The Boolean group has only one primitive data type boolean which is used to store the Boolean values true or false. The size of boolean is 8 bits but only one bit is used to actually store the value. Since boolean values can only be one of the two types true or false, one bit is enough to store them. As we have seen previously, boolean
is the type required by conditional expressions that govern the if
and for
statements. Its time to look at a BlueJ program to see boolean types in action.
public class BooleanType
{
public void demoBoolean() {
//Declare a boolean variable
boolean bTest;
bTest = false;
System.out.println("The value of bTest is " + bTest);
bTest = true;
System.out.println("The value of bTest is " + bTest);
if (bTest)
System.out.println("A boolean value itself is " +
"sufficient as condition of if statement");
bTest = false;
if (bTest) //condition is false, println is not executed
System.out.println("This will not be printed");
}
}
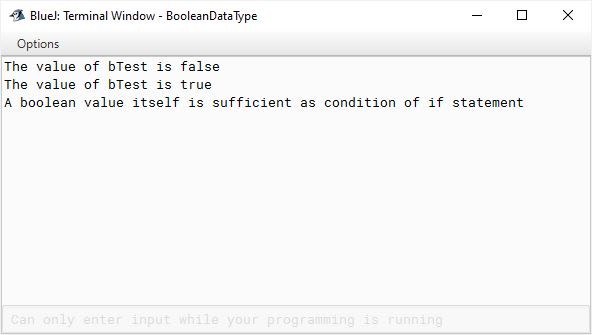
We declare a variable of boolean type like this boolean bTest;
. Next, we assign the boolean literal false
to bTest
and print its value. Notice in the output the value of bTest is printed as the word false.
Next we change the value of bTest
to true
and again print its value. As expected, its value gets printed as the word true.
Next bTest
is used as the condition of if
statement. As bTest
is a boolean variable, it is itself sufficient as the condition of if
statement. No need to write it as if (bTest == true)
, that will work too but it is kind of redundant.
Next we set bTest
to false
and use it in the if
statement. As bTest
is false
, the println
statement following it doesn’t get executed hence we don't see it in the output.