "S" then it will be "E" and if second letter is "C" then it will be "B".
In the third iteration, STRING[N]
will be STRING[7]
i.e., "I" and STRING[NUMBER]
will be the letter coming immediately after the fourth letter of the output in the string "CBSEONLINE" i.e., if fourth letter of output is "E" then it will be "O" and if fourth letter is "B" then it will be "S".
After this the while
loop ends as STRING[N]
i.e., STRING[6]
becomes "L". Thus both, ES#NE#IO# and EC#NB#IS# can be the possible outcomes of this code.
Consider the following code :
import random
print(int(20 + random.random() * 5), end = ' ')
print(int(20 + random.random() * 5), end = ' ')
print(int(20 + random.random() * 5), end = ' ')
print(int(20 + random.random() * 5))
Find the suggested output options 1 to 4. Also, write the least value and highest value that can be generated.
- 20 22 24 25
- 22 23 24 25
- 23 24 23 24
- 21 21 21 21
Answer
23 24 23 24
21 21 21 21
The lowest value that can be generated is 20 and the highest value that can be generated is 24.
import random
— This line imports the random module.print(int(20 + random.random() * 5), end=' ')
— This line generates a random float number usingrandom.random()
, which returns a random float in the range (0.0, 1.0) exclusive of 1. The random number is then multiplied with 5 and added to 20. The int() function converts the result to an integer by truncating its decimal part. Thus,int(20 + random.random() * 5)
will generate an integer in the range [20, 21, 22, 23, 24]. The end=' ' argument in the print() function ensures that the output is separated by a space instead of a newline.- The next print statements are similar to the second line, generating and printing two more random integers within the same range.
The lowest value that can be generated is 20 because random.random() can generate a value of 0, and (0 * 5) + 20 = 20. The highest value that can be generated is 24 because the maximum value random.random() can return is just less than 1, and (0.999... * 5) + 20 = 24.999..., which is truncated to 24 when converted to an integer.
Consider the following code :
import random
print(100 + random.randint(5, 10), end = ' ' )
print(100 + random.randint(5, 10), end = ' ' )
print(100 + random.randint(5, 10), end = ' ' )
print(100 + random.randint(5, 10))
Find the suggested output options 1 to 4. Also, write the least value and highest value that can be generated.
- 102 105 104 105
- 110 103 104 105
- 105 107 105 110
- 110 105 105 110
Answer
105 107 105 110
110 105 105 110
The least value that can be generated is 105 and the highest value that can be generated is 110.
print(100 + random.randint(5, 10), end=' ')
— This statement generates a random integer between 5 and 10, inclusive, and then adds 100 to it. So, the suggested outputs range from 105 to 110.
The lowest value that can be generated is 100 + 5 = 105.
The highest value that can be generated is 100 + 10 = 110.
Consider the following package
music/ Top-level package
├── __init__.py
├── formats/ Subpackage for file format
│ ├── __init__.py
│ └── wavread.py
│ └── wavwrite.py
│
├── effects/ Subpackage for sound effects
│ └── __init__.py
│ └── echo.py
│ └── surround.py
│ └── reverse.py
│
└── filters/ Subpackage for filters
├── __init__.py
└── equalizer.py
└── vocoder.py
└── karaoke.py
Each of the above modules contain functions play(), writefile() and readfile().
(a) If the module wavwrite is imported using command import music.formats.wavwrite. How will you invoke its writefile() function ? Write command for it.
(b) If the module wavwrite is imported using command from music.formats import wavwrite. How will you invoke its writefile() function ? Write command for it.
Answer
(a) If the module wavwrite is imported using the command import music.formats.wavwrite
, we can invoke its writefile() function by using the module name followed by the function name:
import music.formats.wavwrite
music.formats.wavwrite.writefile()
(b) If the module wavwrite is imported using the command from music.formats import wavwrite
, we can directly invoke its writefile() function without prefixing the module name:
from music.formats import wavwrite
writefile()
What are the possible outcome(s) executed from the following code ? Also specify the maximum and minimum values that can be assigned to variable PICK.
import random
PICK = random.randint(0, 3)
CITY = ["DELHI", "MUMBAI", "CHENNAI", "KOLKATA"];
for I in CITY :
for J in range(1, PICK):
print(I, end = " ")
print()
- DELHIDELHI
MUMBAIMUMBAI
CHENNAICHENNAI
KOLKATAKOLKATA - DELHI
DELHIMUMBAI
DELHIMUMBAICHENNAI - DELHI
MUMBAI
CHENNAI
KOLKATA - DELHI
MUMBAIMUMBAI
KOLKATAKOLKATAKOLKATA
Answer
DELHI
MUMBAI
CHENNAI
KOLKATA
DELHIDELHI
MUMBAIMUMBAI
CHENNAICHENNAI
KOLKATAKOLKATA
The minimum value for PICK is 0 and the maximum value for PICK is 3.
import random
— Imports therandom
module.PICK = random.randint(0, 3)
— Generates a random integer between 0 and 3 (inclusive) and assigns it to the variablePICK
.CITY = ["DELHI", "MUMBAI", "CHENNAI", "KOLKATA"]
— Defines a list of cities.for I in CITY
— This loop iterates over each city in theCITY
.for J in range(1, PICK)
— This loop will iterate from 1 up to PICK - 1. The value ofPICK
can be an integer in the range [0, 1, 2, 3]. For the cases when value ofPICK
is 0 or 1, the loop will not execute asrange(1, 0)
andrange(1, 1)
will return empty range.
For the cases when value ofPICK
is 2 or 3, the names of the cities inCITY
will be printed once or twice, respectively. Hence, we get the possible outcomes of this code.
Write a Python program having following functions :
- A function with the following signature :
remove_letter(sentence, letter)
This function should take a string and a letter (as a single-character string) as arguments, returning a copy of that string with every instance of the indicated letter removed. For example, remove_letter("Hello there!", "e") should return the string "Hllo thr!".
Try implementing it using<str>.split()
function. - Write a function to do the following :
Try implementing the capwords() functionality using other functions, i.e., split(), capitalize() and join(). Compare the result with the capwords() function's result.
def remove_letter(sentence, letter):
words = sentence.split()
new_sentence = []
for word in words:
new_word = ''
for char in word:
if char.lower() != letter.lower():
new_word += char
new_sentence.append(new_word)
return ' '.join(new_sentence)
def capwords_custom(sentence):
words = sentence.split()
capitalized_words = []
for word in words:
capitalized_word = word.capitalize()
capitalized_words.append(capitalized_word)
return ' '.join(capitalized_words)
sentence = input("Enter a sentence: ")
letter = input("Enter a letter: ")
print("After removing letter:", remove_letter(sentence, letter))
from string import capwords
sentences_input = input("Enter a sentence: ")
sentences = sentences_input.split('.')
for sentence in sentences:
sentence = sentence.strip()
print("Custom capwords result:", capwords_custom(sentence))
print("Capwords result from string module:", capwords(sentence))
Enter a sentence: Hello there!
Enter a letter: e
After removing letter: Hllo thr!
Enter a sentence: python is best programming language
Custom capwords result: Python Is Best Programming Language
Capwords result from string module: Python Is Best Programming Language
Create a module lengthconversion.py that stores functions for various lengths conversion e.g.,
- miletokm() — to convert miles to kilometer
- kmtomile() — to convert kilometers to miles
- feettoinches()
- inchestofeet()
It should also store constant values such as value of (mile in kilometers and vice versa).
[1 mile = 1.609344 kilometer ; 1 feet = 12 inches]
Help() function should display proper information.
# lengthconversion.py
"""Conversion functions for various lengths"""
#Constants
MILE_TO_KM = 1.609344
KM_TO_MILE = 1 / MILE_TO_KM
FEET_TO_INCHES = 12
INCHES_TO_FEET = 1 / FEET_TO_INCHES
#Functions
def miletokm(miles):
"""Returns: miles converted to kilometers"""
return miles * MILE_TO_KM
def kmtomile(kilometers):
"""Returns: kilometers converted to miles"""
return kilometers * KM_TO_MILE
def feettoinches(feet):
"""Returns: feet converted to inches"""
return feet * FEET_TO_INCHES
def inchestofeet(inches):
"""Returns: inches converted to feet"""
return inches * INCHES_TO_FEET
5 miles to kilometers = 8.04672 km
5 kilometers to miles = 3.1068559611866697 miles
5 feet to inches = 60 inches
24 inches to feet = 2.0 feet
Create a module MassConversion.py that stores function for mass conversion e.g.,
1.kgtotonne() — to convert kg to tonnes
2. tonnetokg() — to convert tonne to kg 3. kgtopound() — to convert kg to pound
4. poundtokg() — to convert pound to kg
(Also store constants 1 kg = 0.001 tonne, 1 kg = 2.20462 pound)
Help( ) function should give proper information about the module.
# MassConversion.py
"""Conversion functions between different masses"""
#Constants
KG_TO_TONNE = 0.001
TONNE_TO_KG = 1 / KG_TO_TONNE
KG_TO_POUND = 2.20462
POUND_TO_KG = 1 / KG_TO_POUND
#Functions
def kgtotonne(kg):
"""Returns: kilogram converted to tonnes"""
return kg * KG_TO_TONNE
def tonnetokg(tonne):
"""Returns: tonne converted to kilogram"""
return tonne * TONNE_TO_KG
def kgtopound(kg):
"""Returns: kilogram converted to pound"""
return kg * KG_TO_POUND
def poundtokg(pound):
"""Returns: pound converted to kilogram"""
return pound * POUND_TO_KG
6 kilograms to tonnes = 0.006 tonnes
8 tonnes to kilograms = 8000.0 kilograms
5 kilograms to pound = 11.0231 pounds
18 pounds to kilograms = 8.164672369841515 kilograms
Create a package from above two modules as this :
Conversion
├──Length
│ └──Lengthconversion.py
└──Mass
└──Massconversion. py
Make sure that above package meets the requirements of being a Python package. Also, you should be able to import above package and/or its modules using import command.
Answer
- The basic structure of above package includes packages's name i.e., Conversion and all modules and subfolders.
- Create the directory structure having folders with names of package and subpackages. In the above package, we created two folders by names Length and Mass. Inside these folders, we created Lengthconversion.py and MassConversion.py modules respectively.
- Create __init__.py files in package and subpackage folders. We created an empty file and saved it as "__init__.py" and then copy this empty __init__.py file to the package and subpackage folders.
- Associate it with python installation. Once we have our package directory ready, we can associate it with Python by attaching it to Python's site-packages folder of current Python distribution in our computer.
- After copying our package folder in site-packages folder of our current Python installation, now it has become a Python library so that now we can import its modules and use its functions.
Directory structure is as follows:
Conversion/
├── __init__.py
├── Length/
│ ├── __init__.py
│ └── Lengthconversion.py
└── Mass/
├── __init__.py
└── MassConversion.py