Computer Applications
Write a menu driven program to accept a number from the user and check whether it is a Buzz number or an Automorphic number.
i. Automorphic number is a number, whose square's last digit(s) are equal to that number. For example, 25 is an automorphic number, as its square is 625 and 25 is present as the last two digits.
ii. Buzz number is a number, that ends with 7 or is divisible by 7.
Java
Java Conditional Stmts
ICSE
49 Likes
Answer
import java.util.Scanner;
public class KboatBuzzAutomorphic
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Buzz number");
System.out.println("2. Automorphic number");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
System.out.print("Enter number: ");
int num = in.nextInt();
switch (choice) {
case 1:
if (num % 10 == 7 || num % 7 == 0)
System.out.println(num + " is a Buzz Number");
else
System.out.println(num + " is not a Buzz Number");
break;
case 2:
int sq = num * num;
int d = 0;
int t = num;
/*
* Count the number of
* digits in num
*/
while(t > 0) {
d++;
t /= 10;
}
/*
* Extract the last d digits
* from square of num
*/
int ld = (int)(sq % Math.pow(10, d));
if (ld == num)
System.out.println(num + " is automorphic");
else
System.out.println(num + " is not automorphic");
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Output
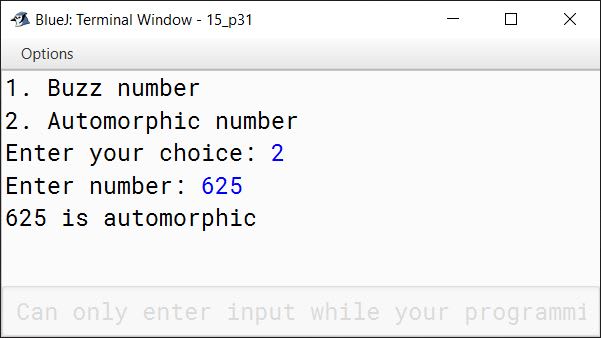
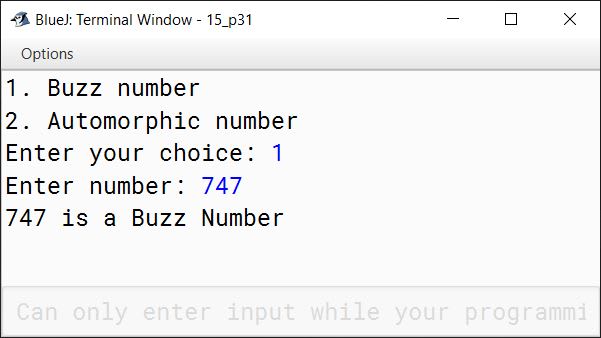
Answered By
17 Likes
Related Questions
Create a program in Java to find out if a number entered by the user is a Duck Number.
A Duck Number is a number which has zeroes present in it, but there should be no zero present in the beginning of the number. For example, 6710, 8066, 5660303 are all duck numbers whereas 05257, 080009 are not.
Write a Java program in which you input students name, class, roll number, and marks in 5 subjects. Find out the total marks, percentage, and grade according to the following table.
Percentage Grade >= 90 A+ >= 80 and < 90 A >= 70 and < 80 B+ >= 60 and < 70 B >= 50 and < 60 C >= 40 and < 50 D < 40 E Write a program in Java to accept three numbers and check whether they are Pythagorean Triplet or not. The program must display the message accordingly. [Hint: h2=p2+b2]
The electricity board charges the bill according to the number of units consumed and the rate as given below:
Units Consumed Rate Per Unit First 100 units 80 Paisa per unit Next 200 units Rs. 1 per unit Above 300 units Rs. 2.50 per unit Write a program in Java to accept the total units consumed by a customer and calculate the bill. Assume that a meter rent of Rs. 500 is charged from the customer.