Computer Applications
Write a Java program in which you input students name, class, roll number, and marks in 5 subjects. Find out the total marks, percentage, and grade according to the following table.
Percentage | Grade |
---|---|
>= 90 | A+ |
>= 80 and < 90 | A |
>= 70 and < 80 | B+ |
>= 60 and < 70 | B |
>= 50 and < 60 | C |
>= 40 and < 50 | D |
< 40 | E |
Java
Java Conditional Stmts
ICSE
86 Likes
Answer
import java.util.Scanner;
public class KboatStudentGrades
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter name of student: ");
String n = in.nextLine();
System.out.print("Enter class of student: ");
int c = in.nextInt();
System.out.print("Enter roll no of student: ");
int r = in.nextInt();
System.out.print("Enter marks in 1st subject: ");
int m1 = in.nextInt();
System.out.print("Enter marks in 2nd subject: ");
int m2 = in.nextInt();
System.out.print("Enter marks in 3rd subject: ");
int m3 = in.nextInt();
System.out.print("Enter marks in 4th subject: ");
int m4 = in.nextInt();
System.out.print("Enter marks in 5th subject: ");
int m5 = in.nextInt();
int t = m1 + m2 + m3 + m4 + m5;
double p = t / 500.0 * 100.0;
String g = "";
if (p >= 90)
g = "A+";
else if (p >= 80)
g = "A";
else if (p >=70)
g = "B+";
else if (p >= 60)
g = "B";
else if (p >= 50)
g = "C";
else if (p >= 40)
g = "D";
else
g = "E";
System.out.println("Total Marks = " + t);
System.out.println("Percentage = " + p);
System.out.println("Grade = " + g);
}
}
Output
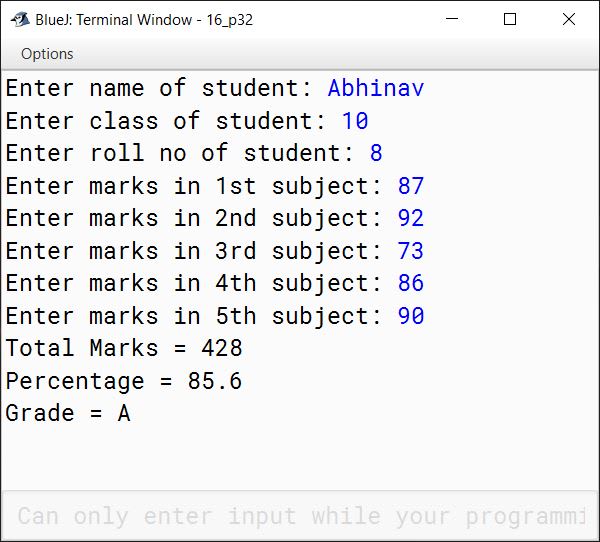
Answered By
39 Likes
Related Questions
The electricity board charges the bill according to the number of units consumed and the rate as given below:
Units Consumed Rate Per Unit First 100 units 80 Paisa per unit Next 200 units Rs. 1 per unit Above 300 units Rs. 2.50 per unit Write a program in Java to accept the total units consumed by a customer and calculate the bill. Assume that a meter rent of Rs. 500 is charged from the customer.
Write a menu driven program to accept a number from the user and check whether it is a Buzz number or an Automorphic number.
i. Automorphic number is a number, whose square's last digit(s) are equal to that number. For example, 25 is an automorphic number, as its square is 625 and 25 is present as the last two digits.
ii. Buzz number is a number, that ends with 7 or is divisible by 7.Write a program in Java to accept three numbers and check whether they are Pythagorean Triplet or not. The program must display the message accordingly. [Hint: h2=p2+b2]
Using switch case, write a program to convert temperature from Fahrenheit to Celsius and Celsius to Fahrenheit.
Fahrenheit to Celsius formula: (32°F - 32) x 5/9
Celsius to Fahrenheit formula: (0°C x 9/5) + 32