In this lesson, we will see how to use the new operator to create a double dimensional array. Let’s say I want to create an int array of dimensions 3 by 2 and I want to name it arr.
First, I will declare a double dimensional array variable like this:
int arr[][];
Then I will create the array with new operator like this:
arr = new int[3][2];
I can perform both these steps with a single statement like this:
int arr[][] = new int[3][2];
This will create a 3 by 2 double dimensional array and initialize all its elements to zero.
The general syntax of creating a 2D array with new operator is this:
<type> array-variable = new type[rows][columns];
It is like single dimensional array just that in this case we must provide one more dimension.
Assigning Values to Elements of 2D Array
We can assign a value to an element of double dimensional array like this:
arr[0][0] = 1;
This statement will assign the value of one to the element of arr at index zero zero. This is like assigning values to the elements of a single dimensional array, just that in this case we need to write two subscripts.
Below statements assign values to the rest of the elements of arr:
arr[0][1] = 3; //Assigns 3 to element at index zero one
arr[1][0] = 5; //Assigns 5 to element at index one zero
arr[1][1] = 7; //Assigns 7 to element at index one one
arr[2][0] = 9; //Assigns 9 to element at index two zero
arr[2][1] = 11; //Assigns 11 to element at index two one
Accepting User Input Row-Wise
For a double dimensional array, we usually store the values provided by the user row-wise. Let's first understand the meaning of storing the values row-wise in a double dimensional array.
As you can see in the animation above, I stored the values that the user entered row-wise. I started with the first row, then I filled the second row and after that I filled the third row.
BlueJ Program to Store User Input Row-wise and Display the 2D Array in Matrix Form
import java.util.Scanner;
public class KboatDDAUserInputDemo
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
/*
* Ask the user to provide the
* dimensions of the 2D array
*/
System.out.print("Enter the number of rows: ");
int rows = in.nextInt();
System.out.print("Enter the number of columns: ");
int cols = in.nextInt();
/*
* Create the 2D array of the
* provided dimensions using
* the new operator
*/
int arr[][] = new int[rows][cols];
/*
* Ask the user to input the
* elements of the array and
* store them row-wise in arr
*/
System.out.println("Enter the elements of the 2D array:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
arr[i][j] = in.nextInt();
}
}
/*
* Print arr in matrix format
*/
System.out.println("2D Array in Matrix form:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
}
}
Output
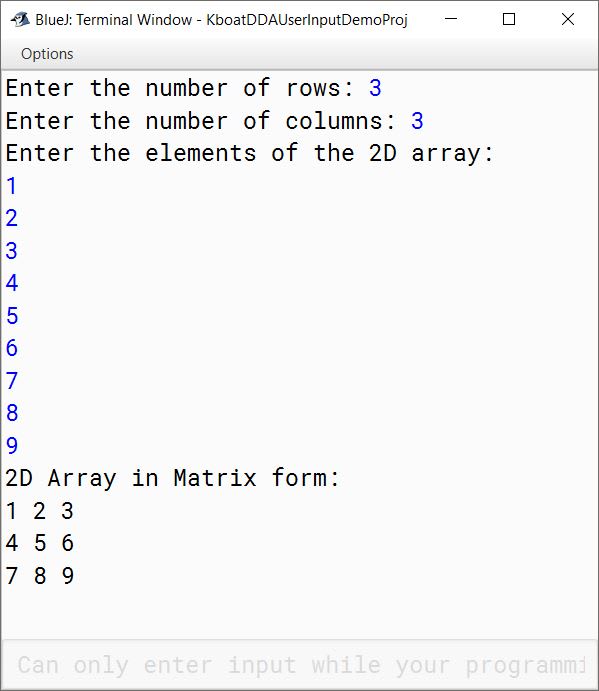
In the program above, we first ask the user to enter the dimensions of the array. After that, we create it using the new operator. Next, we ask the user to enter the elements of the array and store it row-wise. In the end, we display the array in matrix form to ensure that all the elements are correctly stored in the array.