Computer Applications
Design a class overloading a method calculate() as follows:
- void calculate(int m, char ch) with one integer argument and one character argument. It checks whether the integer argument is divisible by 7 or not, if ch is 's', otherwise, it checks whether the last digit of the integer argument is 7 or not.
- void calculate(int a, int b, char ch) with two integer arguments and one character argument. It displays the greater of integer arguments if ch is 'g' otherwise, it displays the smaller of integer arguments.
Java
User Defined Methods
ICSE
70 Likes
Answer
import java.util.Scanner;
public class KboatCalculate
{
public void calculate(int m, char ch) {
if (ch == 's') {
if (m % 7 == 0)
System.out.println("It is divisible by 7");
else
System.out.println("It is not divisible by 7");
}
else {
if (m % 10 == 7)
System.out.println("Last digit is 7");
else
System.out.println("Last digit is not 7");
}
}
public void calculate(int a, int b, char ch) {
if (ch == 'g')
System.out.println(a > b ? a : b);
else
System.out.println(a < b ? a : b);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
KboatCalculate obj = new KboatCalculate();
System.out.print("Enter a number: ");
int n1 = in.nextInt();
obj.calculate(n1, 's');
obj.calculate(n1, 't');
System.out.print("Enter first number: ");
n1 = in.nextInt();
System.out.print("Enter second number: ");
int n2 = in.nextInt();
obj.calculate(n1, n2, 'g');
obj.calculate(n1, n2, 'k');
}
}
Output
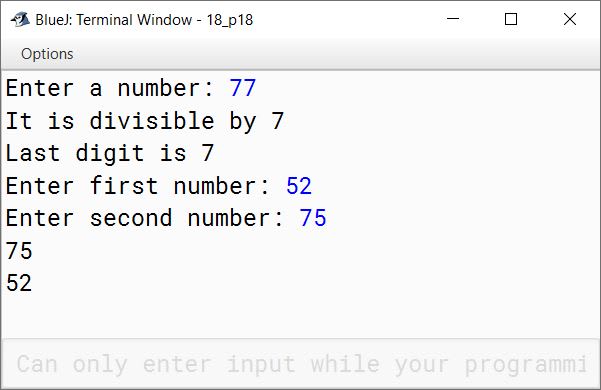
Answered By
16 Likes
Related Questions
Design a class overloading and a method display( ) as follows:
- void display(String str, int p) with one String argument and one integer argument. It displays all the uppercase characters if 'p' is 1 (one) otherwise, it displays all the lowercase characters.
- void display(String str, char chr) with one String argument and one character argument. It displays all the vowels if chr is 'v' otherwise, it displays all the alphabets.
Write a class with the name Perimeter using method overloading that computes the perimeter of a square, a rectangle and a circle.
Formula:
Perimeter of a square = 4 * s
Perimeter of a rectangle = 2 * (l + b)
Perimeter of a circle = 2 * (22/7) * r
Design a class to overload a method series( ) as follows:
- double series(double n) with one double argument and returns the sum of the series.
sum = (1/1) + (1/2) + (1/3) + ………. + (1/n) - double series(double a, double n) with two double arguments and returns the sum of the series.
sum = (1/a2) + (4/a5) + (7/a8) + (10/a11) + ………. to n terms
- double series(double n) with one double argument and returns the sum of the series.
Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.