If return statement is not used inside the function, the function will return:
- 0
- None object
- an arbitrary integer
- Error! Functions in Python must have a return statement.
Answer
None object
Reason — The default return value for a function that does not return any value explicitly is None
.
Which of the following keywords marks the beginning of the function block?
- func
- define
- def
- function
Answer
def
Reason — The function in Python is defined as per following syntax :
def function_name(parameters):
statements
According to this format, function definition begins with keyword def
.
What is the area of memory called, which stores the parameters and local variables of a function call ?
- a heap
- storage area
- a stack
- an array
Answer
a stack
Reason — A stack is the area of memory, which stores the parameters and local variables of a function call.
Find the errors in following function definitions :
def main() print ("hello")
def func2(): print(2 + 3)
def compute(): print (x * x)
square (a) return a * a
Answer
- Colon is missing at the end of function header.
- There is no error.
- The variable
x
is not defined. def
keyword at the beginning and colon at the end of function header are missing.
The corrected function definitions are:
def main(): print("hello")
def func2(): print(2 + 3)
def compute(x): print (x * x)
def square(a): return a * a
What is the default return value for a function that does not return any value explicitly ?
- None
- int
- double
- null
Answer
None
Reason — The default return value for a function that does not return any value explicitly is None
.
Which of the following items are present in the function header ?
- function name only
- both function name and parameter list
- parameter list only
- return value
Answer
both function name and parameter list
Reason — Function header is the first line of function definition that begins with keyword def
and ends with a colon (:), specifies the name of the function and its parameters.
Which of the following keywords marks the beginning of the function block ?
- func
- define
- def
- function
Answer
def
Reason — The function in Python is defined as per following format :
def function_name(parameters):
statements
According to this format, function definition begins with keyword def
.
What is the name given to that area of memory, where the system stores the parameters and local variables of a function call ?
- a heap
- storage area
- a stack
- an array
Answer
a stack
Reason — A stack is the area of memory, which stores the parameters and local variables of a function call.
Pick one the following statements to correctly complete the function body in the given code snippet.
def f(number):
#Missing function body
print(f(5))
- return "number"
- print(number)
- print("number")
- return number
Answer
return number
Reason — The syntax of return statement is :return <value>
According to this, the correct return statement is return number
.
Which of the following function headers is correct ?
- def f(a = 1, b):
- def f(a = 1, b, c = 2):
- def f(a = 1, b = 1, c = 2):
- def f(a = 1, b = 1, c = 2, d):
Answer
def f(a = 1, b = 1, c = 2):
Reason — In a function header, any parameter cannot have a default value unless all parameters appearing on its right have their default values.
Which of the following statements is not true for parameter passing to functions ?
- You can pass positional arguments in any order.
- You can pass keyword arguments in any order.
- You can call a function with positional and keyword arguments.
- Positional arguments must be before keyword arguments in a function call.
Answer
You can pass positional arguments in any order.
Reason — Positional arguments are passed to a function based on their positions in the function call statement. The first positional argument in the function call is assigned to the first parameter in the function definition, the second positional argument is assigned to the second parameter, and so on.
Which of the following is not correct in context of Positional and Default parameters in Python functions ?
- Default parameters must occur to the right of Positional parameters.
- Positional parameters must occur to the right of Default parameters.
- Positional parameters must occur to the left of Default parameters.
- All parameters to the right of a Default parameter must also have Default values.
Answer
Positional parameters must occur to the right of Default parameters.
Reason — The positional parameters must occur to the left of default parameters because in a function header, any parameter cannot have a default value unless all parameters appearing on its right have their default values.
Which of the following is not correct in context of scope of variables ?
- Global keyword is used to change value of a global variable in a local scope.
- Local keyword is used to change value of a local variable in a global scope.
- Global variables can be accessed without using the global keyword in a local scope.
- Local variables cannot be used outside its scope.
Answer
Local keyword is used to change value of a local variable in a global scope.
Reason — If you need to modify a local variable in global scope, you do so by passing it as an argument to a function and/or returning a modified value from the function.
Which of the following function calls can be used to invoke the below function definition ?def test(a, b, c, d)
- test(1, 2, 3, 4)
- test(a = 1, 2, 3, 4)
- test(a = 1, b = 2, c = 3, 4)
- test(a = 1, b = 2, c = 3, d = 4)
Answer
test(1, 2, 3, 4)
test(a = 1, b = 2, c = 3, d = 4)
Reason —
- The function call
test(1, 2, 3, 4)
passes four positional arguments 1, 2, 3, and 4 to the function. - The function call
test(a = 1, b = 2, c = 3, d = 4)
passes four keyword arguments, explicitly stating the parameter names (a, b, c, d) and their corresponding values.
Which of the following function calls will cause Error while invoking the below function definition ?def test(a, b, c, d)
- test(1, 2, 3, 4)
- test(a = 1, 2, 3, 4)
- test(a = 1, b = 2, c = 3, 4)
- test(a = 1, b = 2, c = 3, d = 4)
Answer
test(a = 1, 2, 3, 4)
test(a = 1, b = 2, c = 3, 4)
Reason — In both of these function call test(a = 1, 2, 3, 4)
and test(a = 1, b = 2, c = 3, 4)
, the syntax is incorrect because when using keyword arguments, all arguments following the first one must also be specified with keyword arguments.
For a function header as follows :def Calc(X,Y = 20):
Which of the following function calls will give an error ?
- Calc(15, 25)
- Calc(X = 15, Y = 25)
- Calc(Y = 25)
- Calc(X = 25)
Answer
Calc(Y = 25)
Reason — The function call statement must match the number and order of arguments as defined in the function definition. Here, the X
positional argument is missing.
What is a variable defined outside all the functions referred to as ?
- A static variable
- A global variable
- A local variable
- An automatic variable
Answer
A global variable
Reason — A global variable is a variable declared in top level segment (__main__) of a program and usable inside the whole program and all blocks contained within the program.
What is a variable defined inside a function referred to as
- A static variable
- A global variable
- A local variable
- An automatic variable
Answer
A local variable
Reason — A local variable is a variable declared in a function-body and it can be used only within this function and the other blocks contained under it.
Carefully observe the code and give the answer.
def function1(a):
a = a + '1'
a = a * 2
>>>function1("hello")
- indentation Error
- cannot perform mathematical operation on strings
- hello2
- hello2hello2
Answer
indentation error
Reason — The line a = a * 2
should be indented backwards otherwise it will give an error.
What is the result of this code ?
def print_double(x):
print(2 ** x)
print_double(3)
- 8
- 6
- 4
- 10
Answer
8
Reason — The code defines a function named print_double
that takes one parameter x
. Inside the function, it calculates 2 raised to the power of x using the exponentiation operator **
, and then prints the result. So, when we call print_double(3)
, we're passing 3 as an argument to the function. The function calculates 2 ** 3
, which is 8
, and prints the result.
What is the order of resolving scope of a name in a Python program ?
- B G E L
- L E G B
- G E B L
- L B E G
Answer
L E G B
Reason — When you access a variable from within a program or function, python follows name resolution rule, also known as LEGB rule. When python encounters a name (variable or function), it first searches the local scope (L), then the enclosing scope (E), then the global scope (G), and finally the built-in scope (B).
Which of the given argument types can be skipped from a function call ?
- positional arguments
- keyword arguments
- named arguments
- default arguments
Answer
default arguments
Reason — A default argument is an argument with a default value in the function header, making it optional in the function call. The function call may or may not have a value for it.
A function is a subprogram that acts on data and often returns a value.
Python names the top level segment (main program) as __main__.
In Python, program execution begins with first statement of __main__ segment.
The values being passed through a function-call statement are called arguments / actual parameters / actual arguments.
The values received in the function definition/header are called parameters / formal parameters / formal arguments.
A parameter having default value in the function header is known as a default parameter.
A default argument can be skipped in the function call statement.
Keyword arguments are the named arguments with assigned values being passed in the function call statement.
A void function also returns a None value to its caller.
By default, Python names the segment with top-level statements (main program) as __main__.
The flow of execution refers to the order in which statements are executed during a program run.
The default value for a parameter is defined in function header.
Non-default arguments can be placed before or after a default argument in a function definition.
Answer
False
Reason — In a function header, any parameter cannot have a default value unless all parameters appearing on its right have their default values. Hence, non-default arguments cannot follow default argument.
A parameter having default value in the function header is known as a default parameter.
Answer
True
Reason — A default parameter is a parameter having default value in the function header.
The first line of function definition that begins with keyword def
and ends with a colon (:), is also known as function header.
Answer
True
Reason — The first line of function definition that begins with keyword def
and ends with a colon (:), which specifies the name of the function and its parameter is called function header.
Variables that are listed within the parentheses of a function header are called function variables.
Answer
False
Reason — Variables that are listed within the parentheses of a function header are called parameters.
In Python, the program execution begins with first statement of __main__ segment.
Answer
True
Reason — The Python interpreter starts the execution of a program from top-level statements. The top-level statements are part of main program, __main__ .
Default parameters cannot be skipped in function call.
Answer
False
Reason — A default parameter in function header becomes optional in function call. Function call may or may not have value for default parameters.
The default values for parameters are considered only if no value is provided for that parameter in the function call statement.
Answer
True
Reason — The default values for parameters are considered only if no value is provided for that parameter in the function call statement.
A Python function may return multiple values.
Answer
True
Reason — A Python function may return multiple values i.e., more than one value from a function.
A void function also returns a value i.e., None to its caller.
Answer
True
Reason — A void function do not return a value but they return a legal empty value of Python i.e., None
to its caller.
Variables defined inside functions can have global scope.
Answer
False
Reason — Variables defined inside functions have only local scope. This means that they are only accessible within the function in which they are defined.
A local variable having the same name as that of a global variable, hides the global variable in its function.
Answer
True
Reason — Inside a function, you assign a value to a name which is already there in a global scope, Python won't use the global scope variable because it is an assignment statement and assignment statement creates a variable by default in current environment. That means a local variable having the same name as that of a global variable, hides the global variable in its function.
Assertion. A function is a subprogram.
Reason. A function exists within a program and works within it when called.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
A function is a subprogram that acts on data and often returns a value. It is a self-contained unit of code that performs a specific task within a larger program. Functions are defined within a program and can be called upon to execute a specific set of instructions when needed.
Assertion. Non-default arguments cannot follow default arguments in a function call.
Reason. A function call can have different types of arguments.
Answer
(b)
Both Assertion and Reason are true but Reason is not the correct explanation of Assertion.
Explanation
In a function call, any parameter cannot have a default value unless all parameters appearing on its right have their default values. Hence, non-default arguments cannot follow default arguments in a function call. Python supports three types of formal arguments :
- Positional arguments
- Default arguments
- keyword arguments
Assertion. A parameter having a default in function header becomes optional in function call.
Reason. A function call may or may not have values for default arguments.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
When a parameter in a function header has a default value, it means that if no value is provided for that parameter during the function call, the default value will be used. This effectively makes the parameter optional in the function call. When calling a function with default arguments, we have the option to either provide values for those arguments or omit them, in which case the default values will be used.
Assertion. A variable declared inside a function cannot be used outside it.
Reason. A variable created inside a function has a function scope.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
Variables declared inside a function are typically local to that function, meaning they exist only within the scope of the function and they can only be accessed and used within the function in which they are declared.
Assertion. A variable not declared inside a function can still be used inside the function if it is declared at a higher scope level.
Reason. Python resolves a name using LEGB rule where it checks in Local (L), Enclosing (E), Global (G) and Built-in scopes (B), in the given order.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
In Python, if a variable is not declared inside a function but is declared at a higher scope level (e.g., in the global scope or an enclosing scope), the function can still access and use that variable. Since Python follows the LEGB rule, variables declared at higher scope levels can be accessed within functions.
Assertion. A parameter having a default value in the function header is known as a default parameter.
Reason. The default values for parameters are considered only if no value is provided for that parameter in the function call statement.
Answer
(b)
Both Assertion and Reason are true but Reason is not the correct explanation of Assertion.
Explanation
A default parameter is a parameter in a function definition that has a predefined value. When we call a function with default parameters, if we don't provide a value for a parameter, the default value specified in the function header will be used instead.
Assertion. A function declaring a variable having the same name as a global variable, cannot use that global variable.
Reason. A local variable having the same name as a global variable hides the global variable in its function.
Answer
(a)
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation
Inside a function, you assign a value to a name which is already there in global scope, Python won't use the global scope variable because it is an assignment statement and assignment statement creates a variable by default in current environment. That means a local variable having the same name as a global variable hides the global variable in its function.
Assertion. If the arguments in a function call statement match the number and order of arguments as defined in the function definition, such arguments are called positional arguments.
Reason. During a function call, the argument list first contains default argument(s) followed positional argument(s).
Answer
(c)
Assertion is true but Reason is false.
Explanation
If the arguments in a function call statement match the number and order of arguments as defined in the function definition, such arguments are called positional arguments. During a function call, the argument list first contains positional argument followed by default arguments because any parameter cannot have a default value unless all parameters appearing on its right have their default values.
A program having multiple functions is considered better designed than a program without any functions. Why ?
Answer
A program having multiple functions is considered better designed than a program without any functions because
- It makes program handling easier as only a small part of the program is dealt with at a time, thereby avoiding ambiguity.
- It reduces program size.
- Functions make a program more readable and understandable to a programmer thereby making program management much easier.
What all information does a function header give you about the function ?
Answer
Function header is the first line of function definition that begins with keyword def
and ends with a colon (:), specifies the name of the function and its parameters.
What do you understand by flow of execution ?
Answer
Flow of execution refers to the order in which statements are executed during a program run.
What are arguments ? What are parameters ? How are these two terms different yet related ? Give example.
Answer
Arguments — The values being passed through a function-call statement are called arguments.
Parameters — The values received in the function definition/header are called parameters.
Arguments appear in function call statement whereas parameters appear in function header. The two terms are related because the values passed through arguments while calling a function are received by parameters in the function definition.
For example :
def multiply(a, b):
print(a * b)
multiply(3, 4)
Here, a and b are parameters while 3, 4 are arguments.
What is the utility of : (i) default arguments, (ii) keyword arguments ?
Answer
(i) Default arguments — Default arguments are useful in case a matching argument is not passed in the function call statement. They give flexibility to specify the default value for a parameter so that it can be skipped in the function call, if needed. However, still we cannot change the order of the arguments in the function call.
(ii) Keyword arguments — Keyword arguments are useful when you want to specify arguments by their parameter names during a function call. This allows us to pass arguments in any order, as long as we specify the parameter names when calling the function. It also makes the function call more readable and self-explanatory.
Explain with a code example the usage of default arguments and keyword arguments.
Answer
Default arguments — Default arguments are used to provide a default value to a function parameter if no argument is provided during the function call.
For example :
def greet(name, message="Hello"):
print(message, name)
greet("Alice")
greet("Bob", "Hi there")
Hello Alice
Hi there Bob
In this example, the message parameter has a default value of "Hello". If no message argument is provided during the function call, the default value is used.
Keyword arguments — Keyword arguments allow us to specify arguments by their parameter names during a function call, irrespective of their position.
def person(name, age, city):
print(name, "is", age, "years old and lives in", city)
person(age=25, name="Alice", city="New York")
person(city="London", name="Bob", age=30)
Alice is 25 years old and lives in New York
Bob is 30 years old and lives in London
In this example, the arguments are provided in a different order compared to the function definition. Keyword arguments specify which parameter each argument corresponds to, making the code more readable and self-explanatory.
Both default arguments and keyword arguments provide flexibility and improve the readability of code, especially in functions with multiple parameters or when calling functions with optional arguments.
Describe the different styles of functions in Python using appropriate examples.
Answer
The different styles of functions in Python are as follows :
Built-in functions — These are pre-defined functions and are always available for use. For example:
name = input("Enter your name: ") name_length = len(name) print("Length of your name:", name_length)
In the above example,
print()
,len()
,input()
etc. are built-in functions.User defined functions — These are defined by programmer. For example:
def calculate_area(length, width): area = length * width return area length = 5 width = 3 result = calculate_area(length, width) print("Area of the rectangle:", result)
In the above example,
calculate_area
is a user defined function.Functions defined in modules — These functions are pre-defined in particular modules and can only be used when corresponding module is imported. For example:
import math num = 16 square_root = math.sqrt(num) print("Square root of", num, ":", square_root)
In the above example,
sqrt
is a function defined in math module.
Differentiate between fruitful functions and non-fruitful functions.
Answer
Fruitful functions | Non-fruitful functions |
---|---|
Functions returning some value are called as fruitful functions. | Functions that does not return any value are called as non-fruitful functions. |
They are also called as non-void functions. | They are also called as void functions. |
They have return statements in the syntax : return<value> . | They may or may not have a return statement, but if they do, it typically appears as per syntax : return . |
Fruitful functions return some computed result in terms of a value. | Non-fruitful functions return legal empty value of Python, which is None , to their caller. |
Can a function return multiple values ? How ?
Answer
Yes, a function can return multiple values. To return multiple values from a function, we have to ensure following things :
1. The return statement inside a function body should be of the form :
return <value1/variable1/expression1>,<value2/variable2/expression2>,....
2. The function call statement should receive or use the returned values in one of the following ways :
(a) Either receive the returned values in form a tuple variable.
(b) Or we can directly unpack the received values of tuple by specifying the same number of variables on the left-hand side of the assignment in function call.
What is scope ? What is the scope resolving rule of Python ?
Answer
Scope refers to part(s) of program within which a name is legal and accessible. When we access a variable from within a program or function, Python follows name resolution rule, also known as LEGB rule. When Python encounters a name (variable or function), it first searches the local scope (L), then the enclosing scope (E), then the global scope (G), and finally the built-in scope (B).
What is the difference between local and global variable ?
Answer
Local variable | Global variable |
---|---|
A local variable is a variable defined within a function. | A global variable is a variable defined in the 'main' program. |
They are only accessible within the block in which they are defined. | They are accessible from anywhere within the program, including inside functions. |
These variables have local scope. | These variables have global scope. |
The lifetime of a local variable is limited to the block of code in which it is defined. Once the execution exits that block, the local variable is destroyed, and its memory is released. | Global variables persist throughout the entire execution of the program. They are created when the program starts and are only destroyed when the program terminates. |
When is global statement used ? Why is its use not recommended ?
Answer
If we want to assign some value to the global variable without creating any local variable then global statement is used. It is not recommended because once a variable is declared global in a function, we cannot undo the statement. That is, after a global statement, the function will always refer to the global variable and local variable cannot be created of the same name. Also, by using global statement, programmers tend to lose control over variables and their scopes.
Write the term suitable for following descriptions :
(a) A name inside the parentheses of a function header that can receive a value.
(b) An argument passed to a specific parameter using the parameter name.
(c) A value passed to a function parameter.
(d) A value assigned to a parameter name in the function header.
(e) A value assigned to a parameter name in the function call.
(f) A name defined outside all function definitions.
(g) A variable created inside a function body.
Answer
(a) Parameter
(b) Keyword argument
(c) Argument
(d) Default value
(e) Keyword argument
(f) Global variable
(g) Local variable
What do you understand by local and global scope of variables ? How can you access a global variable inside the function, if function has a variable with same name.
Answer
Local scope — Variables defined within a specific block of code, such as a function or a loop, have local scope. They are only accessible within the block in which they are defined.
Global scope — Variables defined outside of any specific block of code, typically at the top level of a program or module, have global scope. They are accessible from anywhere within the program, including inside functions.
To access a global variable inside a function, even if the function has a variable with the same name, we can use the global
keyword to declare that we want to use the global variable instead of creating a new local variable. The syntax is :
global<variable name>
For example:
def state1():
global tigers
tigers = 15
print(tigers)
tigers = 95
print(tigers)
state1()
print(tigers)
In the above example, tigers
is a global variable. To use it inside the function state1
we have used global
keyword to declare that we want to use the global variable instead of creating a new local variable.
What are the errors in following codes ? Correct the code and predict output :
total = 0;
def sum(arg1, arg2):
total = arg1 + arg2;
print("Total :", total)
return total;
sum(10, 20);
print("Total :", total)
Answer
total = 0
def sum(arg1, arg2):
total = arg1 + arg2
print("Total :", total)
return total
sum(10, 20)
print("Total :", total)
Total : 30
Total : 0
- There is an indentation error in second line.
- The return statement should be indented inside function and it should not end with semicolon.
- Function call should not end with semicolon.
What are the errors in following codes ? Correct the code and predict output :
def Tot(Number) #Method to find Total
Sum = 0
for C in Range (1, Number + 1):
Sum += C
RETURN Sum
print (Tot[3]) #Function Calls
print (Tot[6])
Answer
def Tot(Number): #Method to find Total
Sum = 0
for C in range(1, Number + 1):
Sum += C
return Sum
print(Tot(3)) #Function Calls
print(Tot(6))
6
21
- There should be a colon (:) at the end of the function definition line to indicate the start of the function block.
- Python's built-in function for generating sequences is
range()
, not Range(). - Python keywords like
return
should be in lowercase. - When calling a function in python, the arguments passed to the function should be enclosed inside parentheses () not square brackets [].
Find and write the output of the following python code :
def Call(P = 40, Q = 20):
P = P + Q
Q = P - Q
print(P, '@', Q)
return P
R = 200
S = 100
R = Call(R, S)
print(R, '@', S)
S = Call(S)
print(R, '@', S)
Answer
300 @ 200
300 @ 100
120 @ 100
300 @ 120
- Function Call is defined with two parameters
P
andQ
with default values 40 and 20 respectively. - Inside the function Call,
P
is reassigned to the sum of its original valueP
and the value ofQ
. Q
is reassigned to the difference between the new value ofP
and the original value ofQ
.- Prints the current values of
P
andQ
separated by @. - The function returns the final value of
P
. - Two variables
R
andS
are initialized with values 200 and 100 respectively. - The function Call is called with arguments
R
andS
, which are 200 and 100 respectively. Inside the function,P
becomes 200 + 100 = 300 andQ
becomes 300 - 100 = 200. So, 300 and 200 are printed. The function returns 300, which is then assigned toR
. Therefore,R
becomes 300. S = Call(S)
— The function Call is called with only one argumentS
, which is 100. Since the default value ofQ
is 20,P
becomes 100 + 20 = 120, andQ
becomes 120 - 20 = 100. So, 120 and 100 are printed. The function returns 120, which is then assigned toS
. Therefore,S
becomes 120.
Consider the following code and write the flow of execution for this. Line numbers have been given for your reference.
1. def power(b, p):
2. y = b ** p
3. return y
4.
5. def calcSquare(x):
6. a = power(x, 2)
7. return a
8.
9. n = 5
10. result = calcSquare(n)
11. print(result)
Answer
The flow of execution for the above program is as follows :
1 → 5 → 9 → 10 → 5 → 6 → 1 → 2 → 3 → 6 → 7 → 10 → 11
Line 1 is executed and determined that it is a function header, so entire function-body (i.e., lines 2 and 3) is ignored. Line 5 is executed and determined that it is a function header, so entire function-body (i.e., lines 6 and 7) is ignored. Lines 9 and 10 are executed, line 10 has a function call, so control jumps to function header (line 5) and then to first line of function-body, i.e., line 6, it has a function call , so control jumps to function header (line 1) and then to first line of function-body, i.e, line 2. Function returns after line 3 to line 6 and then returns after line 7 to line containing function call statement i.e, line 10 and then to line 11.
What will the following function return ?
def addEm(x, y, z):
print(x + y + z)
Answer
The function addEm
will return None
. The provided function addEm
takes three parameters x, y, and z, calculates their sum, and then prints the result. However, it doesn't explicitly return any value. In python, when a function doesn't have a return statement, it implicitly returns None
. Therefore, the function addEm will return None
.
What will the following function print when called ?
def addEm(x, y, z):
return x + y + z
print(x + y + z)
Answer
The function addEm prints nothing when called.
- The function
addEm(x, y, z)
takes three parameters x, y, and z. - It returns the sum of x, y, and z.
- Since the return statement is encountered first, the function exits immediately after returning the sum. The print statement after the return statement is never executed. Therefore, it prints nothing.
What will be the output of following program ?
num = 1
def myfunc():
return num
print(num)
print(myfunc())
print(num)
Answer
1
1
1
The code initializes a global variable num
with 1. myfunc
just returns this global variable. Hence, all the three print
statements print 1.
What will be the output of following program ?
num = 1
def myfunc():
num = 10
return num
print(num)
print(myfunc())
print(num)
Answer
1
10
1
num = 1
— This line assigns the value 1 to the global variablenum
.def myfunc()
— This line defines a function namedmyfunc
.print(num)
— This line prints the value of the global variablenum
, which is 1.print(myfunc())
— This line calls themyfunc
function. Insidemyfunc
function,num = 10
defines a local variablenum
and assigns it the value of10
which is then returned by the function. It is important to note that the value of global variablenum
is still 1 asnum
ofmyfunc
is local to it and different from global variablenum
.print(num)
— This line prints the value of the global variablenum
, which is still 1.
What will be the output of following program ?
num = 1
def myfunc():
global num
num = 10
return num
print(num)
print(myfunc())
print(num)
Answer
1
10
10
num = 1
— This line assigns the value 1 to the global variablenum
.def myfunc()
— This line defines a function namedmyfunc
.print(num)
— This line prints the value of the global variablenum
, which is 1.print(myfunc())
— This line calls themyfunc
function. Inside themyfunc
function, the value 10 is assigned to the global variablenum
. Because of the global keyword used earlier, this assignment modifies the value of the global variablenum
to 10. The function then returns 10.print(num)
— This line prints the value of the global variablenum
again, which is still 1.
What will be the output of following program ?
def display():
print("Hello", end='')
display()
print("there!")
Answer
Hellothere!
The function display prints "Hello" without a newline due to the end='' parameter. When called, it prints "Hello". Outside the function, "there!" is printed on the same line due to the absence of a newline.
Predict the output of the following code :
a = 10
y = 5
def myfunc():
y = a
a = 2
print("y =", y, "a =", a)
print("a + y =", a + y)
return a + y
print("y =", y, "a =", a)
print(myfunc())
print("y =", y, "a =", a)
Answer
The code raises an error when executed.
In the provided code, the global variables a
and y
are initialized to 10 and 5, respectively. Inside the myfunc
function, the line a = 2
suggests that a
is a local variable of myfunc
. But the line before it, y = a
is trying to assign the value of local variable a
to local variable y
even before local variable a
is defined. Therefore, this code raises an UnboundLocalError.
What is wrong with the following function definition ?
def addEm(x, y, z):
return x + y + z
print("the answer is", x + y + z)
Answer
In the above function definition, the line print("the answer is", x + y + z)
is placed after the return statement. In python, once a return statement is encountered, the function exits immediately, and any subsequent code in the function is not executed. Therefore, the print statement will never be executed.
Write a function namely fun that takes no parameters and always returns None.
Answer
def fun():
return
def fun():
return
r = fun()
print(r)
The function fun()
returns None
. When called, its return value is assigned to r
, which holds None
. Then print(r)
outputs None
.
Consider the code below and answer the questions that follow :
def multiply(number1, number2):
answer = number1 * number2
print(number1, 'times', number2, '=', answer)
return(answer)
output = multiply(5, 5)
(i) When the code above is executed, what prints out ?
(ii) What is variable output equal to after the code is executed ?
Answer
(i) When the code above is executed, it prints:
5 times 5 = 25
(ii) After the code is executed, the variable output
is equal to 25. This is because the function multiply returns the result of multiplying 5 and 5, which is then assigned to the variable output
.
Consider the code below and answer the questions that follow :
def multiply(number1, number2):
answer = number1 * number2
return(answer)
print(number1, 'times', number2, '=', answer)
output = multiply(5, 5)
(i) When the code above is executed, what gets printed ?
(ii) What is variable output equal to after the code is executed ?
Answer
(i) When the code above is executed, it will not print anything because the print statement after the return statement won't execute. Therefore, the function exits immediately after encountering the return statement.
(ii) After the code is executed, the variable output
is equal to 25. This is because the function multiply returns the result of multiplying 5 and 5, which is then assigned to the variable output
.
Find the errors in code given below :
def minus(total, decrement)
output = total - decrement
print(output)
return (output)
Answer
The errors in the code are:
def minus(total, decrement) # Error 1
output = total - decrement
print(output)
return (output)
- There should be a colon at the end of the function definition line.
The corrected code is given below:
def minus(total, decrement):
output = total - decrement
print(output)
return (output)
Find the errors in code given below :
define check()
N = input ('Enter N: ')
i = 3
answer = 1 + i ** 4 / N
Return answer
Answer
The errors in the code are:
define check() #Error 1
N = input ('Enter N: ') #Error 2
i = 3
answer = 1 + i ** 4 / N
Return answer #Error 3
- The function definition lacks a colon at the end.
- The 'input' function returns a string. To perform arithmetic operations with
N
, it needs to be converted to a numeric type, such as an integer or a float. - The return statement should be in lowercase.
The corrected code is given below:
def check():
N = int(input('Enter N:'))
i = 3
answer = 1 + i ** 4 / N
return answer
Find the errors in code given below :
def alpha (n, string = 'xyz', k = 10) :
return beta(string)
return n
def beta (string)
return string == str(n)
print(alpha("Valentine's Day"):)
print(beta(string = 'true'))
print(alpha(n = 5, "Good-bye"):)
Answer
The errors in the code are:
def alpha (n, string = 'xyz', k = 10) :
return beta(string)
return n #Error 1
def beta (string) #Error 2
return string == str(n)
print(alpha("Valentine's Day"):) #Error 3
print(beta(string = 'true')) #Error 4
print(alpha(n = 5, "Good-bye"):) #Error 5
- The second return statement in the
alpha
function (return n) is unreachable because the first return statementreturn beta(string)
exits the function. - The function definition lacks colon at the end. The variable
n
in thebeta
function is not defined. It's an argument toalpha
, but it's not passed tobeta
explicitly. To accessn
withinbeta
, we need to either pass it as an argument or define it as a global variable. - There should not be colon at the end in the function call.
- In the function call
beta(string = 'true')
, there should be argument for parametern
. - In the function call
alpha(n = 5, "Good-bye")
, the argument "Good-bye" lacks a keyword. It should be string = "Good-bye".
The corrected code is given below:
def alpha(n, string='xyz', k=10):
return beta(string, n)
def beta(string, n):
return string == str(n)
print(alpha("Valentine's Day"))
print(beta(string='true', n=10))
print(alpha(n=5, string="Good-bye"))
Draw the entire environment, including all user-defined variables at the time line 10 is being executed.
1. def sum(a, b, c, d):
2. result = 0
3. result = result + a + b + c + d
4. return result
5.
6. def length():
7. return 4
8.
9. def mean(a, b, c, d):
10. return float(sum(a, b, c, d))/length()
11.
12. print(sum(a, b, c, d), length(), mean(a, b, c, d))
Answer
The environment when the line 10 is being executed is shown below :
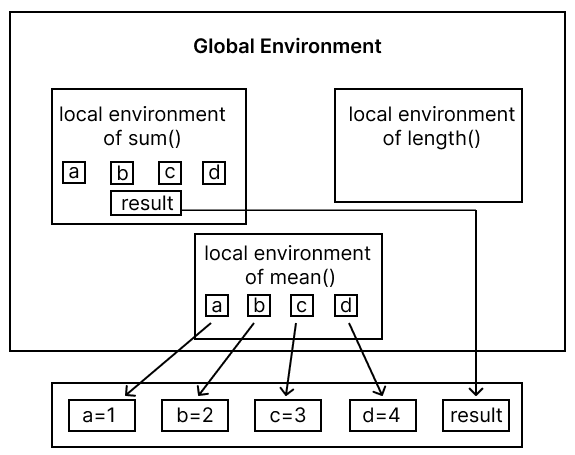
Draw flow of execution for the above program.
Answer
The flow of execution for the above program is as follows :
1 → 6 → 9 → 12 → 1 → 2 → 3 → 4 → 6 → 7 → 9 → 10 → 12
Line 1 is executed and determined that it is a function header, so entire function-body (i.e, lines 2, 3, 4) is ignored.Then line 6 is executed and determined that it is a function header, so entire function-body (i.e, line 7) is ignored, Line 9 is executed and determined that it is a function header, so entire function-body (i.e, line 10) is ignored. Then line 12 is executed and it has a function calls, so control jumps to the function header (line 1) and then first line of function-body, i.e, line 2, function returns after line 4 to function call line (line 12) and then control jumps to line 6, it is a function header and then first line of function-body, i.e., line 7, function returns after line 7 to function call line (line 12) and then control jumps to line 9, it is a function header and then first line of function-body, i.e., line 10, function returns after line 10 to function call line 12.
Find and write the output of the following python code :
a = 10
def call():
global a
a = 15
b = 20
print(a)
call()
Answer
15
a = 10
— This line assigns the value 10 to the global variablea
.def call()
— This line defines a function namedcall
.a = 15
— Inside the call function, this line assigns the value 15 to the global variablea
. Asglobal
keyword is used earlier, this assignment modifies the value of the global variablea
.b = 20
— Inside the call function, this line assigns the value 20 to a local variableb
.print(a)
— This line prints the value of the global variablea
, which is 15. This is because we've modified the global variablea
inside the call function.
In the following code, which variables are in the same scope ?
def func1():
a = 1
b = 2
def func2():
c = 3
d = 4
e = 5
Answer
In the code, variables a
and b
are in the same scope because they are defined within the same function func1()
. Similarly, variables c
and d
are in the same scope because they are defined within the same function func2()
. e
being a global variable is not in the same scope.
Write a program with a function that takes an integer and prints the number that follows after it. Call the function with these arguments :
4, 6, 8, 2 + 1, 4 - 3 * 2, -3 -2
Answer
1. def print_number(number):
2. next_number = number + 1
3. print("The number following", number, "is", next_number)
4. print_number(4)
5. print_number(6)
6. print_number(8)
7. print_number(2 + 1)
8. print_number(4 - 3 * 2)
9. print_number(- 3 - 2)
The print_number following 4 is 5
The print_number following 6 is 7
The print_number following 8 is 9
The print_number following 3 is 4
The print_number following -2 is -1
The print_number following -5 is -4
def print_number(number)
— This line defines a function namedprint_number
that takes one argumentnumber
.next_number = number + 1
— Inside theprint_number
function, this line calculates the next number after the input number by adding 1 to it and assigns the result to the variablenext_number
.print("The number following", number, "is", next_number)
— Inside theprint_number
function, this line prints a message stating the number and its following number.Then the
print_number
function is called multiple times with 4, 6, 8, 3 ,((4 - 3 * 2) = -2), ((-3-2) = -5) as arguments.
Write a program with non-void version of above function and then write flow of execution for both the programs.
Answer
The non-void version of above code is as shown below :
1. def print_number(number):
2. next_number = number + 1
3. return next_number
4. print(print_number(4))
5. print(print_number(6))
6. print(print_number(8))
7. print(print_number(2 + 1))
8. print(print_number(4 - 3 * 2))
9. print(print_number(-3 - 2))
5
7
9
4
-1
-4
def print_number(number)
— This line defines a function namedprint_number
that takes one argumentnumber
.next_number = number + 1
— Inside theprint_number
function, this line calculates the next number after the input number by adding 1 to it and assigns the result to the variablenext_number
.return next_number
— Inside theprint_number
function, this line returnsnext_number
.Then the
print_number
function is called multiple times with 4, 6, 8, 3 ,((4 - 3 * 2) = -2), ((-3-2) = -5) as arguments.
The flow of execution for the above program with non-void version is as follows :
1 → 4 → 1 → 2 → 3 → 4 → 5 → 1 → 2 → 3 → 5 → 6 → 1 → 2 → 3 → 6 → 7 → 1 → 2 → 3 → 7 → 8 → 1 → 2 → 3 → 8 → 9 → 1 → 2 → 3 → 9
Line 1 is executed and determined that it is a function header, so entire function-body (i.e., line 2 and 3) is ignored. Then line 4 is executed and it has function call, so control jumps to the function header (line 1) and then to first line of function-body, i.e., line 2, function returns after line 3 to line containing function call statement i.e., line 4. The next lines 5, 6, 7, 8, 9 have function calls so they repeat the above steps.
The flow of execution for the void version program is as follows :
1 → 4 → 1 → 2 → 3 → 4 → 5 → 1 → 2 → 3 → 5 → 6 → 1 → 2 → 3 → 6 → 7 → 1 → 2 → 3 → 7 → 8 → 1 → 2 → 3 → 8 → 9 → 1 → 2 → 3 → 9
Line 1 is executed and determined that it is a function header, so entire function-body (i.e., line 2 and 3) is ignored. Then line 4 is executed and it has function call, so control jumps to the function header (line 1) and then to first line of function-body, i.e., line 2, function returns after line 3 to line containing function call statement i.e., line 4. The next lines 5, 6, 7, 8, 9 have function calls so they repeat the above steps.
What is the output of following code fragments ?
def increment(n):
n.append([4])
return n
L = [1, 2, 3]
M = increment(L)
print(L, M)
Answer
[1, 2, 3, [4]] [1, 2, 3, [4]]
In the code, the function increment
appends [4] to list n
, modifying it in place. When L = [1, 2, 3]
, calling increment(L)
changes L to [1, 2, 3, [4]]. Variable M
receives the same modified list [1, 2, 3, [4]], representing L. Thus, printing L and M results in [1, 2, 3, [4]], confirming they reference the same list. Therefore, modifications made to list inside a function affect the original list passed to the function.
What is the output of following code fragments ?
def increment(n):
n.append([49])
return n[0], n[1], n[2], n[3]
L = [23, 35, 47]
m1, m2, m3, m4 = increment(L)
print(L)
print(m1, m2, m3, m4)
print(L[3] == m4)
Answer
[23, 35, 47, [49]]
23 35 47 [49]
True
The function increment
appends [49] to list n
and returns its first four elements individually. When L = [23, 35, 47]
, calling increment(L)
modifies L to [23, 35, 47, [49]]. Variables m1, m2, m3, and m4
are assigned the same list [23, 35, 47, [49]], representing the original list L. Thus, printing L and m1, m2, m3, m4 yields [23, 35, 47, [49]]. The expression L[3] == m4 evaluates to True, indicating that the fourth element of L is the same as m4.
What will be the output of the following Python code ?
V = 25
def Fun(Ch):
V = 50
print(V, end = Ch)
V *= 2
print(V, end = Ch)
print(V, end = "*")
Fun("!")
print(V)
- 25*50!100!25
- 50*100!100!100
- 25*50!100!100
- Error
Answer
25*50!100!25
V = 25
— initializes global variable V to 25.print(V, end = "*")
— Prints the global variableV
(25) followed by an asterisk without a newline.Fun("!")
— Calls the functionFun
with the argument "!".- Inside function
Fun
,V = 50
initializes a local variableV
within the function with the value 50. This variable is different from the global variableV
that has the value 25. print(V, end = Ch)
— Prints the local variableV
(50) followed by the character passed as an argument to the function, which is "!".V *= 2
— Multiplies the local variableV
(50) by 2, making it 100.print(V, end = Ch)
— Prints the updated local variable V (100) followed by the character "!".- The function
Fun
returns and the lineprint(V)
is executed. It prints the global variableV
without any newline character. The global variableV
remains unchanged (25).
Write a function that takes amount-in-dollars and dollar-to-rupee conversion price; it then returns the amount converted to rupees. Create the function in both void and non-void forms.
def convert_dollars_to_rupees(amount_in_dollars, conversion_rate):
amount_in_rupees = amount_in_dollars * conversion_rate
return amount_in_rupees
def convert_dollars_to_rupees_void(amount_in_dollars, conversion_rate):
amount_in_rupees = amount_in_dollars * conversion_rate
print("Amount in rupees:", amount_in_rupees)
amount = float(input("Enter amount in dollars "))
conversion_rate = float(input("Enter conversion rate "))
# Non-void function call
converted_amount = convert_dollars_to_rupees(amount, conversion_rate)
print("Converted amount (non-void function):", converted_amount)
# Void function call
convert_dollars_to_rupees_void(amount, conversion_rate)
Enter amount in dollars 50
Enter conversion rate 74.5
Converted amount (non-void function): 3725.0
Amount in rupees: 3725.0
Enter amount in dollars 100
Enter conversion rate 75
Converted amount (non-void function): 7500.0
Amount in rupees: 7500.0
Write a function to calculate volume of a box with appropriate default values for its parameters. Your function should have the following input parameters :
(a) length of box ;
(b) width of box ;
(c) height of box.
Test it by writing complete program to invoke it.
def calculate_volume(length = 5, width = 3, height = 2):
return length * width * height
default_volume = calculate_volume()
print("Volume of the box with default values:", default_volume)
v = calculate_volume(10, 7, 15)
print("Volume of the box with default values:", v)
a = calculate_volume(length = 23, height = 6)
print("Volume of the box with default values:", a)
b = calculate_volume(width = 19)
print("Volume of the box with default values:", b)
Volume of the box with default values: 30
Volume of the box with default values: 1050
Volume of the box with default values: 414
Volume of the box with default values: 190
Write a program to have following functions :
(i) a function that takes a number as argument and calculates cube for it. The function does not return a value. If there is no value passed to the function in function call, the function should calculate cube of 2.
(ii) a function that takes two char arguments and returns True if both the arguments are equal otherwise False.
Test both these functions by giving appropriate function call statements.
# Function to calculate cube of a number
def calculate_cube(number = 2):
cube = number ** 3
print("Cube of", number, "is", cube)
# Function to check if two characters are equal
def check_equal_chars(char1, char2):
return char1 == char2
calculate_cube(3)
calculate_cube()
char1 = 'a'
char2 = 'b'
print("Characters are equal:", check_equal_chars(char1, char1))
print("Characters are equal:", check_equal_chars(char1, char2))
Cube of 3 is 27
Cube of 2 is 8
Characters are equal: True
Characters are equal: False
Write a function that receives two numbers and generates a random number from that range. Using this function, the main program should be able to print three numbers randomly.
import random
def generate_random_number(num1, num2):
low = min(num1, num2)
high = max(num1, num2)
return random.randint(low, high)
num1 = int(input("Enter the first number: "))
num2 = int(input("Enter the second number: "))
for i in range(3):
random_num = generate_random_number(num1, num2)
print("Random number between", num1, "and", num2, ":", random_num)
Enter the first number: 2
Enter the second number: 78
Random number between 2 and 78 : 77
Random number between 2 and 78 : 43
Random number between 2 and 78 : 52
Enter the first number: 100
Enter the second number: 599
Random number between 100 and 599 : 187
Random number between 100 and 599 : 404
Random number between 100 and 599 : 451
Write a function that receives two string arguments and checks whether they are same-length strings (returns True in this case otherwise False).
def same_length_strings(str1, str2):
return len(str1) == len(str2)
s1 = "hello"
s2 = "world"
s3 = "python"
print(same_length_strings(s1, s2))
print(same_length_strings(s1, s3))
True
False
Write a function namely nthRoot( ) that receives two parameters x and n and returns nth root of x i.e., x^(1/n).
The default value of n is 2.
def nthRoot(x, n = 2):
return x ** (1/n)
x = int(input("Enter the value of x:"))
n = int(input("Enter the value of n:"))
result = nthRoot(x, n)
print("The", n, "th root of", x, "is:", result)
default_result = nthRoot(x)
print("The square root of", x, "is:", default_result)
Enter the value of x:36
Enter the value of n:6
The 6 th root of 36 is: 1.8171205928321397
The square root of 36 is: 6.0
Write a function that takes a number n and then returns a randomly generated number having exactly n digits (not starting with zero) e.g., if n is 2 then function can randomly return a number 10-99 but 07, 02 etc. are not valid two digit numbers.
import random
def generate_number(n):
lower_bound = 10 ** (n - 1)
upper_bound = (10 ** n) - 1
return random.randint(lower_bound, upper_bound)
n = int(input("Enter the value of n:"))
random_number = generate_number(n)
print("Random number:", random_number)
Enter the value of n:2
Random number: 10
Enter the value of n:2
Random number: 50
Enter the value of n:3
Random number: 899
Enter the value of n:4
Random number: 1204
Write a function that takes two numbers and returns the number that has minimum one's digit.
[For example, if numbers passed are 491 and 278, then the function will return 491 because it has got minimum one's digit out of two given numbers (491's 1 is < 278's 8)].
def min_ones_digit(num1, num2):
ones_digit_num1 = num1 % 10
ones_digit_num2 = num2 % 10
if ones_digit_num1 < ones_digit_num2:
return num1
else:
return num2
num1 = int(input("Enter first number:"))
num2 = int(input("Enter second number:"))
result = min_ones_digit(num1, num2)
print("Number with minimum one's digit:", result)
Enter first number:491
Enter second number:278
Number with minimum one's digit: 491
Enter first number:543
Enter second number:765
Number with minimum one's digit: 543
Write a program that generates a series using a function which takes first and last values of the series and then generates four terms that are equidistant e.g., if two numbers passed are 1 and 7 then function returns 1 3 5 7.
def generate_series(first, last):
step = (last - first) // 3
series = [first, first + step, first + 2 * step, last]
return series
first_value = int(input("Enter first value:"))
last_value = int(input("Enter last value:"))
result_series = generate_series(first_value, last_value)
print("Generated Series:", result_series)
Enter first value:1
Enter last value:7
Generated Series: [1, 3, 5, 7]
Enter first value:10
Enter last value:25
Generated Series: [10, 15, 20, 25]