Computer Applications
Write a menu driven program in Java to accept a number and perform the following operations depending on the user's choice:
- Entered number is a Buzz number or not.
- Entered number is even or odd.
- Entered number is positive or negative.
Note: A Buzz number is a number which is either divisible by 7 or has 7 in its unit's place.
Java
Java Conditional Stmts
ICSE
63 Likes
Answer
import java.util.Scanner;
public class KboatMenu
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for Buzz Number");
System.out.println("Type 2 for Even/Odd");
System.out.println("Type 3 for Positive/Negative");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
System.out.print("Enter number: ");
int n = in.nextInt();
switch (ch) {
case 1:
if (n % 7 == 0 || n % 10 == 7)
System.out.println(n + " is a Buzz Number");
else
System.out.println(n + " is not a Buzz Number");
break;
case 2:
if (n % 2 == 0)
System.out.println(n + " is a Even Number");
else
System.out.println(n + " is an Odd Number");
break;
case 3:
if (n >= 0)
System.out.println(n + " is a Positive Number");
else
System.out.println(n + " is a Negative Number");
break;
default:
System.out.println("Incorrect choice");
}
}
}
Output
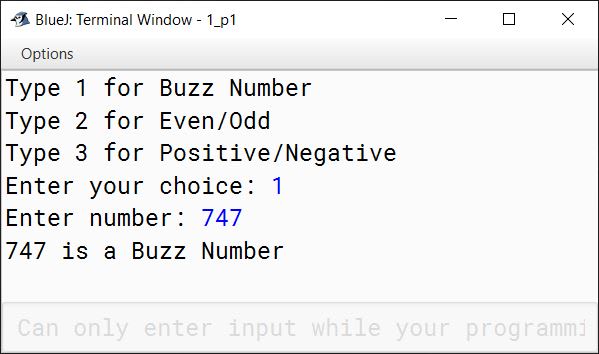
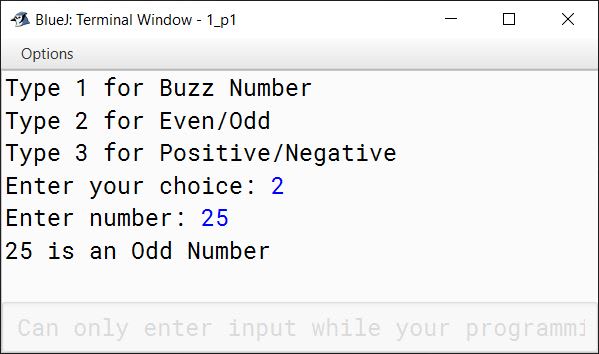
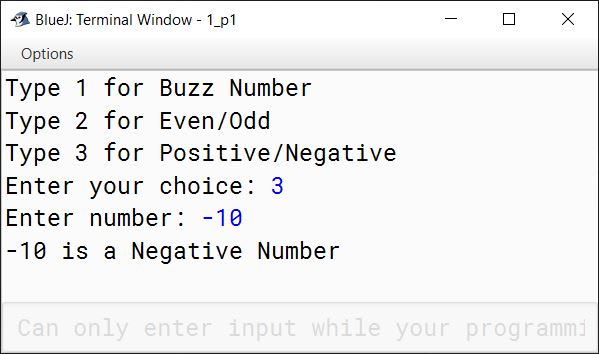
Answered By
13 Likes