Computer Applications
Using switch statement, write a menu driven program for the following:
(a) To find and display the sum of the series given below:
S = x1 - x2 + x3 - x4 + x5 - ………………….. - x20
(b) To find and display the sum of the series given below:
S = ………………….. to n terms
For an incorrect option, an appropriate error message should be displayed.
Java
Java Iterative Stmts
ICSE
5 Likes
Answer
import java.util.Scanner;
public class KboatSeriesSum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Sum of x^1 - x^2 + .... - x^20");
System.out.println("2. Sum of 1/a^2 + 1/a^4 + .... to n terms");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
System.out.print("Enter the value of x: ");
int x = in.nextInt();
long sum1 = 0;
for (int i = 1; i <= 20; i++) {
int term = (int)Math.pow(x, i);
if (i % 2 == 0)
sum1 -= term;
else
sum1 += term;
}
System.out.println("Sum = " + sum1);
break;
case 2:
System.out.print("Enter the number of terms: ");
int n = in.nextInt();
System.out.print("Enter the value of a: ");
int a = in.nextInt();
int c = 2;
double sum2 = 0;
for(int i = 1; i <= n; i++) {
sum2 += (1/Math.pow(a, c));
c += 2;
}
System.out.println("Sum = " + sum2);
break;
default:
System.out.println("Incorrect choice");
break;
}
}
}
Output
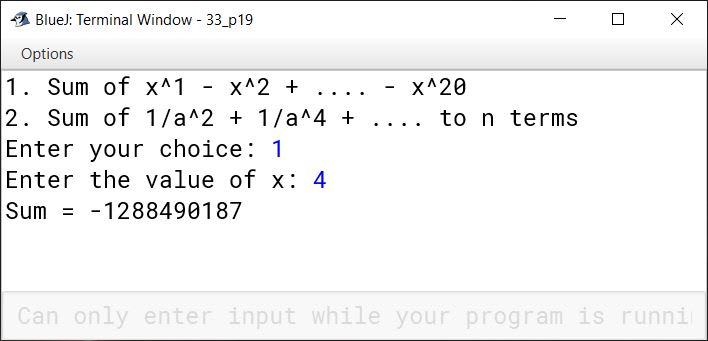
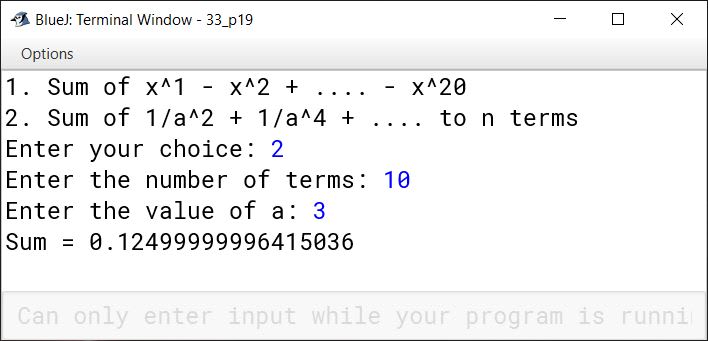
Answered By
3 Likes
Related Questions
You can multiply two numbers 'm' and 'n' by repeated addition method.
For example, 5 * 3 = 15 can be performed by adding 5 three times ⇒ 5 + 5 + 5 = 15Similarly, successive subtraction of two numbers produces 'Quotient' and 'Remainder' when a number 'a' is divided by 'b' (a>b).
For example, 5/2 ⇒ Quotient = 2 and Remainder = 1
Follow steps shown below:Process Result Counter 5 - 2 3 1 3 - 2 1 2 Sample Output: The last counter value represents 'Quotient' ⇒ 2
The last result value represents 'Remainder' ⇒ 1Write a program to accept two numbers. Perform multiplication and division of the numbers as per the process shown above by using switch case statement.
Using a switch statement, write a menu driven program to:
(a) generate and display the first 10 terms of the Fibonacci series
0, 1, 1, 2, 3, 5
The first two Fibonacci numbers are 0 and 1, and each subsequent number is the sum of the previous two.(b) find the sum of the digits of an integer that is input by the user.
Sample Input: 15390
Sample Output: Sum of the digits = 18
For an incorrect choice, an appropriate error message should be displayed.Write a program to display all Pronic numbers in the range from 1 to n.
Hint: A Pronic number is a number which is the product of two consecutive numbers in the form of n * (n + 1).
For example;
2, 6, 12, 20, 30,………..are Pronic numbers.Write a menu driven program to perform the following tasks:
(a) Tribonacci numbers are a sequence of numbers similar to Fibonacci numbers, except that a number is formed by adding the three previous numbers. Write a program to display the first twenty Tribonacci numbers.
For example;
1, 1, 2, 4, 7, 13, ………………….(b) Write a program to display all Sunny numbers in the range from 1 to n.
[Hint: A number is said to be sunny number if square root of (n+1) is an integer.]
For example,
3, 8, 15, 24, 35,………………….are sunny numbers.For an incorrect option, an appropriate error message should be displayed.