Computer Applications
Design a class to overload the method display(…..) as follows:
- void display(String str, char ch) — checks whether the word str contains the letter ch at the beginning as well as at the end or not. If present, print 'Special Word' otherwise print 'No special word'.
- void display(String str1, String str2) — checks and prints whether both the words are equal or not.
- void display(String str, int n) — prints the character present at nth position in the word str.
Write a suitable main() method.
Java
User Defined Methods
ICSE
32 Likes
Answer
public class KboatWordCheck
{
public void display(String str, char ch) {
String temp = str.toUpperCase();
ch = Character.toUpperCase(ch);
int len = temp.length();
if (temp.indexOf(ch) == 0 &&
temp.lastIndexOf(ch) == (len - 1))
System.out.println("Special Word");
else
System.out.println("No Special Word");
}
public void display(String str1, String str2) {
if (str1.equals(str2))
System.out.println("Equal");
else
System.out.println("Not Equal");
}
public void display(String str, int n) {
int len = str.length();
if (n < 0 || n > len) {
System.out.println("Invalid value for argument n");
return;
}
char ch = str.charAt(n - 1);
System.out.println(ch);
}
public static void main(String args[]) {
KboatWordCheck obj = new KboatWordCheck();
obj.display("Tweet", 't');
obj.display("Tweet", "Massachusetts");
obj.display("Massachusetts", 8);
}
}
Output
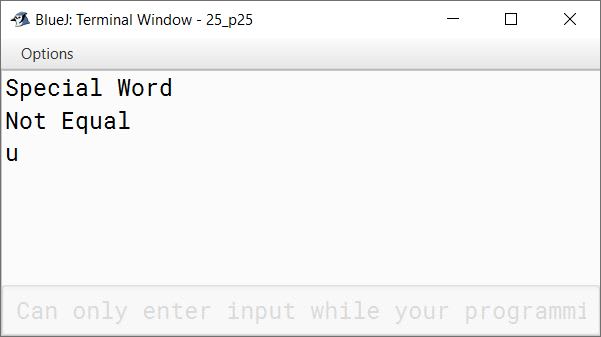
Answered By
9 Likes
Related Questions
Design a class to overload a method series( ) as follows:
- double series(double n) with one double argument and returns the sum of the series.
sum = (1/1) + (1/2) + (1/3) + ………. + (1/n) - double series(double a, double n) with two double arguments and returns the sum of the series.
sum = (1/a2) + (4/a5) + (7/a8) + (10/a11) + ………. to n terms
- double series(double n) with one double argument and returns the sum of the series.
Design a class to overload a method volume( ) as follows:
- double volume(double r) — with radius (r) as an argument, returns the volume of sphere using the formula:
V = (4/3) * (22/7) * r * r * r - double volume(double h, double r) — with height(h) and radius(r) as the arguments, returns the volume of a cylinder using the formula:
V = (22/7) * r * r * h - double volume(double 1, double b, double h) — with length(l), breadth(b) and height(h) as the arguments, returns the volume of a cuboid using the formula:
V = l*b*h ⇒ (length * breadth * height)
- double volume(double r) — with radius (r) as an argument, returns the volume of sphere using the formula:
Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.
Design a class to overload the method display(…..) as follows:
- void display(int num) — checks and prints whether the number is a perfect square or not.
- void display(String str, char ch) — checks and prints if the word str contains the letter ch or not.
- void display(String str) — checks and prints the number of special characters present in the word str.
Write a suitable main( ) function.