In this lesson, we will look at a Java program which checks if a number is a Buzz number or not. A number is a Buzz number if it ends with 7 or is divisible by 7. 63 is a Buzz number as it is divisible by 7. 747 is also a Buzz number as it ends with 7. 83 is not a Buzz number as it is neither divisible by 7 nor it ends in 7.
Here is the Buzz number Java program:
import java.util.Scanner;
public class KboatBuzzNumber
{
public void buzzNumberTest() {
Scanner in = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = in.nextInt();
if (number % 10 == 7 || number % 7 == 0)
System.out.println(number + " is a Buzz number.");
else
System.out.println(number + " is a not Buzz number.");
}
}
First we take the number to be checked as input from the user with the help of Scanner class and store it in int variable number
. Next, the if condition checks if the number is a Buzz number or not. First part of the if condition — number % 10 == 7
checks if last digit of the number is 7. We discussed this in extracting digits example that when we divide any number by 10, the remainder is the digit in units place. We check if it is equal to 7.
In the next part — number % 7 == 0
we check if the number is divisible by 7. Both checks are joined together with logical OR operator — ||
so if any one of them is true we print that the number is a Buzz number otherwise it is not.
Fairly simple and straightforward, isn’t it?
Below is the output of Buzz number program for 63, 747 and 83 as inputs:
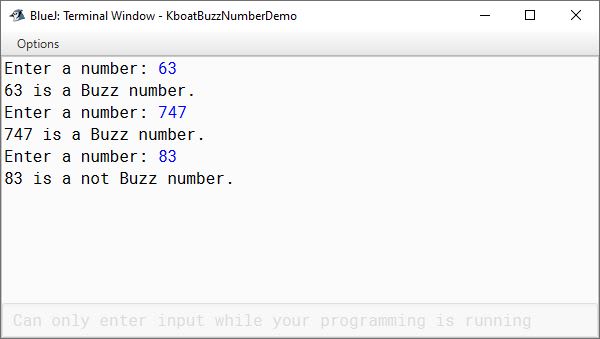