Multiple Choice Questions
Question 1
Which of the following is a primitive data type?
- int
- float
- char
- All of these
Answer
All of these
Reason — int, float, char, all three are primitive data types in Java.
Question 2
Which of the following is a composite data type?
- int
- float
- char
- String
Answer
String
Reason — String is a composite data type.
Question 3
The return type of the isLowerCase() method is ............... .
- int
- boolean
- char
- String
Answer
boolean
Reason — The return type of isLowerCase() method is boolean as it returns true if the character argument is in lower case else it returns false.
Question 4
The return type of the toLowerCase() method is ............... .
- int
- boolean
- char
- String
Answer
char
Reason — The return type of the toLowerCase() method is char as it converts the given character argument into lower case.
Question 5
The value returned by Integer.parseInt("-321") is ............... .
- -321
- 321
- 321.0
- "321"
Answer
-321
Reason — parseInt() converts the given String argument into an integer value if the given string is a combination of numbers and decimal. The String can have a '-' sign in the beginning.
Question 6
Name the method that can convert a string into its integer equivalent.
- Integer.parseInteger()
- Integer.getInt()
- Integer.parseInt()
- Integer.readInt()
Answer
Integer.parseInt()
Reason — Integer.parseInt() method parses the String argument as a signed integer.
Question 7
What will be the result when the following statement is executed?int count = new Integer(12);
- Variable count will be initialised with value 12.
- Variable count will be initialised with default value of int, i.e., zero (0).
- An array count will be initialised with 12 elements, all having a default value of zero (0).
- Value of count will be unknown as no value has been assigned yet.
Answer
Variable count will be initialised with value 12.
Reason — The given statement initialises the variable count with the integer value 12.
Question 8
In which package is the wrapper class Integer available?
- java.io
- java.util
- java.awt
- java.lang
Answer
java.lang
Reason — All the wrapper classes are available in java.lang package.
Assignment Questions
Question 1
What are the library classes in Java? What is their use?
Answer
Library classes are the pre-written classes which are a part of the Java system. For example, the String and Scanner class.
Java environment has a huge library of library classes that contain pre-defined methods to simplify the job of a programmer. These methods support input/output operations, String handling and help in development of network and graphical user interface.
Question 2(i)
Define Primitive data type
Answer
Primitive data types are pre-defined by the language and form the basic building blocks of representing data. They store a single value of a specific declared type at a time. The eight built-in primitive data types in Java are byte, short, int, long, float, double, char, boolean.
Question 2(ii)
Define Composite data type
Answer
A composite data type is a data type which can be constructed in a program using the programming language's primitive data types. It is a collection of primitive data types. Examples of composite data types are String and Array.
Question 2(iii)
Define User-defined data type
Answer
The data type defined by the user to perform some specific task is known as a user-defined data type. For example, the classes created by the user are user defined data types.
Question 3
Why is a class called a composite data type? Explain.
Answer
In a class, one can assemble items of different data types to create a composite data type. The class can also be considered as a new data type created by the user, that has its own functionality. The classes allow these user-defined types to be used in programs. Hence, a class is called a composite data type.
Question 4
What is a wrapper class? Name three wrapper classes in Java.
Answer
A wrapper class allows us to convert a primitive data type into an object type. Each of Java's eight primitive data types has a wrapper class dedicated to it. These are known as wrapper classes because they wrap the primitive data type into an object of that class.
The three wrapper classes are Integer, Float and Double.
Question 5
Explain the terms, Autoboxing and Auto-unboxing in Java.
Answer
The automatic conversion of primitive data type into an object of its equivalent wrapper class is known as Autoboxing. For example, the below statement shows the conversion of an int to an Integer.
Integer a = 20;
Here, the int value 20
is autoboxed into the wrapper class Integer variable myinteger
.
Auto-unboxing is the reverse process of Autoboxing. It is the automatic conversion of a wrapper class object into its corresponding primitive type. For example,
Integer myinteger = 20;
int myint = myinteger;
Here, the object myInteger
is automatically unboxed into primitive type int variable myint
when the assignment takes place.
Question 6
How do you convert a numeric string into a double value?
Answer
The wrapper class Double has a method parseDouble() which is used to parse a numeric string into a double value.
Syntax:
Double.parseDouble(String s);
Example:
String str = "437246.643";
double d = Double.parseDouble(str);
Question 7
Describe wrapper class methods available in Java to parse string values to their numeric equivalents.
Answer
The wrapper class methods available in Java to parse string values to their numeric equivalents are described below:
parseInt(string) — It is a part of wrapper class Integer. It parses the string argument as a signed integer. The characters in the string must be digits or digits separated with a decimal. The first character may be a minus sign (-) to indicate a negative value or a plus sign (+) to indicate a positive value.
Syntax:int parseInt(String s)
parseLong(string) — It is a part of wrapper class Long. It parses the string argument as a signed long. The characters in the string must be digits or digits separated with a decimal. The first character may be a minus sign (-) to indicate a negative value or a plus sign (+) to indicate a positive value.
Syntax:long parseLong(String s)
parseFloat(string) — It is a part of wrapper class Float. It returns a float value represented by the specified string.
Syntax:float parseFloat(String s)
parseDouble(string) — It is a part of wrapper class Double. It returns a double value represented by the specified string.
Syntax:double parseDouble(String s)
Question 8
How can you check if a given character is a digit, a letter or a space?
Answer
We can check if a given character is a digit, a letter or a space by using the following methods of Character class:
isDigit(char) — It returns true if the specified character is a digit; returns false otherwise.
Syntax:boolean isDigit(char ch)
isLetter(char) — It returns true if the specified character is a letter; returns false otherwise.
Syntax:boolean isLetter(char ch)
isLetterOrDigit(char) — It returns true if the specified character is a letter or a digit; returns false otherwise.
Syntax:boolean isLetterOrDigit(char ch)
isWhitespace(char) — It returns true if the specified character is whitespace; returns false otherwise.
Syntax:boolean isWhitespace(char ch)
Question 9(i)
Distinguish between isLowerCase() and toLowerCase()
Answer
isLowerCase() | toLowerCase() |
---|---|
isLowerCase( ) function checks if a given character is in lower case or not. | toLowerCase( ) function converts a given character to lower case. |
Its return type is boolean. | Its return type is char. |
Question 9(ii)
Distinguish between isUpperCase() and toUpperCase()
Answer
isUpperCase( ) | toUpperCase( ) |
---|---|
isUpperCase( ) function checks if a given character is in upper case or not. | toUpperCase( ) function converts a given character to upper case. |
Its return type is boolean. | Its return type is char. |
Question 9(iii)
Distinguish between isDigit() and isLetter()
Answer
isDigit() | isLetter() |
---|---|
isDigit() method returns true if the specified character is a digit; returns false otherwise. | isLetter() method returns true if the specified character is a letter; returns false otherwise. |
Question 9(iv)
Distinguish between parseFloat() and parseDouble()
Answer
parseFloat() | parseDouble() |
---|---|
parseFloat() method returns a float value represented by the specified string. | parseDouble() method returns a double value represented by the specified string. |
Question 10
Define a class (using the Scanner class) to generate a pattern of a word in the form of a triangle or in the form of an inverted triangle, depending upon user's choice.
Sample Input:
Enter a word: CLASS
Enter your choice: 1
Sample Output:
C
CL
CLA
CLAS
CLASS
Enter your choice: 2
Sample Output:
CLASS
CLAS
CLA
CL
C
import java.util.Scanner;
public class KboatTriangleMenu
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a word: ");
String word = in.nextLine();
System.out.println("Type 1 for a triangle");
System.out.println("Type 2 for an inverted triangle");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
int len = word.length();
switch (choice) {
case 1:
for(int i = 0; i < len; i++) {
for(int j = 0; j <= i; j++) {
System.out.print(word.charAt(j));
}
System.out.println();
}
break;
case 2:
for (int i = len - 1; i >= 0; i--) {
for (int j = 0; j <= i; j++) {
char ch = word.charAt(j);
System.out.print(ch);
}
System.out.println();
}
break;
default:
System.out.println("Incorrect choice");
break;
}
}
}
Output
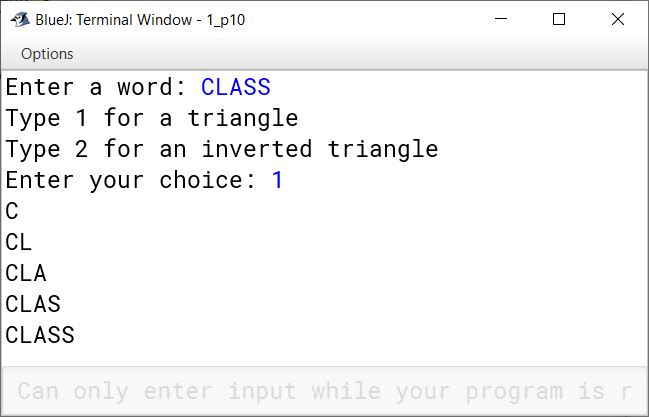
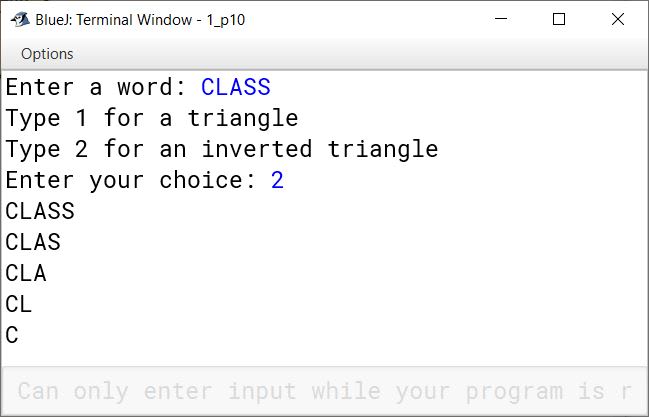
Question 11
Define a class called mobike with the following description:
Instance variables/Data members:
int bno - to store the bike's number
int phno - to store the phone number of the customer
String name - to store the name of the customer
int days - to store the number of days the bike is taken on rent
int charge - to calculate and store the rental charge
Member Methods:
void input() - to input and store the details of the customer
void compute() - to compute the rental charge
The rent for a mobike is charged on the following basis:
First five days Rs 500 per day;
Next five days Rs 400 per day;
Rest of the days Rs 200 per day.
void display () - to display the details in the following format:
Bike No. Phone No. No. of days Charge
import java.util.Scanner;
public class Mobike
{
private int bno;
private int phno;
private int days;
private int charge;
private String name;
public void input() {
Scanner in = new Scanner(System.in);
System.out.print("Enter Customer Name: ");
name = in.nextLine();
System.out.print("Enter Customer Phone Number: ");
phno = in.nextInt();
System.out.print("Enter Bike Number: ");
bno = in.nextInt();
System.out.print("Enter Number of Days: ");
days = in.nextInt();
}
public void compute() {
if (days <= 5)
charge = days * 500;
else if (days <= 10)
charge = (5 * 500) + ((days - 5) * 400);
else
charge = (5 * 500) + (5 * 400) + ((days - 10) * 200);
}
public void display() {
System.out.println("Bike No.\tPhone No.\tNo. of days\tCharge");
System.out.println(bno + "\t" + phno + "\t" + days + "\t" + charge);
}
public static void main(String args[]) {
Mobike obj = new Mobike();
obj.input();
obj.compute();
obj.display();
}
}
Output
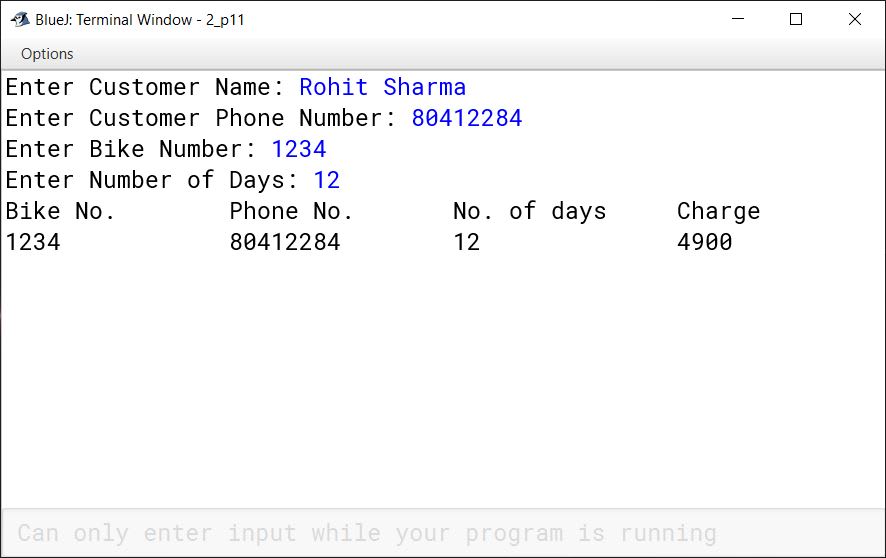