Computer Applications
Write a program to input a number and check and print whether it is a 'Pronic' number or not. Use a method int Pronic(int n) to accept a number. The method returns 1, if the number is 'Pronic', otherwise returns zero (0).
(Hint: Pronic number is the number which is the product of two consecutive integers)
Examples:
12 = 3 * 4
20 = 4 * 5
42 = 6 * 7
Java
User Defined Methods
ICSE
66 Likes
Answer
import java.util.Scanner;
public class KboatPronicNumber
{
public int pronic(int n) {
int isPronic = 0;
for (int i = 1; i <= n - 1; i++) {
if (i * (i + 1) == n) {
isPronic = 1;
break;
}
}
return isPronic;
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the number to check: ");
int num = in.nextInt();
KboatPronicNumber obj = new KboatPronicNumber();
int r = obj.pronic(num);
if (r == 1)
System.out.println(num + " is a pronic number");
else
System.out.println(num + " is not a pronic number");
}
}
Output
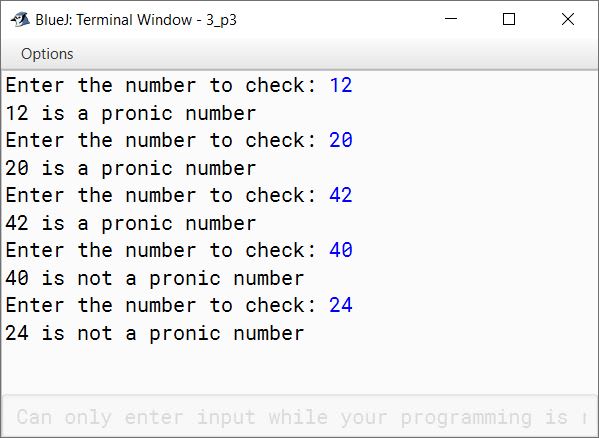
Answered By
30 Likes
Related Questions
Write a program to enter a two digit number and find out its first factor excluding 1 (one). The program then find the second factor (when the number is divided by the first factor) and finally displays both the factors.
Hint: Use a non-return type method as void fact(int n) to accept the number.Sample Input: 21
The first factor of 21 is 3
Sample Output: 3, 7
Sample Input: 30
The first factor of 30 is 2
Sample Output: 2, 15Write a program in Java using a method Discount( ), to calculate a single discount or a successive discount. Use overload methods Discount(int), Discount(int,int) and Discount(int,int,int) to calculate single discount and successive discount respectively. Calculate and display the amount to be paid by the customer after getting discounts on the printed price of an article.
Sample Input:
Printed price: ₹12000
Successive discounts = 10%, 8%
= ₹(12000 - 1200)
= ₹(10800 - 864)
Amount to be paid = ₹9936Write a program to input a three digit number. Use a method int Armstrong(int n) to accept the number. The method returns 1, if the number is Armstrong, otherwise zero(0).
Sample Input: 153
Sample Output: 153 ⇒ 13 + 53 + 33 = 153
It is an Armstrong Number.Write a method fact(int n) to find the factorial of a number n. Include a main class to find the value of S where:
S = n! / (m!(n - m)!)
where, n! = 1 x 2 x 3 x ………. x n