The two actions here that we can move to their own methods are calculating the area and perimeter of the rectangles.
Method to Calculate Area
Let’s start with area first.
public static int computeArea(int l, int w)
{
int a = l * w;
return a;
}
This is how the method to compute area of the rectangle looks like. We have used public and static as access-specifier and modifier, respectively. The return type is int as we are assuming the sides of the rectangle to be integers between 1 to 100, so multiplying them will also result in an integer. The name of this method is computeArea.
The method has two int variables l
and w
in its parameter list as its formal arguments. The first formal argument l
is for the length of the rectangle and the second one w
is for width.
In the method body, we have written the statement to compute the area of the rectangle. We are returning this area as the output of the method using the return statement.
The below picture shows the different elements of method definition for computeArea method:
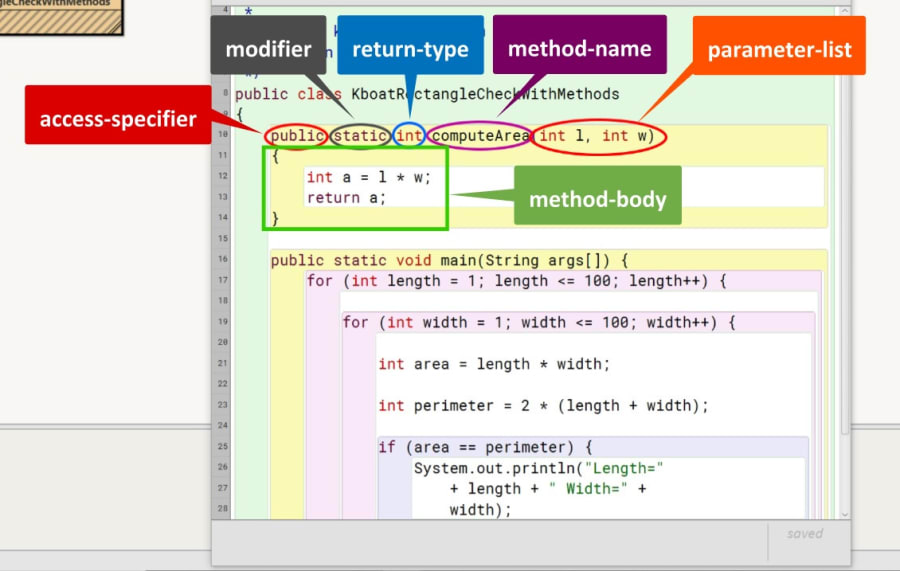
Method to Calculate Perimeter
Similarly, we can write the method for calculating the perimeter like this:
public static int computePerimeter(int l, int w)
{
int p = 2 * (l + w);
return p;
}
Calling the Methods
There are two steps involved in using methods. First step is defining the methods and we have already completed that. Defining the method means that our method is created. Defining the method doesn’t mean that the statements in the method's body will start executing right away. To use our method, that is, to execute the statements of our method and get an output from it, we need to call the methods.
Below is the complete program with method definitions and calls:
public class KboatRectangleCheckWithMethods
{
/*
* Method to calculate
* area of the rectangle
*/
public static int computeArea (int l, int w)
{
int a = l * w;
return a;
}
/*
* Method to calculate
* perimeter of the rectangle
*/
public static int computePerimeter(int l, int w)
{
int p = 2 * (l + w);
return p;
}
public static void main(String args[]) {
for (int length = 1; length <= 100; length++) {
for (int width = 1; width <= 100; width++) {
/*
* Method call for
* calculating area
*/
int area = computeArea (length, width);
/*
* Method call for
* calculating perimeter
*/
int perimeter = computePerimeter(length, width);
if (area == perimeter)
System.out.println("Length=" + length + "\t"
+ "Width=" + width);
}
}
}
}
When we are calling the method, we need to supply it the inputs that we mentioned in the parameter list while defining the method. Similar to the parameter list of the method definition, here also the inputs are enclosed within parentheses and separated by commas. These arguments or parameters that we use in the method-call are termed as actual parameters or actual arguments.
So, the formal arguments of method computeArea are l
and w
that are mentioned in the method definition while the actual arguments for the method call of computeArea are length
and width
. Formal arguments are used in method definition whereas actual arguments are used per method call. One important thing to note here is that unlike the method definition, when we are calling the method, we don’t mention the data types of the inputs.
Notice the assignment part in the call to computeArea method — int area = computeArea(length, width);
. computeArea method computes the area and returns it back as its output by using the return statement. That value of area returned by computeArea method gets assigned to the int variable area
. Similarly, the value of perimeter — p
, returned by computePerimeter method is assigned to int variable perimeter
.
So that’s how we define, call and execute methods. You might wonder at this point that the program we wrote initially without methods was also working fine. So, what is the benefit of using methods in it? Is it just some fancy way of coding or are there any real advantages also in using methods? Turns out that methods offer many advantages in programming. We will look at it in the next lesson.