So far, we have been using literals for initializing the variables. But that’s not the only way to initialize the variables. We can also use dynamic expressions for initialization. Let me show you what I mean by dynamic initialization through a BlueJ program.
This program computes the distance between 2 points specified by their coordinates (x1, y1) and (x2, y2)
public class DynamicInitializationDemo
{
public void computeDistance() {
//Initialization using literals
int x1 = 10, y1 = 20, x2 = 30, y2 = 40;
//Initialization using dynamic expression involving other variables
int xDist = x2 - x1;
int yDist = y2 - y1;
//Initialization using dynamic expression involving call to method
double computedDistance = Math.sqrt((xDist * xDist) + (yDist * yDist));
System.out.println(
"Distance between ("
+ x1
+ ", "
+ y1
+ ") and ("
+ x2
+ ", "
+ y2
+ ") is "
+ computedDistance
);
}
}
Here is the output of the program:
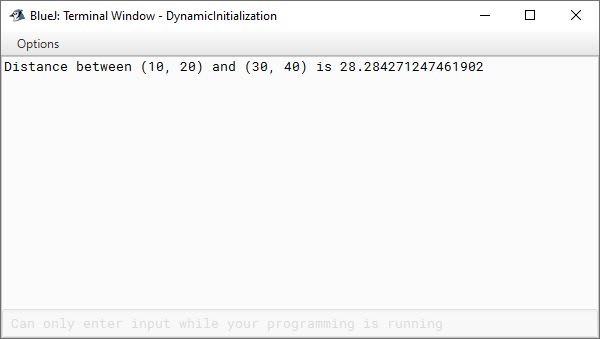
Let's try to understand the program. In the first set of lines:
//Initialization using literals
int x1 = 10, y1 = 20, x2 = 30, y2 = 40;
we are initialization the coordinates of the points in the regular way using integer literals 10
, 20
, 30
, 40
.
//Initialization using dynamic expression involving other variables
int xDist = x2 - x1;
int yDist = y2 - y1;
After that, we are dynamically initializing xDist and yDist by storing the difference between x2 and x1 in xDist and y2 & y1 in yDist.
//Initialization using dynamic expression involving call to method
double computedDistance = Math.sqrt((xDist * xDist) + (yDist * yDist));
Then we are dynamically initializing computedDistance
by storing the value returned by Math.sqrt
method into it. Math.sqrt
is one of Java’s built-in methods. It returns the square root of its argument. Finally we print the computed distance between the two points on the screen.
As you have seen in this program, we can use literals, other variables and calls to other methods in the initialization expression.