Multiple Choice Questions
Question 1
In a Python program, a control structure:
- directs the order of execution of the statements in the program ✓
- dictates what happens before the program starts and after it terminates
- defines program-specific data structures
- manages the input and output of control characters
Question 2
An empty/null statement in Python is
- go
- pass ✓
- over
- ;
Question 3
The order of statement execution in the form of top to bottom, is known as .......... construct.
- selection
- repetition
- sequence ✓
- flow
Question 4
The .......... construct allows to choose statements to be executed, depending upon the result of a condition.
- selection ✓
- repetition
- sequence
- flow
Question 5
The .......... construct repeats a set of statements a specified number of times or as long as a condition is true.
- selection
- repetition ✓
- sequence
- flow
Question 6
Which of the following statements will make a selection construct?
- if ✓
- if-else ✓
- for
- while
Question 7
Which of the following statements will make a repetition construct?
- if
- if-else
- for ✓
- while ✓
Question 8
In Python, which of the following will create a block in a compound statement ?
- colon
- statements indented at a lower, same level ✓
- indentation in any form
- { }
Question 9
What signifies the end of a statement block or suite in Python ?
- A comment
- }
- end
- A line that is indented less than the previous line ✓
Question 10
Which one of the following if statements will not execute successfully ?
- if (1, 2) :
print('foo') - if (1, 2)
print('foo') ✓ - if (1, 2) :
print('foo') ✓ - if (1) :
print( 'foo' )
Question 11
What does the following Python program display ?
x = 3
if x == 0:
print ("Am I here?", end = ' ')
elif x == 3:
print("Or here?", end = ' ')
else :
pass
print ("Or over here?")
- Am I here?
- Or here?
- Am I here? Or here?
- Or here? Or over here? ✓
- Am I here? Or over here?
Question 12
If the user inputs : 2<ENTER>, what does the following code snippet print?
x = float(input())
if(x==1):
print("Yes")
elif (x >= 2):
print("Maybe")
else:
print ("No")
- Yes
- No
- Maybe ✓
- Nothing is printed
- Error
Question 13
Consider the following code segment:
a = int(input("Enter an integer: "))
b = int(input("Enter an integer: "))
if a <= 0:
b = b +1
else:
a = a + 1
if a > 0 and b > 0:
print ("W")
elif a > 0:
print("X")
if b > 0:
print("Y")
else:
print("Z")
What letters will be printed if the user enters 0 for a and 0 for b ?
- Only W
- Only X
- Only Y ✓
- W and X
- W, X and Y
Question 14
Consider the following code segment:
a = int(input("Enter an integer: "))
b = int(input("Enter an integer: "))
if a <= 0:
b = b +1
else:
a = a + 1
if a > 0 and b > 0:
print ("W")
elif a > 0:
print("X")
if b > 0:
print("Y")
else:
print("Z")
What letters will be printed if the user enters 1 for a and 1 for b ?
- W and X
- W and Y ✓
- X and Y
- X and Z
- W, X and Y
Question 15
Consider the following code segment:
a = int(input("Enter an integer: "))
b = int(input("Enter an integer: "))
if a <= 0:
b = b +1
else:
a = a + 1
if a > 0 and b > 0:
print ("W")
elif a > 0:
print("X")
if b > 0:
print("Y")
else:
print("Z")
What letters will be printed if the user enters 1 for a and -1 for b?
- W and X
- X and Y
- Y and Z
- X and Z ✓
- W and Z
Question 16
Consider the following code segment:
a = int(input("Enter an integer: "))
b = int(input("Enter an integer: "))
if a <= 0:
b = b +1
else:
a = a + 1
if a > 0 and b > 0:
print ("W")
elif a > 0:
print("X")
if b > 0:
print("Y")
else:
print("Z")
What letters will be printed if the user enters 1 for a and 0 for b ?
- W and X
- X and Y
- Y and Z
- X and Z ✓
- W and Z
Question 17
Consider the following code segment:
a = int(input("Enter an integer: "))
b = int(input("Enter an integer: "))
if a <= 0:
b = b +1
else:
a = a + 1
if a > 0 and b > 0:
print ("W")
elif a > 0:
print("X")
if b > 0:
print("Y")
else:
print("Z")
What letters will be printed if the user enters -1 for a and -1 for b?
- Only W
- Only X
- Only Y
- Only Z ✓
- No letters are printed
Question 18
What values are generated when the function range(6, 0, -2) is executed ?
- [4, 2]
- [4, 2, 0]
- [6, 4, 2] ✓
- [6, 4, 2, 0]
- [6, 4, 2, 0, -2]
Question 19
Which of the following is not a valid loop in Python ?
- for
- while
- do-while ✓
- if-else ✓
Question 20
Which of the following statement(s) will terminate the whole loop and proceed to the statement following the loop ?
- pass
- break ✓
- continue
- goto
Question 21
Which of the following statement(s) will terminate only the current pass of the loop and proceed with the next iteration of the loop?
- pass
- break
- continue ✓
- goto
Question 22
Function range(3) is equivalent to :
- range(1, 3)
- range(0, 3) ✓
- range(0, 3, 1) ✓
- range(1, 3, 0)
Question 23
Function range(3) will yield an iteratable sequence like
- [0, 1, 2] ✓
- [0, 1, 2, 3]
- [1, 2, 3]
- [0, 2]
Question 24
Function range(0, 5, 2) will yield on iterable sequence like
- [0, 2, 4] ✓
- [1, 3, 5]
- [0, 1, 2, 5]
- [0, 5, 2]
Question 25
Function range(10, 5, -2) will yield an iterable sequence like
- [10, 8, 6] ✓
- [9, 7, 5]
- [6, 8, 10]
- [5, 7, 9]
Question 26
Function range(10, 5, 2) will yield an iterable sequence like
- [] ✓
- [10, 8, 6]
- [2, 5, 8]
- [8, 5, 2]
Question 27
Consider the loop given below :
for i in range(-5) :
print(i)
How many times will this loop run?
- 5
- 0 ✓
- infinite
- Error
Question 28
Consider the loop given below :
for i in range(10, 5, -3) :
print(i)
How many times will this loop run?
- 3
- 2 ✓
- 1
- Infinite
Question 29
Consider the loop given below :
for i in range(3) :
pass
What will be the final value of i after this loop ?
- 0
- 1
- 2 ✓
- 3
Question 30
Consider the loop given below :
for i in range(7, 4, -2) :
break
What will be the final value of i after this loop ?
- 4
- 5
- 7 ✓
- -2
Question 31
In for a in __________ : , the blank can be filled with
- an iterable sequence ✓
- a range( ) function ✓
- a single value
- an expression
Question 32
Which of the following are entry controlled loops ?
- if
- if-else
- for ✓
- while ✓
Question 33
Consider the loop given below. What will be the final value of i after the loop?
for i in range(10) :
break
- 10
- 0 ✓
- Error
- 9
Question 34
The else statement can be a part of .......... statement in Python.
- if ✓
- def
- while ✓
- for ✓
Question 35
Which of the following are jump statements ?
- if
- break ✓
- while
- continue ✓
Question 36
Consider the following code segment :
for i in range(2, 4):
print(i)
What values(s) are printed when it executes?
- 2
- 3
- 2 and 3 ✓
- 3 and 4
- 2, 3 and 4
Question 37
When the following code runs, how many times is the line "x = x * 2" executed?
x = 1
while ( x < 20 ):
x = x * 2
- 2
- 5 ✓
- 19
- 4
- 32
Question 38
What is the output when this code executes ?
x = 1
while (x <= 5):
x + 1
print(x)
- 6
- 1
- 4
- 5
- no output ✓
Question 39
How many times does the following code execute ?
x = 1
while (x <= 5):
x + 1
print (x)
- 6
- 1
- 4
- 5
- infinite ✓
Question 40
What is the output produced when this code executes?
i = 1
while (i <= 7):
i*= 2
print (i)
- 8 ✓
- 16
- 4
- 14
- no output
Question 41
Consider the following loop:
j = 10
while j >= 5:
print("X")
j=j-1
Which of the following for loops will generate the same output as the loop shown previously?
- for j in range(-1, -5, -1):
print("X") - for j in range(0, 5):
print("X") - for j in range(10, -1, -2):
print("X") ✓ - for j in range(10, 5):
print("X") - for j in range(10, 5, -1):
print("X")
Question 42
What is the output produced when this code executes?
a = 0
for i in range(4,8):
if i % 2 == 0:
a = a + i
print (a)
- 4
- 8
- 10 ✓
- 18
Question 43
Which of the following code segments contain an example of a nested loop?
- for i in range(10):
print(i)
for j in range(10):
print(j) - for i in range(10):
print(i)
for j in range(10):
print(j) ✓ - for i in range(10):
print(i)
while i < 20:
print(i)
i = i + 1 ✓ - for i in range(10):
print(i)
while i < 20:
print(i)
Fill in the Blanks
Question 1
The if statement forms the selection construct in Python.
Question 2
The pass statement is a do nothing statement in Python.
Question 3
The for and while statements form the repetition construct in Python.
Question 4
Three constructs that govern the flow of control are sequence, selection/decision and repetition/iteration.
Question 5
In Python, indentation defines a block of statements.
Question 6
An if-else statement has less number of conditional checks than two successive ifs.
Question 7
The in operator tests if a given value is contained in a sequence or not.
Question 8
The two membership operators are in and not in.
Question 9
An iteration refers to one repetition of a loop.
Question 10
The for loop iterates over a sequence.
Question 11
The while loop tests a condition before executing the body-of-the-loop.
Question 12
The else clause can occur with an if as well as with loops.
Question 13
The else block of a loop gets executed when a loop ends normally.
Question 14
The else block of a loop will not get executed if a break statement has terminated the loop.
Question 15
The break statement terminates the execution of the whole loop.
Question 16
The continue statement terminates only a single iteration of the loop.
Question 17
The break and continue statements, together are called jump statements.
Question 18
In a nested loop, a break statement inside the inner loop, will terminate the inner loop only.
True/False Questions
Question 1
An if-else tests less number of conditions than two successive ifs.
True
Question 2
A for loop is termed as a determinable loop.
True
Question 3
The while loop is an exit controlled loop.
False
Question 4
The range( ) creates an iterable sequence.
True
Question 5
The for loop can also tests a condition before executing the loop-body.
False
Question 6
Only if statement can have an else clause.
False
Question 7
A loop can also take an else clause.
True
Question 8
The else clause of a loop gets executed only when a break statement terminates it.
False
Question 9
A loop with an else clause executes its else clause only when the loop terminates normally.
True
Question 10
A loop with an else clause cannot have a break statement.
False
Question 11
A continue statement can replace a break statement.
False
Question 12
For a for loop, an equivalent while loop can always be written.
True
Question 13
For a while loop, an equivalent for loop can always be written.
False
Question 14
The range( ) function can only be used in for loops.
False
Question 15
An if-elif-else statement is equivalent to a nested-if statement.
True
Type A : Short Answer Questions/Conceptual Questions
Question 1
What is the common structure of Python compound statements?
Answer
The common structure of a Python compound statement is as shown below:
<compound statement header>:
<indented body with multiple simple\
and/or compound statements>
It has the following components:
- A header line which begins with a keyword and ends with a colon.
- A body containing a sequence of statements at the same level of indentation.
Question 2
What is the importance of the three programming constructs?
Answer
The importance of the three programming constructs is a given below:
- Sequence — Statements get executed sequentially.
- Selection — Execution of statements depends on a condition test.
- Repetition\Iteration — Repetition of a set of statements depends on a condition test.
Question 3
What is empty statement in Python? What is its need?
Answer
In Python, an empty statement is pass statement. Its syntax is:
pass
When pass statement is encountered, Python does nothing and moves to next statement in the flow of control.
It is needed in those instances where the syntax of the language requires the presence of a statement but where the logic of the program does not.
Question 4
Which Python statement can be termed as empty statement?
Answer
In Python, an empty statement is pass statement. Its syntax is:
pass
Question 5
What is an algorithm?
Answer
An algorithm is defined as the sequence of instructions written in simple English that are required to get the desired results. It helps to develop the fundamental logic of a problem that leads to a solution.
Question 6
What is a flowchart? How is it useful?
Answer
A flowchart is a pictorial representation of an algorithm. It uses boxes of different shapes to represent different types of instructions. These boxes are connected with arrow marks to indicate the flow of operations. It helps in:
- Communication — The pictorial representation of the flowchart provides better communication. It is easier for the programmer to explain the logic of a program.
- Effective Analysis — It is a very useful technique, as flowchart is a pictorial representation that helps the programmer to analyze the problem in detail.
Question 7
Draw flowchart for displaying first 10 odd numbers.
Answer
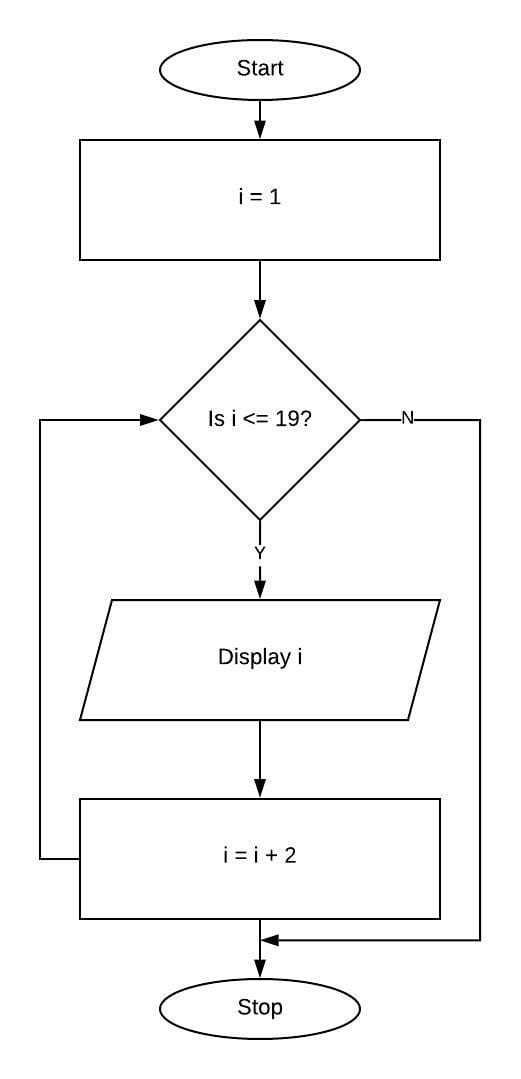
Question 8
What is entry-controlled loop?
Answer
An entry-controlled loop checks the condition at the time of entry. Only if the condition is true, the program control enters the body of the loop.
Question 9
What are the four elements of a while loop in Python?
Answer
The four elements of a while loop in Python are:
- Initialization Expressions — It initializes the loop control variable and it is given outside the while loop before the beginning of the loop.
- Test Expression — If its truth value is true then the loop-body gets executed otherwise not.
- The Body of the Loop — It is the set of statements that are executed repeatedly in loop.
- Update Expressions — It updates the value of loop control variable and it is given inside the while loop.
Question 10
What is the difference between else clause of if-else and else clause of Python loops?
Answer
The else clause of an if-else statement is executed when the condition of the if statement results into false. The else clause of a loop is executed when the loop is terminating normally i.e., when its test condition has become false for a while loop or when the for loop has executed for the last value in sequence.
Question 11
In which cases, the else clause of a loop does not get executed?
Answer
The else clause of a loop does not get executed if the loop is terminated due to the execution of a break statement inside the loop.
Question 12
What are jump statements? Name them.
Answer
Jump statements are used to unconditionally transfer program control to other parts within a program. Python provides the below jump statements:
- break
- continue
Question 13
How and when are named conditions useful?
Answer
Sometimes the conditions being used in the code are complex and repetitive. In such cases, to make the program more readable and maintainable, named conditions can be used.
Question 14
What are endless loops ? Why do such loops occur?
Answer
A loop which continues iterating indefinitely and never stops is termed as an endless or infinite loop. Such loops can occur primarily due to two reasons:
- Logical errors when the programmer misses updating the value of loop control variable.
- Purposefully created endless loops that have a break statement within their body to terminate the loop.
Question 15
How is break statement different from continue?
Answer
When the break statement gets executed, it terminates its loop completely and control reaches to the statement immediately following the loop. The continue statement terminates only the current iteration of the loop by skipping rest of the statements in the body of the loop.
Type B: Application Based Questions
Question 1
Rewrite the following code fragment that saves on the number of comparisons:
if (a == 0) :
print ("Zero")
if (a == 1) :
print ("One")
if (a == 2) :
print ("Two")
if (a == 3) :
print ("Three")
Answer
if (a == 0) :
print ("Zero")
elif (a == 1) :
print ("One")
elif (a == 2) :
print ("Two")
elif (a == 3) :
print ("Three")
Question 2
Under what conditions will this code fragment print "water"?
if temp < 32 :
print ("ice")
elif temp < 212:
print ("water")
else :
print ("steam")
Answer
When value of temp is greater than or equal to 32 and less than 212 then this code fragment will print "water".
Question 3
What is the output produced by the following code?
x = 1
if x > 3 :
if x > 4 :
print ("A", end = ' ')
else :
print ("B", end = ' ')
elif x < 2:
if (x != 0):
print ("C", end = ' ')
print ("D")
Answer
Output
C D
Explanation
As value of x is 1 so statements in the else part of outer if i.e. elif x < 2:
will get executed. The condition if (x != 0)
is true so C is printed. After that the statement print ("D")
prints D.
Question 4
What is the error in following code? Correct the code:
weather = 'raining'
if weather = 'sunny' :
print ("wear sunblock")
elif weather = "snow":
print ("going skiing")
else :
print (weather)
Answer
In this code, assignment operator (=) is used in place of equality operator (==) for comparison. The corrected code is below:
weather = 'raining'
if weather == 'sunny' :
print ("wear sunblock")
elif weather == "snow":
print ("going skiing")
else :
print (weather)
Question 5
What is the output of the following lines of code?
if int('zero') == 0 :
print ("zero")
elif str(0) == 'zero' :
print (0)
elif str(0) == '0' :
print (str(0))
else:
print ("none of the above")
Answer
The above lines of code will cause an error as in the line if int('zero') == 0 :
, 'zero' is given to int() function but string 'zero' doesn't have a valid numeric representation.
Question 6
Find the errors in the code given below and correct the code:
if n == 0
print ("zero")
elif : n == 1
print ("one")
elif
n == 2:
print ("two")
else n == 3:
print ("three")
Answer
The corrected code is below:
if n == 0 : #1st Error
print ("zero")
elif n == 1 : #2nd Error
print ("one")
elif n == 2: #3rd Error
print ("two")
elif n == 3: #4th Error
print ("three")
Question 7
What is following code doing? What would it print for input as 3?
n = int(input( "Enter an integer:" ))
if n < 1 :
print ("invalid value")
else :
for i in range(1, n + 1):
print (i * i)
Answer
The code will print the square of each number from 1 till the number given as input by the user if the input value is greater than 0. Output of the code for input as 3 is shown below:
Enter an integer:3
1
4
9
Question 8
How are following two code fragments different from one another? Also, predict the output of the following code fragments :
(a)
n = int(input( "Enter an integer:" ))
if n > 0 :
for a in range(1, n + n ) :
print (a / (n/2))
else :
print ("Now quiting")
(b)
n = int(input("Enter an integer:"))
if n > 0 :
for a in range(1, n + n) :
print (a / (n/2))
else :
print ("Now quiting")
Answer
In part (a) code, the else clause is part of the loop i.e. it is a loop else clause that will be executed when the loop terminates normally. In part (b) code, the else clause is part of the if statement i.e. it is an if-else clause. It won't be executed if the user gives a greater than 0 input for n.
Output of part a:
Enter an integer:3
0.6666666666666666
1.3333333333333333
2.0
2.6666666666666665
3.3333333333333335
Now quiting
Output of part b:
Enter an integer:3
0.6666666666666666
1.3333333333333333
2.0
2.6666666666666665
3.3333333333333335
Question 9a
Rewrite the following code fragment using for loop:
i = 100
while (i > 0) :
print (i)
i -= 3
Answer
for i in range(100, 0, -3) :
print (i)
Question 9b
Rewrite the following code fragment using for loop:
while num > 0 :
print (num % 10)
num = num/10
Answer
l = [1]
for x in l:
l.append(x + 1)
if num <= 0:
break
print (num % 10)
num = num/10
Question 9c
Rewrite the following code fragment using for loop:
while num > 0 :
count += 1
sum += num
num –= 2
if count == 10 :
print (sum/float(count))
break
Answer
for i in range(num, 0, -2):
count += 1
sum += i
if count == 10 :
print (sum/float(count))
break
Question 10a
Rewrite following code fragment using while loops :
min = 0
max = num
if num < 0 :
min = num
max = 0 # compute sum of integers
# from min to max
for i in range(min, max + 1):
sum += i
Answer
min = 0
max = num
if num < 0 :
min = num
max = 0 # compute sum of integers
# from min to max
i = min
while i <= max:
sum += i
i += 1
Question 10b
Rewrite following code fragment using while loops :
for i in range(1, 16) :
if i % 3 == 0 :
print (i)
Answer
i = 1
while i < 16:
if i % 3 == 0 :
print (i)
i += 1
Question 10c
Rewrite following code fragment using while loops :
for i in range(4) :
for j in range(5):
if i + 1 == j or j + 1 == 4 :
print ("+", end = ' ')
else :
print ("o", end = ' ')
print()
Answer
i = 0
while i < 4:
j = 0
while j < 5:
if i + 1 == j or j + 1 == 4 :
print ("+", end = ' ')
j += 1
else :
print ("o", end = ' ')
i += 1
print()
Question 11a
Predict the output of the following code fragments:
count = 0
while count < 10:
print ("Hello")
count += 1
Answer
Output
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Explanation
The while loop executes 10 times so "Hello" is printed 10 times
Question 11b
Predict the output of the following code fragments:
x = 10
y = 0
while x > y:
print (x, y)
x = x - 1
y = y + 1
Answer
Output
10 0
9 1
8 2
7 3
6 4
Explanation
x | y | Output | Remarks |
---|---|---|---|
10 | 0 | 10 0 | 1st Iteration |
9 | 1 | 10 0 9 1 | 2nd Iteration |
8 | 2 | 10 0 9 1 8 2 | 3rd Iteration |
7 | 3 | 10 0 9 1 8 2 7 3 | 4th Iteration |
6 | 4 | 10 0 9 1 8 2 7 3 6 4 | 5th Iteration |
Question 11c
Predict the output of the following code fragments:
keepgoing = True
x=100
while keepgoing :
print (x)
x = x - 10
if x < 50 :
keepgoing = False
Answer
Output
100
90
80
70
60
50
Explanation
Inside while loop, the line x = x - 10
is decreasing x by 10 so after 5 iterations of while loop x will become 40. When x becomes 40, the condition if x < 50
becomes true so keepgoing
is set to False
due to which the while loop stops iterating.
Question 11d
Predict the output of the following code fragments:
x = 45
while x < 50 :
print (x)
Answer
This is an endless (infinite) loop that will keep printing 45 continuously.
As the loop control variable x is not updated inside the loop neither there is any break statement inside the loop so it becomes an infinite loop.
Question 11e
Predict the output of the following code fragments:
for x in [1,2,3,4,5]:
print (x)
Answer
Output
1
2
3
4
5
Explanation
x will be assigned each of the values from the list one by one and that will get printed.
Question 11f
Predict the output of the following code fragments:
for x in range(5):
print (x)
Answer
Output
0
1
2
3
4
Explanation
range(5)
will generate a sequence like this [0, 1, 2, 3, 4]. x will be assigned each of the values from this sequence one by one and that will get printed.
Question 11g
Predict the output of the following code fragments:
for p in range(1,10):
print (p)
Answer
Output
1
2
3
4
5
6
7
8
9
Explanation
range(1,10)
will generate a sequence like this [1, 2, 3, 4, 4, 5, 6, 7, 8, 9]. p will be assigned each of the values from this sequence one by one and that will get printed.
Question 11h
Predict the output of the following code fragments:
for q in range(100, 50, -10):
print (q)
Answer
Output
100
90
80
70
60
Explanation
range(100, 50, -10) will generate a sequence like this [100, 90, 80, 70, 60]. q will be assigned each of the values from this sequence one by one and that will get printed.
Question 11i
Predict the output of the following code fragments:
for z in range(-500, 500, 100):
print (z)
Answer
Output
-500
-400
-300
-200
-100
0
100
200
300
400
Explanation
range(-500, 500, 100)
generates a sequence of numbers from -500 to 400 with each subsequent number incrementing by 100. Each number of this sequence is assigned to z
one by one and then z
gets printed inside the for loop.
Question 11j
Predict the output of the following code fragments:
for y in range(500, 100, 100):
print (" * ", y)
Answer
This code generates No Output.
The for loop doesn't execute as range(500, 100, 100)
returns an empty sequence — [ ].
Question 11k
Predict the output of the following code fragments:
x = 10
y = 5
for i in range(x-y * 2):
print (" % ", i)
Answer
This code generates No Output.
Explanation
The x-y * 2
in range(x-y * 2)
is evaluated as below:
x - y * 2
⇒ 10 - 5 * 2
⇒ 10 - 10 [∵ * has higher precedence than -]
⇒ 0
Thus range(x-y * 2)
is equivalent to range(0)
which returns an empty sequence — [ ].
Question 11l
Predict the output of the following code fragments:
for x in [1,2,3]:
for y in [4, 5, 6]:
print (x, y)
Answer
Output
1 4
1 5
1 6
2 4
2 5
2 6
3 4
3 5
3 6
Explanation
For each iteration of outer loop, the inner loop will execute three times generating this output.
Question 11m
Predict the output of the following code fragments:
for x in range(3):
for y in range(4):
print (x, y, x + y)
Answer
Output
0 0 0
0 1 1
0 2 2
0 3 3
1 0 1
1 1 2
1 2 3
1 3 4
2 0 2
2 1 3
2 2 4
2 3 5
Explanation
For each iteration of outer loop, the inner loop executes four times (with value of y ranging from 0 to 3) generating this output.
Question 11n
Predict the output of the following code fragments:
c = 0
for x in range(10):
for y in range(5):
c += 1
print (c)
Answer
Output
50
Explanation
Outer loop executes 10 times. For each iteration of outer loop, inner loop executes 5 times. Thus, the statement c += 1
is executed 10 * 5 = 50 times. c is incremented by 1 in each execution so final value of c becomes 50.
Question 12
What is the output of the following code?
for i in range(4):
for j in range(5):
if i + 1 == j or j + i == 4:
print ("+", end = ' ')
else:
print ("o", end = ' ')
print()
Answer
Output
o + o o + o o + + o o o + + o o + o o +
Explanation
Outer loop executes 4 times. For each iteration of outer loop, inner loop executes 5 times. Therefore, the total number of times body of inner loop gets executed is 4 * 5 = 20. Thats why there are 20 characters in the output (leaving spaces). When the condition is true then + is printed else o is printed.
Question 13
In the nested for loop code below, how many times is the condition of the if clause evaluated?
for i in range(4):
for j in range(5):
if i + 1 == j or j + i == 4:
print ("+", end = ")
else:
print ("o", end = ")
print()
Answer
Outer loop executes 4 times. For each iteration of outer loop, inner loop executes 5 times. Therefore, the total number of times the condition of the if clause gets evaluated is 4 * 5 = 20.
Question 14
Which of the following Python programs implement the control flow graph shown?
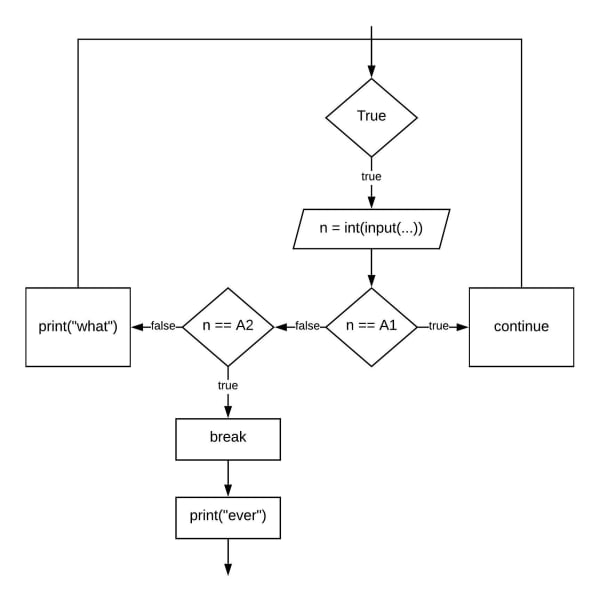
(a)
while True :
n = int(input("Enter an int:"))
if n == A1 :
continue
elif n == A2 :
break
else :
print ("what")
else:
print ("ever")
(b)
while True :
n = int(input("Enter an int:"))
if n == A1 :
continue
elif n == A2 :
break
else :
print ("what")
print ("ever")
(c)
while True :
n = int(input("Enter an int:"))
if n == A1 :
continue
elif n == A2 :
break
print ("what")
print ("ever")
Answer
Python program given in Option (b) implements this flowchart:
while True :
n = int(input("Enter an int:"))
if n == A1 :
continue
elif n == A2 :
break
else :
print ("what")
print ("ever")
Question 15
Find the error. Consider the following program :
a = int(input("Enter a value: "))
while a != 0:
count = count + 1
a = int(input("Enter a value: "))
print("You entered", count, "values.")
It is supposed to count the number of values entered by the user until the user enters 0 and then display the count (not including the 0). However, when the program is run, it crashes with the following error message after the first input value is read :
Enter a value: 14
Traceback (most recent call last):
File "count.py", line 4, in <module>
count = count + 1
NameError: name 'count' is not defined
What change should be made to this program so that it will run correctly ? Write the modification that is needed into the program above, crossing out any code that should be removed and clearly indicating where any new code should be inserted.
Answer
The line count = count + 1
is incrementing the value of variable count
by 1 but the variable count
has not been initialized before this statement. This causes an error when trying to execute the program. The corrected program is below:
count = 0 #count should be initialized before incrementing
a = int(input("Enter a value: "))
while a != 0:
count = count + 1
a = int(input("Enter a value: "))
print("You entered", count, "values.")
Type C: Programming Practice/Knowledge based Questions
Question 1
Write a Python script that asks the user to enter a length in centimetres. If the user enters a negative length, the program should tell the user that the entry is invalid. Otherwise, the program should convert the length to inches and print out the result. There are 2.54 centimetres in an inch.
Solution
len = int(input("Enter length in cm: "))
if len < 0:
print("Invalid input")
else:
inch = len / 2.54
print(len, "centimetres is equal to", inch, "inches")
Output
Enter length in cm: 150
150 centimetres is equal to 59.05511811023622 inches
Question 2
A store charges ₹120 per item if you buy less than 10 items. If you buy between 10 and 99 items, the cost is ₹100 per item. If you buy 100 or more items, the cost is ₹70 per item. Write a program that asks the user how many items they are buying and prints the total cost.
Solution
n = int(input("Enter number of items: "))
cost = 0
if n >= 100 :
cost = n * 70
elif n >= 10 :
cost = n * 100
else :
cost = n * 120
print("Total Cost =", cost)
Output
Enter number of items: 58
Total Cost = 5800
Question 3
Write a program that reads from user — (i) an hour between 1 to 12 and (ii) number of hours ahead. The program should then print the time after those many hours, e.g.,
Enter hour between 1-12 : 9
How many hours ahead : 4
Time at that time would be : 1 O'clock
Solution
hr = int(input("Enter hour between 1-12 : "))
n = int(input("How many hours ahead : "))
s = hr + n
if s > 12:
s -= 12
print("Time at that time would be : ", s, "O'clock")
Output
Enter hour between 1-12 : 9
How many hours ahead : 4
Time at that time would be : 1 O'clock
Question 4
Write a program that asks the user for two numbers and prints Close if the numbers are within .001 of each other and Not close otherwise.
Solution
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
d = 0
if a > b :
d = a - b
else :
d = b - a
if d <= 0.001 :
print("Close")
else :
print("Not Close")
Output
Enter first number: 10.12345
Enter second number: 10.12354
Close
Question 5
A year is a leap year if it is divisible by 4, except that years divisible by 100 are not leap years unless they are also divisible by 400. Write a program that asks the user for a year and prints out whether it is a leap year or not.
Solution
year = int(input("Enter year: "))
if year % 400 == 0 :
print(year, "is a Leap Year")
elif year % 100 == 0 :
print(year, "is not a Leap Year")
elif year % 4 == 0 :
print(year, "is a Leap Year")
else :
print(year, "is not a Leap Year")
Output
Enter year: 1800
1800 is not a Leap Year
Question 6
Write a program to input length of three sides of a triangle. Then check if these sides will form a triangle or not.
(Rule is: a+b>c;b+c>a;c+a>b)
Solution
a = int(input("Enter first side : "))
b = int(input("Enter second side : "))
c = int(input("Enter third side : "))
if a + b > c and b + c > a and a + c > b :
print("Triangle Possible")
else :
print("Triangle Not Possible")
Output
Enter first side : 3
Enter second side : 5
Enter third side : 6
Triangle Possible
Question 7
Write a short program to input a digit and print it in words.
Solution
d = int(input("Enter a digit(0-9): "))
if d == 0 :
print("Zero")
elif d == 1 :
print("One")
elif d == 2 :
print("Two")
elif d == 3 :
print("Three")
elif d == 4 :
print("Four")
elif d == 5 :
print("Five")
elif d == 6 :
print("Six")
elif d == 7 :
print("Seven")
elif d == 8 :
print("Eight")
elif d == 9 :
print("Nine")
else :
print("Invalid Digit")
Output
Enter a digit(0-9): 6
Six
Question 8
Write a short program to check whether square root of a number is prime or not.
Solution
import math
n = int(input("Enter a number: "))
sr = math.sqrt(n)
c = 0
for i in range(1, int(sr + 1)) :
if (sr % i == 0) :
c += 1
if c == 2 :
print("Square root is prime")
else :
print("Square root is not prime")
Output
Enter a number: 49
Square root is prime
Question 9
Write a short program to print first n odd numbers in descending order.
Solution
n = int(input("Enter n: "))
x = n * 2 - 1
for i in range(x, 0, -2) :
print(i)
Output
Enter n: 5
9
7
5
3
1
Question 10
Write a short program to print the following series :
(i) 1 4 7 10 .......... 40.
(ii) 1 -4 7 -10 .......... -40
Solution
print("First Series:")
for i in range(1, 41, 3) :
print(i, end = ' ')
print("\nSecond Series:")
x = 1
for i in range(1, 41, 3) :
print(i * x, end = ' ')
x *= -1
Output
First Series:
1 4 7 10 13 16 19 22 25 28 31 34 37 40
Second Series:
1 -4 7 -10 13 -16 19 -22 25 -28 31 -34 37 -40
Question 11
Write a short program to find average of list of numbers entered through keyboard.
Solution
sum = count = 0
print("Enter numbers")
print("(Enter 'q' to see the average)")
while True :
n = input()
if n == 'q' or n == 'Q' :
break
else :
sum += int(n)
count += 1
avg = sum / count
print("Average = ", avg)
Output
Enter numbers
(Enter 'q' to see the average)
2
5
7
15
12
q
Average = 8.2
Question 12
Write a program to input 3 sides of a triangle and print whether it is an equilateral, scalene or isosceles triangle.
Solution
a = int(input("Enter first side : "))
b = int(input("Enter second side : "))
c = int(input("Enter third side : "))
if a == b and b == c :
print("Equilateral Triangle")
elif a == b or b == c or c == a:
print("Isosceles Triangle")
else :
print("Scalene Triangle")
Output
Enter first side : 10
Enter second side : 5
Enter third side : 10
Isosceles Triangle
Question 13
Write a program to take an integer a as an input and check whether it ends with 4 or 8. If it ends with 4, print "ends with 4", if it ends with 8, print "ends with 8", otherwise print "ends with neither".
Solution
a = int(input("Enter an integer: "))
if a % 10 == 4 :
print("ends with 4")
elif a % 10 == 8 :
print("ends with 8")
else :
print("ends with neither")
Output
Enter an integer: 18
ends with 8
Question 14
Write a program to take N (N > 20) as an input from the user. Print numbers from 11 to N. When the number is a multiple of 3, print "Tipsy", when it is a multiple of 7, print "Topsy". When it is a multiple of both, print "TipsyTopsy".
Solution
n = int(input("Enter a number greater than 20: "))
if n <= 20 :
print("Invalid Input")
else :
for i in range(11, n + 1) :
print(i)
if i % 3 == 0 and i % 7 == 0 :
print("TipsyTopsy")
elif i % 3 == 0 :
print("Tipsy")
elif i % 7 == 0 :
print("Topsy")
Output
Enter a number greater than 20: 25
11
12
Tipsy
13
14
Topsy
15
Tipsy
16
17
18
Tipsy
19
20
21
TipsyTopsy
22
23
24
Tipsy
25
Question 15
Write a short program to find largest number of a list of numbers entered through keyboard.
Solution
print("Enter numbers:")
print("(Enter 'q' to see the result)")
l = input()
if l != 'q' and l != 'Q' :
l = int(l)
while True:
n = input()
if n == 'q' or n == 'Q' :
break
n = int(n)
if n > l :
l = n
print("Largest Number =", l)
Output
Enter numbers:
(Enter 'q' to see the result)
3
5
8
2
4
q
Largest Number = 8
Question 16
Write a program to input N numbers and then print the second largest number.
Solution
n = int(input("How many numbers you want to enter? "))
if n > 1 :
l = int(input()) # Assume first input is largest
sl = int(input()) # Assume second input is second largest
if sl > l :
t = sl
sl = l
l = t
for i in range(n - 2) :
a = int(input())
if a > l :
sl = l
l = a
elif a > sl :
sl = a
print("Second Largest Number =", sl)
else :
print("Please enter more than 1 number")
Output
How many numbers you want to enter? 5
55
25
36
12
18
Second Largest Number = 36
Question 17
Given a list of integers, write a program to find those which are palindromes. For example, the number 4321234 is a palindrome as it reads the same from left to right and from right to left.
Solution
print("Enter numbers:")
print("(Enter 'q' to stop)")
while True :
n = input()
if n == 'q' or n == 'Q' :
break
n = int(n)
t = n
r = 0
while (t != 0) :
d = t % 10
r = r * 10 + d
t = t // 10
if (n == r) :
print(n, "is a Palindrome Number")
else :
print(n, "is not a Palindrome Number")
Output
Enter numbers:
(Enter 'q' to stop)
67826
67826 is not a Palindrome Number
4321234
4321234 is a Palindrome Number
256894
256894 is not a Palindrome Number
122221
122221 is a Palindrome Number
q
Question 18
Write a complete Python program to do the following :
(i) read an integer X.
(ii) determine the number of digits n in X.
(iii) form an integer Y that has the number of digits n at ten's place and the most significant digit of X at one's place.
(iv) Output Y.
(For example, if X is equal to 2134, then Y should be 42 as there are 4 digits and the most significant number is 2).
Solution
x = int(input("Enter an integer: "))
temp = x
count = 0
digit = -1
while temp != 0 :
digit = temp % 10
count += 1
temp = temp // 10
y = count * 10 + digit
print("Y =", y)
Output
Enter an integer: 2134
Y = 42
Question 19
Write a Python program to print every integer between 1 and n divisible by m. Also report whether the number that is divisible by m is even or odd.
Solution
m = int(input("Enter m: "))
n = int(input("Enter n: "))
for i in range(1, n) :
if i % m == 0 :
print(i, "is divisible by", m)
if i % 2 == 0 :
print(i, "is even")
else :
print(i, "is odd")
Output
Enter m: 3
Enter n: 20
3 is divisible by 3
3 is odd
6 is divisible by 3
6 is even
9 is divisible by 3
9 is odd
12 is divisible by 3
12 is even
15 is divisible by 3
15 is odd
18 is divisible by 3
18 is even
Question 20a
Write Python programs to sum the given sequences:
2/9 - 5/13 + 8/17 ...... (print 7 terms)
Solution
n = 2 #numerator initial value
d = 9 #denominator initial value
m = 1 #to add/subtract alternate terms
sum = 0
for i in range(7) :
t = n / d
sum += t * m
n += 3
d += 4
m *= -1
print("Sum =", sum)
Output
Sum = 0.3642392586003134
Question 20b
Write Python programs to sum the given sequences:
12 + 32 + 52 + ..... + n2 (Input n)
Solution
n = int(input("Enter the value of n: "))
i = 1
sum = 0
while i <= n :
sum += i ** 2
i += 2
print("Sum =", sum)
Output
Enter the value of n: 9
Sum = 165
Question 21
Write a Python program to sum the sequence:
1 + 1/1! + 1/2! + 1/3! + ..... + 1/n! (Input n)
Solution
n = int(input("Enter the value of n: "))
sum = 0
for i in range(n + 1) :
fact = 1
for j in range(1, i) :
fact *= j
term = 1 / fact
sum += term
print("Sum =", sum)
Output
Sum = 3.708333333333333
Question 22
Write a program to accept the age of n employees and count the number of persons in the following age group:
(i) 26 - 35
(ii) 36 - 45
(iii) 46 - 55
Solution
n = int(input("Enter the value of n: "))
g1 = g2 = g3 = 0
for i in range(1, n + 1) :
age = int(input("Enter employee age: "))
#We have used chained comparison operators
if 26 <= age <= 35 :
g1 += 1
elif 36 <= age <= 45 :
g2 += 1
elif 46 <= age <= 55 :
g3 += 1
print("Employees in age group 26 - 35: ", g1)
print("Employees in age group 36 - 45: ", g2)
print("Employees in age group 46 - 55: ", g3)
Output
Enter the value of n: 10
Enter employee age: 45
Enter employee age: 53
Enter employee age: 28
Enter employee age: 32
Enter employee age: 34
Enter employee age: 49
Enter employee age: 30
Enter employee age: 38
Enter employee age: 33
Enter employee age: 53
Employees in age group 26 - 35: 5
Employees in age group 36 - 45: 2
Employees in age group 46 - 55: 3
Question 23a
Write programs to find the sum of the following series:
x - x2/2! + x3/3! - x4/4! + x5/5! - x6/6! (Input x)
Solution
x = int(input("Enter the value of x: "))
sum = 0
m = 1
for i in range(1, 7) :
fact = 1
for j in range(1, i+1) :
fact *= j
term = x ** i / fact
sum += term * m
m = m * -1
print("Sum =", sum)
Output
Enter the value of x: 2
Sum = 0.8444444444444444
Question 23b
Write programs to find the sum of the following series:
x + x2/2 + x3/3 + ...... + xn/n (Input x and n both)
Solution
x = int(input("Enter the value of x: "))
n = int(input("Enter the value of n: "))
sum = 0
for i in range(1, n + 1) :
term = x ** i / i
sum += term
print("Sum =", sum)
Output
Enter the value of x: 2
Enter the value of n: 5
Sum = 17.066666666666666
Question 24a
Write programs to print the following shapes:
*
* *
* * *
* *
*
Solution
n = 3 # number of rows
# upper half
for i in range(n) :
for j in range(n, i+1, -1) :
print(' ', end = '')
for k in range(i+1) :
print('*', end = ' ')
print()
# lower half
for i in range(n-1) :
for j in range(i + 1) :
print(' ', end = '')
for k in range(n-1, i, -1) :
print('*', end = ' ')
print()
Output
*
* *
* * *
* *
*
Question 24b
Write programs to print the following shapes:
*
* *
* * *
* *
*
Solution
n = 3 # number of rows
# upper half
for i in range(n) :
for k in range(i+1) :
print('*', end = ' ')
print()
# lower half
for i in range(n-1) :
for k in range(n-1, i, -1) :
print('*', end = ' ')
print()
Output
*
* *
* * *
* *
*
Question 24c
Write programs to print the following shapes:
*
* *
* *
* *
*
Solution
n = 3 # number of rows
# upper half
for i in range(1, n+1) :
# for loop for initial spaces
for j in range(n, i, -1) :
print(' ', end = '')
#while loop for * and spaces
x = 1
while x < 2 * i :
if x == 1 or x == 2 * i - 1 :
print('*', end = '')
else :
print(' ', end = '')
x += 1
print()
# lower half
for i in range(n-1, 0, -1) :
# for loop for initial spaces
for j in range(n, i, -1) :
print(' ', end = '')
#while loop for * and spaces
x = 1
while x < 2 * i :
if x == 1 or x == 2 * i - 1 :
print('*', end = '')
else :
print(' ', end = '')
x += 1
print()
Output
*
* *
* *
* *
*
Question 24d
Write programs to print the following shapes:
*
* *
* *
* *
* *
* *
*
Solution
n = 4 # number of row
#upper half
for i in range(1, n+1) :
#while loop for * and spaces
x = 1
while x < 2 * i :
if x == 1 or x == 2 * i - 1 :
print('*', end = '')
else :
print(' ', end = '')
x += 1
print()
#lower half
for i in range(n-1, 0, -1) :
#while loop for * and spaces
x = 1
while x < 2 * i :
if x == 1 or x == 2 * i - 1 :
print('*', end = '')
else :
print(' ', end = '')
x += 1
print()
Output
*
* *
* *
* *
* *
* *
*
Question 25a
Write programs using nested loops to produce the following patterns:
A
A B
A B C
A B C D
A B C D E
A B C D E F
Solution
n = 6
for i in range(n) :
t = 65
for j in range(i + 1) :
print(chr(t), end = ' ')
t += 1
print()
Output
A
A B
A B C
A B C D
A B C D E
A B C D E F
Question 25b
Write programs using nested loops to produce the following patterns:
A
B B
C C C
D D D D
E E E E E
Solution
n = 5
t = 65
for i in range(n) :
for j in range(i + 1) :
print(chr(t), end = ' ')
t += 1
print()
Output
A
B B
C C C
D D D D
E E E E E
Question 25c
Write programs using nested loops to produce the following patterns:
0
2 2
4 4 4
6 6 6 6
8 8 8 8 8
Solution
for i in range(0, 10, 2):
for j in range(0, i + 1, 2) :
print(i, end = ' ')
print()
Output
0
2 2
4 4 4
6 6 6 6
8 8 8 8 8
Question 25d
Write programs using nested loops to produce the following patterns:
2
4 4
6 6 6
8 8 8 8
Solution
for i in range(2, 10, 2) :
for j in range(2, i + 1, 2) :
print(i, end = ' ')
print()
Output
2
4 4
6 6 6
8 8 8 8
Question 26
Write a program using nested loops to produce a rectangle of *'s with 6 rows and 20 *'s per row.
Solution
for i in range(6) :
for j in range(20) :
print('*', end = '')
print()
Output
********************
********************
********************
********************
********************
********************
Question 27
Given three numbers A, B and C, write a program to write their values in an ascending order. For example, if A = 12, B = 10, and C = 15, your program should print out:
Smallest number = 10
Next higher number = 12
Highest number = 15
Solution
a = int(input("Enter first number: "))
b = int(input("Enter second number: "))
c = int(input("Enter third number: "))
if a < b and a < c :
small = a
if b < c :
middle = b
large = c
else :
middle = c
large = b
elif b < a and b < c :
small = b
if a < c :
middle = a
large = c
else :
middle = c
large = a
else :
small = c
if a < b :
middle = a
large = b
else :
middle = b
large = a
print("Smallest number =", small)
print("Next higher number =", middle)
print("Highest number =", large)
Output
Enter first number: 10
Enter second number: 5
Enter third number: 15
Smallest number = 5
Next higher number = 10
Highest number = 15
Question 28
Write a Python script to input temperature. Then ask them what units, Celsius or Fahrenheit, the temperature is in. Your program should convert the temperature to the other unit. The conversions are:
F = 9/5C + 32 and C = 5/9 (F 32).
Solution
temp = float(input("Enter Temperature: "))
unit = input("Enter unit('C' for Celsius or 'F' for Fahrenheit): ")
if unit == 'C' or unit == 'c' :
newTemp = 9 / 5 * temp + 32
print("Temperature in Fahrenheit =", newTemp)
elif unit == 'F' or unit == 'f' :
newTemp = 5 / 9 * (temp - 32)
print("Temperature in Celsius =", newTemp)
else :
print("Unknown unit", unit)
Output
Enter Temperature: 38
Enter unit('C' for Celsius or 'F' for Fahrenheit): C
Temperature in Fahrenheit = 100.4
Question 29
Ask the user to enter a temperature in Celsius. The program should print a message based on the temperature:
- If the temperature is less than -273.15, print that the temperature is invalid because it is below absolute zero.
- If it is exactly -273.15, print that the temperature is absolute 0.
- If the temperature is between -273.15 and 0, print that the temperature is below freezing.
- If it is 0, print that the temperature is at the freezing point.
- If it is between 0 and 100, print that the temperature is in the normal range.
- If it is 100, print that the temperature is at the boiling point.
- If it is above 100, print that the temperature is above the boiling point.
Solution
temp = float(input("Enter Temperature in Celsius: "))
if temp < -273.15 :
print("Temperature is invalid as it is below absolute zero")
elif temp == -273.15 :
print("Temperature is absolute zero")
elif -273.15 <= temp < 0:
print("Temperature is below freezing")
elif temp == 0 :
print("Temperature is at the freezing point")
elif 0 < temp < 100:
print("Temperature is in the normal range")
elif temp == 100 :
print("Temperature is at the boiling point")
else :
print("Temperature is above the boiling point")
Output
Enter Temperature in Celsius: -273.15
Temperature is absolute zero
Question 30
Write a program to display all of the integers from 1 up to and including some integer entered by the user followed by a list of each number's prime factors. Numbers greater than 1 that only have a single prime factor will be marked as prime.
For example, if the user enters 10 then the output of the program should be:
Enter the maximum value to display: 10
1 = 1
2 = 2 (prime)
3 = 3 (prime)
4 = 2x2
5 = 5 (prime)
6 = 2x3
7 = 7 (prime)
8 = 2x2x2
9 = 3x3
10 = 2x5
Solution
import math
n = int(input("Enter an integer: "))
for i in range(1, n + 1) :
if i == 1:
print("1 = 1")
else :
print(i, "=", end=' ')
c = 0
for j in range(1, i + 1) :
if i % j == 0:
c += 1
if c == 2:
print(i, "(prime)", end = '')
print()
else :
t = i
while t % 2 == 0 :
print("2", end='x')
t = t // 2
k = 3
x = math.ceil(math.sqrt(t)) + 1
while k <= x :
while (t % k == 0) :
print(k, end='x')
t = t // k
k += 2
if t > 2 :
print(t, end='x')
print()
Output
Enter an integer: 10
1 = 1
2 = 2 (prime)
3 = 3 (prime)
4 = 2x2x
5 = 5 (prime)
6 = 2x3x
7 = 7 (prime)
8 = 2x2x2x
9 = 3x3x
10 = 2x5x