Computer Applications
Write a method fact(int n) to find the factorial of a number n. Include a main class to find the value of S where:
S = n! / (m!(n - m)!)
where, n! = 1 x 2 x 3 x ………. x n
Java
User Defined Methods
ICSE
53 Likes
Answer
import java.util.Scanner;
public class KboatFactorial
{
public long fact(int n) {
long f = 1;
for (int i = 1; i <= n; i++) {
f *= i;
}
return f;
}
public static void main(String args[]) {
KboatFactorial obj = new KboatFactorial();
Scanner in = new Scanner(System.in);
System.out.print("Enter m: ");
int m = in.nextInt();
System.out.print("Enter n: ");
int n = in.nextInt();
double s = (double)(obj.fact(n)) / (obj.fact(m) * obj.fact(n - m));
System.out.println("S=" + s);
}
}
Output
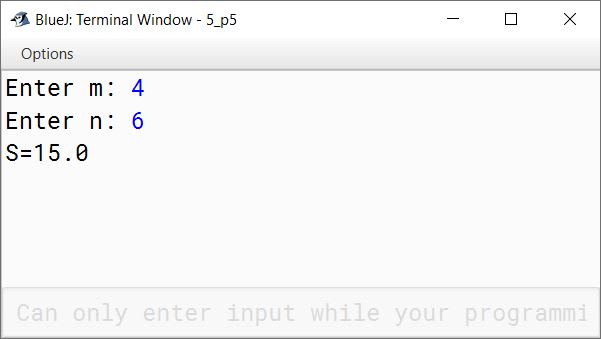
Answered By
20 Likes
Related Questions
Write a program to enter a two digit number and find out its first factor excluding 1 (one). The program then find the second factor (when the number is divided by the first factor) and finally displays both the factors.
Hint: Use a non-return type method as void fact(int n) to accept the number.Sample Input: 21
The first factor of 21 is 3
Sample Output: 3, 7
Sample Input: 30
The first factor of 30 is 2
Sample Output: 2, 15Write a program to input a number and check and print whether it is a 'Pronic' number or not. Use a method int Pronic(int n) to accept a number. The method returns 1, if the number is 'Pronic', otherwise returns zero (0).
(Hint: Pronic number is the number which is the product of two consecutive integers)Examples:
12 = 3 * 4
20 = 4 * 5
42 = 6 * 7Write a program using a method called area() to compute area of the following:
(a) Area of circle = (22/7) * r * r
(b) Area of square= side * side
(c) Area of rectangle = length * breadth
Display the menu to display the area as per the user's choice.
Write a program using method name Glcm(int,int) to find the Lowest Common Multiple (LCM) of two numbers by GCD (Greatest Common Divisor) of the numbers. GCD of two integers is calculated by continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process is repeated till the remainder is zero. The divisor then results in the GCD.
LCM = product of two numbers / GCD