Computer Applications
Write a program to perform binary search on a list of integers given below, to search for an element input by the user. If it is found display the element along with its position, otherwise display the message "Search element not found".
5, 7, 9, 11, 15, 20, 30, 45, 89, 97
Java
Java Arrays
ICSE
142 Likes
Answer
import java.util.Scanner;
public class KboatBinarySearch
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = {5, 7, 9, 11, 15, 20, 30, 45, 89, 97};
System.out.print("Enter number to search: ");
int n = in.nextInt();
int l = 0, h = arr.length - 1, index = -1;
while (l <= h) {
int m = (l + h) / 2;
if (arr[m] < n)
l = m + 1;
else if (arr[m] > n)
h = m - 1;
else {
index = m;
break;
}
}
if (index == -1) {
System.out.println("Search element not found");
}
else {
System.out.println(n + " found at position " + index);
}
}
}
Output
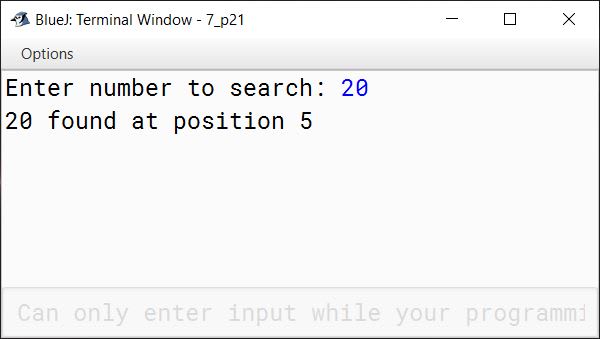
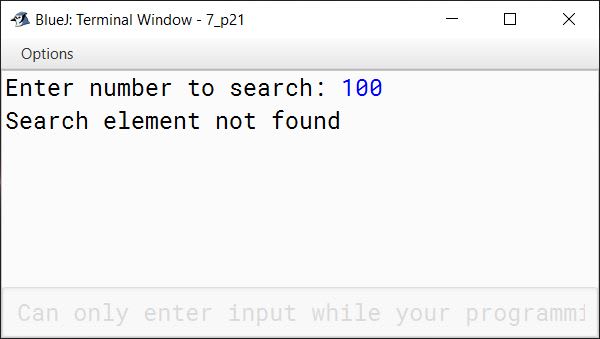
Answered By
40 Likes
Related Questions
Write a program to input 10 integer elements in an array and sort them in descending order using bubble sort technique.
Write a program that reads a long number, counts and displays the occurrences of each digit in it.
Write a program to store 6 elements in an array P and 4 elements in an array Q. Now, produce a third array R, containing all the elements of array P and Q. Display the resultant array.
Input Input Output P[ ] Q[ ] R[ ] 4 19 4 6 23 6 1 7 1 2 8 2 3 3 10 10 19 23 7 8 The annual examination result of 50 students in a class is tabulated in a Single Dimensional Array (SDA) is as follows:
Roll No. Subject A Subject B Subject C ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. Write a program to read the data, calculate and display the following:
(a) Average marks obtained by each student.
(b) Print the roll number and the average marks of the students whose average is above. 80.
(c) Print the roll number and the average marks of the students whose average is below 40.