Computer Applications
Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.
Java
User Defined Methods
ICSE
133 Likes
Answer
import java.util.Scanner;
public class KboatCompare
{
public void compare(int a, int b) {
if (a > b) {
System.out.println(a);
}
else {
System.out.println(b);
}
}
public void compare(char a, char b) {
int x = (int)a;
int y = (int)b;
if (x > y) {
System.out.println(a);
}
else {
System.out.println(b);
}
}
public void compare(String a, String b) {
int l1 = a.length();
int l2 = b.length();
if (l1 > l2) {
System.out.println(a);
}
else {
System.out.println(b);
}
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
KboatCompare obj = new KboatCompare();
System.out.print("Enter first integer: ");
int n1 = in.nextInt();
System.out.print("Enter second integer: ");
int n2 = in.nextInt();
obj.compare(n1, n2);
System.out.print("Enter first character: ");
char c1 = in.next().charAt(0);
System.out.print("Enter second character: ");
char c2 = in.next().charAt(0);
in.nextLine();
obj.compare(c1, c2);
System.out.print("Enter first string: ");
String s1 = in.nextLine();
System.out.print("Enter second string: ");
String s2 = in.nextLine();
obj.compare(s1, s2);
}
}
Output
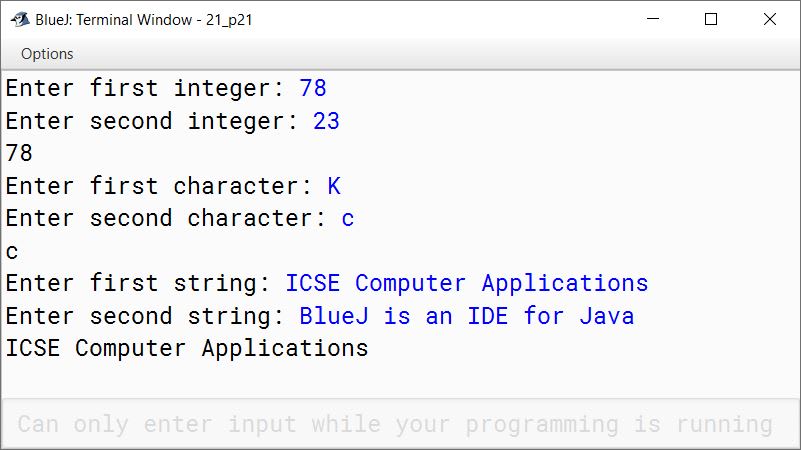
Answered By
48 Likes
Related Questions
Design a class overloading a method calculate() as follows:
- void calculate(int m, char ch) with one integer argument and one character argument. It checks whether the integer argument is divisible by 7 or not, if ch is 's', otherwise, it checks whether the last digit of the integer argument is 7 or not.
- void calculate(int a, int b, char ch) with two integer arguments and one character argument. It displays the greater of integer arguments if ch is 'g' otherwise, it displays the smaller of integer arguments.
Design a class to overload a method series( ) as follows:
- double series(double n) with one double argument and returns the sum of the series.
sum = (1/1) + (1/2) + (1/3) + ………. + (1/n) - double series(double a, double n) with two double arguments and returns the sum of the series.
sum = (1/a2) + (4/a5) + (7/a8) + (10/a11) + ………. to n terms
- double series(double n) with one double argument and returns the sum of the series.
Design a class overloading and a method display( ) as follows:
- void display(String str, int p) with one String argument and one integer argument. It displays all the uppercase characters if 'p' is 1 (one) otherwise, it displays all the lowercase characters.
- void display(String str, char chr) with one String argument and one character argument. It displays all the vowels if chr is 'v' otherwise, it displays all the alphabets.
Design a class to overload the method display(…..) as follows:
- void display(int num) — checks and prints whether the number is a perfect square or not.
- void display(String str, char ch) — checks and prints if the word str contains the letter ch or not.
- void display(String str) — checks and prints the number of special characters present in the word str.
Write a suitable main( ) function.